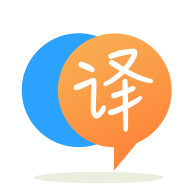
[英]Python - Can not download file from Google Drive using Google Drive API
[英]Download file from Google Drive using Python 3.10
使用 Drive API 下载文件。
应该可以使用官方文档中 Python 快速入门中的示例来下载文件。 我有一个基于它的代码,允许我下载图像:
from __future__ import print_function
import os.path
import requests
import io
from google.auth.transport.requests import Request
from google.oauth2.credentials import Credentials
from google_auth_oauthlib.flow import InstalledAppFlow
from googleapiclient.discovery import build
from googleapiclient.errors import HttpError
from googleapiclient.http import MediaFileUpload
from googleapiclient.http import MediaIoBaseDownload
# If modifying these scopes, delete the file token.json.
SCOPES = ['https://www.googleapis.com/auth/drive']
def creds():
creds = None
if os.path.exists('token.json'):
creds = Credentials.from_authorized_user_file('token.json', SCOPES)
if not creds or not creds.valid:
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
else:
flow = InstalledAppFlow.from_client_secrets_file(
'credentials.json', SCOPES)
creds = flow.run_local_server(port=0)
with open('token.json', 'w') as token:
token.write(creds.to_json())
return creds
def download(creds, real_file_id):
try:
# create drive api client
service = build('drive', 'v3', credentials=creds)
file_id = real_file_id
# pylint: disable=maybe-no-member
request = service.files().get_media(fileId=file_id)
file = io.BytesIO()
downloader = MediaIoBaseDownload(file, request)
done = False
while done is False:
status, done = downloader.next_chunk()
print(F'Download {int(status.progress() * 100)}.')
except HttpError as error:
print(F'An error occurred: {error}')
return file.getvalue()
if __name__ == '__main__':
file = open("test.jpg","wb")
file.write(download(creds(), "ID of the Image"))
您只需要在示例代码的末尾有您需要下载并粘贴以进行测试的文件的 ID。
确保已创建凭据并按照快速启动过程在 Python 下使用 Google 云端硬盘。
参考:
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.