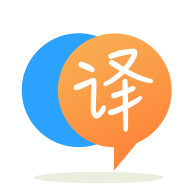
[英]Prime numbers between given two numbers in Julia and Python comparison
[英]Write a python script to print all Prime numbers between two given numbers (both values inclusive)
编写一个 python 脚本来打印两个给定数字(包括两个值)之间的所有素数,谁能告诉我在这里做错了什么?
a = int(input("Enter the value of a : "))
b = int(input("Enter the value of b : "))
for k in range(a,b):
for i in range(2,k):
if k%i!=0:
if k!=i:
continue
elif k==i:
print(k)
break
elif k!=i:
break
您正在检查一个数字是否以错误的方式出现在您的代码中有许多未处理的情况,例如,如果 a 大于 b,您无论如何都会从“a”开始循环,即使这些值正确设置,算法也不正确这是我的最佳解决方案希望它会有所帮助
a = int(input("Enter the value of a : "))
b = int(input("Enter the value of b : "))
def is_prime(n):
# negative numbers cannot be primes 1 and 0 are also not primes
if n <= 1:
return False
# since 2 is the first prime we will start looping from it
# until n since you mentioned that n is included
for i in range(2, n + 1):
# if n is cleanly divisible by any number less than n
# that means that n is not prime
if n % i == 0 and n != i:
return False
return True
for k in range(a,b):
if a > b:
print ("a cannot be bigger than b")
if is_prime(k):
print(k)
这是解决方案。 我添加了一些注释来解释代码的作用。
a = int(input("Enter the value of a : "))
b = int(input("Enter the value of b : "))
# Include b in the range by adding 1
for num in range(a, b + 1):
# Prime numbers are greater than 1
if num > 1:
for i in range(2, num):
# If the number is divisible, it is not prime
if num % i == 0:
# Check if the number is equal to the number itself
if num != i:
break
else:
# If the loop was not broken, the number isn't divisible
# by other numbers except itself and 1, so it's prime
print(num)
好吧,素数是“一个只能被它自己和 1 整除的数字”,所以实际上首先我会 go 只到range(2, k-1)
。 您拥有的这种方法是最直接的方法之一,并且在计算上并不友好。 有专门研究这种素数查找的算法。
我已经修复了代码,简化了表达式并添加了已经提到的 +1 以实现包容性。
a = int(input("Enter the value of a : "))
b = int(input("Enter the value of b : "))
for k in range(a,b+1):
for i in range(2,k+1):
if k==i:
print(k)
elif k%i!=0:
continue
else: # is divisible and isn't 1 or the number
break
我鼓励你看看这篇文章,他们是如何做到的。
另一种方法是:
#Check only 1 number at time:
def check_prime(check):
if check > 1:
for i in range(2, check):
if check % i == 0:
return False
return True
return False
#then check your numbers in a loop
list_prime = []
for i in range(50, 250):
if check_prime(i):
list_prime.append(i)
print(list_prime)
这样您就可以一次检查 1 个可能的素数。 如果您需要介于两者之间的数字,请将其放入循环中。
通常,当使用某种形式的“Erotosthenes”算法生成素数时,只需要检查分母值直到被评估数的平方根。 考虑到这一点,这里还有另一种可能的素数循环测试。
import math
a = int(input("Enter the value of a : "))
b = int(input("Enter the value of b : "))
for k in range(a,b):
if k == 2 or k == 3: # Pick up the prime numbers whose square root value is less than 2
print(k)
x = int(math.sqrt(k) + 1) # Need only check up to the square root of your test number
for i in range(2,x):
if k%i!=0:
if x-1 > i:
continue
else:
print(k)
else: # If the remainder is zero, this is not a prime number
break
另一个版本试试。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.