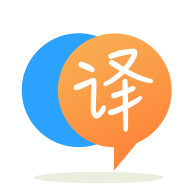
[英]Python w/ Stripe: How do I get valid JSON from a charge response?
[英]How do i properly get struct items from stripe subscription response object?
我正在尝试从条带响应对象的结构中获取一些数据以进行订阅。 这里是响应结构的链接 object stripe 订阅 object
这是我所拥有的以及正在尝试做的事情
type SubscriptionDetails struct {
CustomerId string `json:"customer_id"`
SubscritpionId string `json:"subscritpion_id"`
StartAt time.Time `json:"start_at"`
EndAt time.Time `json:"end_at"`
Interval string `json:"interval"`
Plan string `json:"plan"`
PlanId string `json:"plan_id"`
SeatCount uint8 `json:"seat_count"`
PricePerSeat float64 `json:"price_per_seat"`
}
func CreateStripeSubscription(CustomerId string, planId string) (*SubscriptionDetails, error) {
stripe.Key = StripeKey
params := &stripe.SubscriptionParams{
Customer: stripe.String(CustomerId),
Items: []*stripe.SubscriptionItemsParams{
&stripe.SubscriptionItemsParams{
Price: stripe.String(planId),
},
},
}
result, err := sub.New(params)
if err != nil {
return nil, err
}
data := &SubscriptionDetails{}
data.CustomerId = result.Customer
data.SubscritpionId = result.ID
data.StartAt = result.CurrentPeriodStart
data.EndAt = result.CurrentPeriodEnd
data.Interval = result.Items.Data.Price.Recurring.Interval
data.Plan = result.Items.Data.Price.Nickname
data.SeatCount = result.Items.Data.Quantity
data.PricePerSeat = result.Items.Data.Price.UnitAmount
return data, nil
}
有些项目很容易直接得到,比如我很容易用result.ID
得到的ID
字段,没有投诉,但对于其他项目,这里是错误消息
cannot use result.CurrentPeriodStart (type int64) as type time.Time in assignment
...
cannot use result.Customer (type *stripe.Customer) as type string in assignment
...
result.Items.Data.price undefined (type []*stripe.SubscriptionItem has no field or method price)
那么如何获取data.CustomerId
和data.PricePerSeat
的数据呢?
更新:
这是来自条带的订阅 object 的结构
type FilesStripeCreateSubscription struct {
ID string `json:"id"`
CancelAt interface{} `json:"cancel_at"`
CancelAtPeriodEnd bool `json:"cancel_at_period_end"`
CanceledAt interface{} `json:"canceled_at"`
CurrentPeriodEnd int64 `json:"current_period_end"`
CurrentPeriodStart int64 `json:"current_period_start"`
Customer string `json:"customer"`
Items struct {
Data []struct {
ID string `json:"id"`
BillingThresholds interface{} `json:"billing_thresholds"`
Created int64 `json:"created"`
Metadata struct {
} `json:"metadata"`
Object string `json:"object"`
Price struct {
ID string `json:"id"`
Active bool `json:"active"`
Currency string `json:"currency"`
CustomUnitAmount interface{} `json:"custom_unit_amount"`
Metadata struct {
} `json:"metadata"`
Nickname string `json:"nickname"`
Object string `json:"object"`
Product string `json:"product"`
Recurring struct {
AggregateUsage interface{} `json:"aggregate_usage"`
Interval string `json:"interval"`
IntervalCount int64 `json:"interval_count"`
UsageType string `json:"usage_type"`
} `json:"recurring"`
TaxBehavior string `json:"tax_behavior"`
TiersMode interface{} `json:"tiers_mode"`
TransformQuantity interface{} `json:"transform_quantity"`
Type string `json:"type"`
UnitAmount int64 `json:"unit_amount"`
UnitAmountDecimal int64 `json:"unit_amount_decimal,string"`
} `json:"price"`
Quantity int64 `json:"quantity"`
Subscription string `json:"subscription"`
TaxRates []interface{} `json:"tax_rates"`
} `json:"data"`
} `json:"items"`
}
查看您提到的 3 个错误
cannot use result.CurrentPeriodStart (type int64) as type time.Time in assignment
result.CurrentPeriodStart
的类型是 int64 并且您试图将其设置为time.Time
类型的字段,这显然会失败。 API 以 unix 格式发送时间,您需要将其解析为 time.Time。 也对其他时间字段执行此操作
data.StartAt = time.Unix(result.CurrentPeriodStart, 0)
cannot use result.Customer (type *stripe.Customer) as type string in assignment
与上面类似的问题,字段result.Customer
是*stripe.Customer
类型,而您尝试将其设置为string
类型的字段。 客户 ID 是结构Customer
中的一个字段
data.CustomerId = result.Customer.ID
result.Items.Data.price undefined (type []*stripe.SubscriptionItem has no field or method price)
stripe.SubscriptionItem
结构没有price
字段。 我不确定你想要什么。 我建议阅读订阅 object 文档。
让我们首先 go 了解在后台运行的代码,返回的内容,然后我们一次看一个问题。
当我们使用params
调用sub.New()
方法时,它返回Subscription
类型,可以在here
看到
注意:我只会显示有限的类型定义,因为添加完整的结构会使答案变得很大,而不是特定于问题上下文
让我们只看Subscription
类型
type Subscription struct {
...
