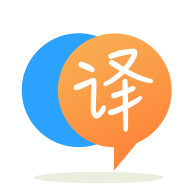
[英]How to create the insert function for a binary search tree built with a vector?
[英]how do you create an Insert function for Binary search tree using nodes as parameters?
我刚刚开始我的第二个 C++ class 并且正在努力掌握节点和链表的概念。 我为二叉树找到的每个插入 function 都使用 int 作为参数,或者使用 int 节点。 例子:
Node* InsertNode(Node* root, int data)
{
// If the tree is empty, assign new node address to root
if (root == NULL) {
root = CreateNode(data);
return root;
}
// Else, do level order traversal until we find an empty
// place, i.e. either left child or right child of some
// node is pointing to NULL.
queue<Node*> q;
q.push(root);
while (!q.empty()) {
Node* temp = q.front();
q.pop();
if (temp->left != NULL)
q.push(temp->left);
else {
temp->left = CreateNode(data);
return root;
}
if (temp->right != NULL)
q.push(temp->right);
else {
temp->right = CreateNode(data);
return root;
}
}
但是这个练习题需要两个节点作为参数。
function (Insert(Node *insert_node, Node *tree_root){
}
这是完整的代码:
#include <iostream>
#include <cstddef>
using std::cout;
using std::endl;
class Node {
int value;
public:
Node* left; // left child
Node* right; // right child
Node* p; // parent
Node(int data) {
value = data;
left = NULL;
right = NULL;
p = NULL;
}
~Node() {
}
int d() {
return value;
}
void print() {
std::cout << value << std::endl;
}
};
int main(int argc, const char * argv[])
{
}
function insert(Node *insert_node, Node *tree_root){
//Your code here
}
function delete_node(int value, Node *tree_root){
//Your code here
}
function search(int value, Node *tree_root){
//Your code here
}
我不知道为什么我如此纠结于这些概念。 谁能指出正确的方向?
你有一个Node
对象,它有一个名为data
的成员,它是一个int
。
您还没有提供完整的插入内容(带有数字参数),所以我只会告诉您如何重构它:
int data = insert_node->data;
,所以稍后data
将具有正确的值temp->left = CreateNode(data);
或temp->right = CreateNode(data);
,你将需要temp->left = insert_node;
和temp->right = insert_node;
,分别,因为你已经有一个节点可以使用,不需要创建一个tree_root
,之前它被称为root
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.