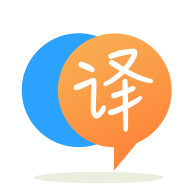
[英]Time Complexity while deleting last element from arraylist and linkedlist
[英]Problem with deleting the last element of a LinkedList
我正在尝试在LinkedList
中实现delete()
方法,这是链表数据结构的自定义实现。
如果您删除第一个元素或中间的一个元素,以下代码似乎可以正常工作。 但是如果我试图删除LinkedList
中的最后一个元素,它会抛出NullPointerException
。
我该如何解决这个问题?
我的代码:
public class LinkedList implements List {
Node first;
Node last;
int size;
public LinkedList() {
this.first = null;
this.last = null;
this.size = 0;
}
public void add(double element) {
Node newNode = new Node(element);
if (first == null) {
first = newNode;
last = newNode;
} else {
last.next = newNode;
last = newNode;
}
size++;
}
// more methods (unrelated to the problem)
public void delete(double element) {
if (first == null) {
return;
}
if (first.value == element) {
first = first.next;
return;
}
Node current = first;
Node previous = null;
while (current != null && current.next.value != element) {
previous = current;
current = current.next;
}
if (current == null) {
return;
} else {
current.next = current.next.next;
}
}
@Override
public String toString() {
if (first == null) {
return "[]";
} else {
StringBuffer sb = new StringBuffer();
sb.append("[");
for (Node current = first; current != last; current = current.next) {
sb.append(current.value);
sb.append(",");
}
sb.append(last.value);
sb.append("]");
return sb.toString();
}
}
}
class LinkedListTest {
public static void main(String[] args) {
LinkedList list = new LinkedList();
list.add(5);
list.add(25);
list.add(-7);
list.add(80);
System.out.println(list);
list.delete(80);
System.out.println(list);
}
}
delete()
方法的实现有两个问题:
while
循环中的条件不正确。 相反,检查current != null
是否需要确保current.next
不为null
(请注意,访问current
的next
属性是安全的,因为迭代从将current
分配给first
开始,这被证明是非 null )。
当要删除的节点是最后一个节点时,您没有考虑边缘情况。 在这种情况下,当控制从while
循环中跳出时, current.next
将指向last
节点,这意味着应该重新分配last
字段(因为我们要删除最后一个节点)。
此外,局部变量previous
是多余的。
public void delete(double element) {
if (first == null) {
return;
}
if (first.value == element) {
first = first.next;
return;
}
Node current = first;
while (current.next != null && current.next.value != element) {
current = current.next;
}
if (current.next == last) { // reassign last if the Node to be removed is the current last Node
last = current;
}
if (current.next != null) { // if the Node with given value was found
current.next = current.next.next;
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.