// Start of the current period that the subscription has been invoiced for.
CurrentPeriodStart int64 `json:"current_period_start"`
// ID of the customer who owns the subscription.
Customer *Customer `json:"customer"`
...
// List of subscription items, each with an attached price.
Items *SubscriptionItemList `json:"items"`
...
}
让我们看看第一个错误
cannot use result.CurrentPeriodStart (type int64) as type time.Time in assignment
根据Subscription
类型,我们可以看到CurrentPeriodStart
的类型为int64
,而您尝试将其设置为SubscriptionDetails
类型的StartAt
字段,该字段的类型为time.Time
,因为类型不同,因此无法分配以解决我们需要明确的问题将其转换为time.Time
可以按如下方式完成:
data.StartAt = time.Unix(result.CurrentPeriodStart, 0)
然后您可以将time.Unix
方法的返回值分配给StartAt
字段
现在让我们继续第二个错误
cannot use result.Customer (type *stripe.Customer) as type string in assignment
正如我们从Subscription
定义中看到的那样, Customer
字段的类型是*Customer
,它不是字符串类型,因为您试图将*Customer
类型分配给字符串类型的CustomerId
字段,这不可能导致上述错误,因为我们指的是不正确的数据正确的数据在哪里 正确的数据在*Customer
type withing ID
字段中可用,可以按如下方式检索
data.CustomerId = result.Customer.ID
让 go 超过最后一个错误
result.Items.Data.price undefined (type []*stripe.SubscriptionItem has no field or method price)
同样,如果我们查看Subscription
定义,我们可以看到Items
字段的类型为*SubscriptionItemList
类型,我们查看*SubscriptionItemList
定义
type SubscriptionItemList struct {
APIResource
ListMeta
Data []*SubscriptionItem `json:"data"`
}
它包含一个字段名称Data
和 Data 的类型是[]*SubscriptionItem
注意它是*SubscriptionItem
SubscriptionItem 的 slice []
,现在让我们看看*SubscriptionItem
定义
type SubscriptionItem struct {
...
Price *Price `json:"price"`
...
}
它包含Price
字段注意名称以大写字母开头,最后如果我们查看Price
定义
type Price struct {
...
// The unit amount in %s to be charged, represented as a whole integer if possible. Only set if `billing_scheme=per_unit`.
UnitAmount int64 `json:"unit_amount"`
// The unit amount in %s to be charged, represented as a decimal string with at most 12 decimal places. Only set if `billing_scheme=per_unit`.
UnitAmountDecimal float64 `json:"unit_amount_decimal,string"`
...
}
它包含我们需要的UnitAmount
字段,但是这里有一个问题UnitAmount
是int64
类型,但PricePerSeat
是float64
类型,所以您可能需要根据要求使用float64
类型的UnitAmountDecimal
,因此按照说明我们可以解决问题如下
data.PricePerSeat = result.Items.Data[0].Price.UnitAmountDecimal
访问价格result.Items.Data[0].Price.UnitAmount
如果您使用调试器,只需在sub.New
行之后放置一个断点并探索结果变量的内容
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.