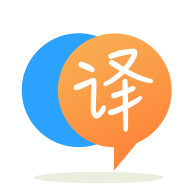
[英]Converting CSV to jSON - Keep getting "End of File expected" error
[英]Converting dynamic JSON to CSV in Golang
我试图将动态 JSON 转换为 CSV,我调查了库和答案,我找不到什么了不起的东西。
这个和这个示例可能会有所帮助,但我无法将 JSON 的结构添加到我的代码中,JSON 是动态的。
在 Python & JS 中,我看到了这些例子;
Python;
# Python program to convert
# JSON file to CSV
import json
import csv
# Opening JSON file and loading the data
# into the variable data
with open('data.json') as json_file:
data = json.load(json_file)
employee_data = data['emp_details']
# now we will open a file for writing
data_file = open('data_file.csv', 'w')
# create the csv writer object
csv_writer = csv.writer(data_file)
# Counter variable used for writing
# headers to the CSV file
count = 0
for emp in employee_data:
if count == 0:
# Writing headers of CSV file
header = emp.keys()
csv_writer.writerow(header)
count += 1
# Writing data of CSV file
csv_writer.writerow(emp.values())
data_file.close()
JS;
const items = json3.items
const replacer = (key, value) => value === null ? '' : value // specify how you want to handle null values here
const header = Object.keys(items[0])
const csv = [
header.join(','), // header row first
...items.map(row => header.map(fieldName => JSON.stringify(row[fieldName], replacer)).join(','))
].join('\r\n')
console.log(csv)
这些代码有助于轻松地将动态 JSON 转换为 CSV。
示例输入 & output;
JSON(输入);
[
{
"name": "John",
"age": "21"
},
{
"name": "Noah",
"age": "23"
},
{
"name": "Justin",
"age": "25"
}
]
CSV(输出);
"name","age"
"John","21"
"Noah","23"
"Justi","25"
那么如何在 Go 中将动态 JSON 转换为 CSV ?
PS:我发现了一个 Golang 库( json2csv ),它有助于转换,但只能在命令提示符下工作。
例如,我很少使用在线工具;
将此粘贴到https://github.com/yukithm/json2csv/blob/master/cmd/json2csv/main.go
并将函数插入到 main。
func stackoverflow() {
jsonStr := `
[
{
"name": "John",
"age": "21"
},
{
"name": "Noah",
"age": "23"
},
{
"name": "Justin",
"age": "25"
}
]`
buff := bytes.NewBufferString(jsonStr)
data, _ := readJSON(buff)
results, _ := json2csv.JSON2CSV(data)
headerStyle := headerStyleTable["jsonpointer"]
err := printCSV(os.Stdout, results, headerStyle, false)
if err != nil {
log.Fatal(err)
}
}
这个对我有用。
➜ json2csv git:(master) ✗ go run main.go
/age,/name
21,John
23,Noah
25,Justin
我想我用yukithm/json2csv
package 处理它。
package main
import (
"bytes"
"encoding/json"
"github.com/yukithm/json2csv"
"log"
"os"
)
func main() {
b := &bytes.Buffer{}
wr := json2csv.NewCSVWriter(b)
j, _ := os.ReadFile("your-input-path\\input.json")
var x []map[string]interface{}
// unMarshall json
err := json.Unmarshal(j, &x)
if err != nil {
log.Fatal(err)
}
// convert json to CSV
csv, err := json2csv.JSON2CSV(x)
if err != nil {
log.Fatal(err)
}
// CSV bytes convert & writing...
err = wr.WriteCSV(csv)
if err != nil {
log.Fatal(err)
}
wr.Flush()
got := b.String()
//Following line prints CSV
println(got)
// create file and append if you want
createFileAppendText("output.csv", got)
}
//
func createFileAppendText(filename string, text string) {
f, err := os.OpenFile(filename, os.O_APPEND|os.O_WRONLY|os.O_CREATE, 0600)
if err != nil {
panic(err)
}
defer f.Close()
if _, err = f.WriteString(text); err != nil {
panic(err)
}
}
输入。json;
[
{
"Name": "Japan",
"Capital": "Tokyo",
"Continent": "Asia"
},
{
"Name": "Germany",
"Capital": "Berlin",
"Continent": "Europe"
},
{
"Name": "Turkey",
"Capital": "Ankara",
"Continent": "Europe"
},
{
"Name": "Greece",
"Capital": "Athens",
"Continent": "Europe"
},
{
"Name": "Israel",
"Capital": "Jerusalem",
"Continent": "Asia"
}
]
output.csv
/Capital,/Continent,/Name
Tokyo,Asia,Japan
Berlin,Europe,Germany
Ankara,Europe,Turkey
Athens,Europe,Greece
Jerusalem,Asia,Israel
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.