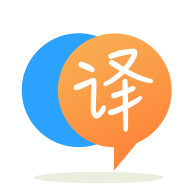
[英]breaking an outer Python for loop based on condition in inner for loop
[英]Python: Breaking out of inner loop to a specific condition in the outer while loop
该程序运行良好,但如果我可以将内部循环中断到外部 while 循环中的特定条件会更好。 基本上,我不想再次运行整个循环,因为再次显示主菜单是多余的。 如果答案是“否”,则应该只是 go 到“查看并退出”选项。 如何从第 52 行(内循环)到第 84 行(外循环条件)中的 go?
def menu():
print('Main Menu')
print('1) Chair')
print('2) Table')
print('3) Review and Exit')
def menu2():
print('')
print('1) Yes')
print('2) No')
item1_count = 0
item2_count = 0
chair_count = 0
table_count = 0
loop = 1
while loop == 1:
menu()
while True:
try:
q = int(input('Choose an item: '))
except ValueError:
print('Choose 1-3.')
continue
else:
break
if q == 1:
item1_count = item1_count + q
while True:
try:
q2 = int(input('How many chairs? '))
except ValueError:
print('Type a number.')
continue
else:
break
chair_count = chair_count + q2
menu2()
while True:
try:
q3 = int(input('Would you like anything else? '))
except ValueError:
print('Choose 1 or 2.')
continue
if q3 == 1:
print('yes')
break
elif q3 == 2:
print('no.')
# i want to go to line 83 here if i choose no.. how?
# 'break' takes me back to the main loop (line 19)
break
elif q == 2:
item2_count = item2_count + q
while True:
try:
q4 = int(input('How many tables? '))
except ValueError:
print('Type a number.')
continue
else:
break
table_count = table_count + q4
menu2()
while True:
try:
q3 = int(input('Would you like anything else? '))
except ValueError:
print('Choose 1 or 2.')
continue
if q3 == 1:
print('yes')
break
elif q3 == 2:
print('no.')
# i want to go to line 83 here if i choose no.. how?
# 'break' takes me back to the main loop (line 19)
break
elif q == 3:
print('You ordered', chair_count, 'chair(s) and', table_count, 'table(s).')
break
else:
print('Choose 1-3.')
continue
print(item1_count)
print(round(item2_count / 2))
print(chair_count)
print(table_count)
第 19 行:在开头初始化 q3
第 23 行:添加了 if 语句
第 32 行:添加了 elif 语句
def menu():
print('Main Menu')
print('1) Chair')
print('2) Table')
print('3) Review and Exit')
def menu2():
print('')
print('1) Yes')
print('2) No')
item1_count = 0
item2_count = 0
chair_count = 0
table_count = 0
loop = 1
q3 = 0 # initialize q3 from the start because it can cause NameError on line 23 otherwise
while loop == 1:
while True:
if q3 != 2: # create this condition, so if the user chose number 2 in the last question the code below will not execute
menu()
try:
q = int(input('Choose an item: '))
except ValueError:
print('Choose 1-3.')
continue
else:
break
elif q3 == 2: # and if the user chose 2 in the last question, we will set var q to 3 and break from the loop
q = 3
break
if q == 1:
item1_count = item1_count + q
while True:
try:
q2 = int(input('How many chairs? '))
except ValueError:
print('Type a number.')
continue
else:
break
chair_count = chair_count + q2
menu2()
while True:
try:
q3 = int(input('Would you like anything else? '))
except ValueError:
print('Choose 1 or 2.')
continue
if q3 == 1:
print('yes')
break
elif q3 == 2:
print('no.')
# i want to go to line 83 here if i choose no.. how?
# 'break' takes me back to the main loop (line 19)
break
elif q == 2:
item2_count = item2_count + q
while True:
try:
q4 = int(input('How many tables? '))
except ValueError:
print('Type a number.')
continue
else:
break
table_count = table_count + q4
menu2()
while True:
try:
q3 = int(input('Would you like anything else? '))
except ValueError:
print('Choose 1 or 2.')
continue
if q3 == 1:
print('yes')
break
elif q3 == 2:
print('no.')
# i want to go to line 83 here if i choose no.. how?
# 'break' takes me back to the main loop (line 19)
break
elif q == 3:
print('You ordered', chair_count, 'chair(s) and', table_count, 'table(s).')
break
else:
print('Choose 1-3.')
continue
print(item1_count)
print(round(item2_count / 2))
print(chair_count)
print(table_count)
老实说,这个程序很糟糕,它不可读并且很难修复错误。 我建议你尝试将这个程序拆分成函数,这样代码会更易读,更专业。
你可以阅读这篇文章有一个更好的理解。
我不确定是否要根据外循环条件打破内循环,除非我们在内循环的每次迭代中检查条件。
但我希望下面的修改会产生你想要的结果:
def menu():
print('Main Menu')
print('1) Chair')
print('2) Table')
print('3) Review and Exit')
def menu2():
print('')
print('1) Yes')
print('2) No')
item1_count = 0
item2_count = 0
chair_count = 0
table_count = 0
loop = 1
stopped=False
while loop == 1:
while stopped==False:
menu()
try:
q = int(input('Choose an item: '))
except ValueError:
print('Choose 1-3.')
continue
else:
break
if q == 1:
item1_count = item1_count + q
while True:
try:
q2 = int(input('How many chairs? '))
except ValueError:
print('Type a number.')
continue
else:
break
chair_count = chair_count + q2
menu2()
while True:
try:
q3 = int(input('Would you like anything else? '))
except ValueError:
print('Choose 1 or 2.')
continue
if q3 == 1:
print('yes')
break
elif q3 == 2:
print('no.')
stopped=True
q=3
break
elif q == 2:
item2_count = item2_count + q
while True:
try:
q4 = int(input('How many tables? '))
except ValueError:
print('Type a number.')
continue
else:
break
table_count = table_count + q4
menu2()
while True:
try:
q3 = int(input('Would you like anything else? '))
except ValueError:
print('Choose 1 or 2.')
continue
if q3 == 1:
print('yes')
break
elif q3 == 2:
print('no.')
q=3
stopped=True
break
elif q == 3:
print('You ordered', chair_count, 'chair(s) and', table_count, 'table(s).')
break
else:
print('Choose 1-3.')
continue
print(item1_count)
print(round(item2_count / 2))
print(chair_count)
print(table_count)
在这里,我们在全局级别定义了一个名为stopped的变量,它告诉用户想要停止运行循环,并且当用户想要退出时我们将其设置为True 。 如果是真的,我们将停止征求意见。 此外,我们将值 3 分配给变量q以将 if 语句重定向到打印评论。 请注意,我们将调用menu() function 转移到了 while 循环内部。
and statements.但是尝试用更少的和语句来设计流程。 可以针对上述情况进行。
我希望这有帮助。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.