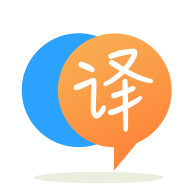
[英]How can I use Firebase Parameterized configuration with Typescript for Cloud Functions?
[英]How can I retrieve and use firebase data in a Google Cloud Function written in typescript?
我有一份关于 Firebase 的文档,其存储结构如下所示。
从 typescript 中编写的谷歌云Function中,我想访问和使用存储在我的 Firebase 项目中的 JSON 数据。 我不确定要使用的正确语法。
Firebase 文档结构:
"users": {
"tim": {
"score": 1200
"health": 200
"items": {
123123,
182281,
123344,
}
}
"james": {
"score": 100
"health": 50
"items": {
143553,
567454,
}
}
}
我目前使用以下代码从 Firebase 检索数据。
import * as admin from "firebase-admin";
export class ScoreHandler {
constructor(private firestore: FirebaseFirestore.Firestore) {}
async calculateFinalScore(name: string): Promise<number> {
try{
const document = await this.firestore
.collection("users")
.doc("users")
.get();
// Check if record exists
if (document.data() === undefined) {
throw console.warn("unable to find users document data);
}
//!!THIS IS WRONG!! not sure how to read in and access this data?
const score: number = document.data()["users"]["tim"]["score"];
const health: number = document.data()["users"]["tim"]["health"];
const items: Array<number> = document.data()["users"]["tim"]["items"];
//Calculate Final score using score, health and items
const finalScore: number = score * health * items.length;
return finalScore;
} catch (e) {
// Log the Error on the Console
console.error(e);
return 0.0;
}
}
我不确定从 Typescript 中的文档快照访问数据的正确语法。我希望能够从我的 firebase 项目中获取分数、健康状况和项目数组到我的云 function class 中。
任何帮助是极大的赞赏。
谢谢!
我试图获取数据,但一直收到 object is possibly undefined errors 并且无法实际获取数据。
要访问数据,您可以在快照 object 上使用data()
方法。此方法将文档中的数据返回为 object。
您正在使用data()
方法获取文档快照中的数据,但您没有使用正确的属性名称来访问数据。 data()
方法返回一个 object,而不是 JSON object。因此,您需要为 object 使用正确的属性名称。
尝试像这样访问 tim object 的 score 属性:
const score: number = document.data()["users"]["tim"]["score"];
访问 data() 方法返回的 JavaScript object 中的得分属性的正确方法如下所示:
const score: number = document.data()["tim"]["score"];
要访问时间 object 中的其他属性,您可以使用以下语法:
const health: number = document.data()["tim"]["health"];
const items: Array<number> = document.data()["tim"]["items"];
以下是您的calculateFinalScore()
方法应该如何使用正确的语法来访问 Firestore 文档快照中的数据:
async calculateFinalScore(name: string): Promise<number> {
try{
const document = await this.firestore
.collection("users")
.doc("users")
.get();
// Check if record exists
if (document.data() === undefined) {
throw console.warn("unable to find users document data");
}
// Get the score, health and items from the document snapshot
const score: number = document.data()["tim"]["score"];
const health: number = document.data()["tim"]["health"];
const items: Array<number> = document.data()["tim"]["items"];
// Calculate Final score using score, health and items
const finalScore: number = score * health * items.length;
return finalScore;
} catch (e) {
// Log the Error on the Console
console.error(e);
return 0.0;
}
}
用户名被硬编码为“tim”。 如果要访问不同用户的数据,则需要将“tim”属性名称替换为要访问的用户的名称。 像:
const score: number = document.data()["james"]["score"];
const health: number = document.data()["james"]["health"];
const items: Array<number> = document.data()["james"]["items"];
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.