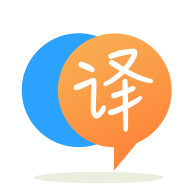
[英]How do I iterate over two lists of dictionaries to find a common key-value pair?
[英]How do I check if any key-value pair appears more than once in a list of dictionaries?
例如:
people_list = [{"name":"joe", "age":20}, {"name":"tom", "age":35}, {"name":"joe", "age":46}]
如何检查任何键值对是否在此字典列表中出现多次?
我知道柜台 function。
from collections import Counter
for i in range(len(people_list):
Counter(people_list[i]["name"])
for key,value in Counter:
if Counter(key:value) > 1:
...
只是不确定如何让它在这个字典列表中查找和计算特定的键值对,并检查它是否出现不止一次。
您想要获取每个字典中的所有键/值对,并将它们添加到一个Counter
实例中,该实例的键为 (key, value),其值最终将成为该对存在的次数:
from itertools import chain
from collections import Counter
l = [
{"name":"joe", "age":20},
{"name":"tom", "age":35},
{"name":"joe", "age":46}
]
c = Counter(chain.from_iterable(d.items() for d in l))
# Now build the new list of tuples (key-name, key-value, count-of-occurrences)
# but include only those key/value pairs that occur more than once:
l = [(k[0], k[1], v) for k, v in c.items() if v > 1]
print(l)
印刷:
[('name', 'joe', 2)]
如果要创建一个新的字典列表,其中“name”键值是原始字典列表中所有不同的名称值,并且其“age”键的值是该名称的年龄总和,则:
from collections import defaultdict
l = [
{"name":"joe", "age":20},
{"name":"tom", "age":35},
{"name":"joe", "age":46}
]
d = defaultdict(int)
for the_dict in l:
d[the_dict["name"]] += the_dict["age"]
new_l = [{"name": k, "age": v} for k, v in d.items()]
print(new_l)
印刷:
[{'name': 'joe', 'age': 66}, {'name': 'tom', 'age': 35}]
您可以使用collections.Counter
并像下面这样update
:
from collections import Counter
people_list = [{"name":"joe", "age":20}, {"name":"tom", "age":35}, {"name":"joe", "age":46}]
cnt = Counter()
for dct in people_list:
cnt.update(dct.items())
print(cnt)
# Get the items > 1
for k,v in cnt.items():
if v>1:
print(k)
Output:
Counter({('name', 'joe'): 2, ('age', 20): 1, ('name', 'tom'): 1, ('age', 35): 1, ('age', 46): 1})
('name', 'joe')
如果你想得到 output “joe”,因为它针对键“name”出现了不止一次,那么:
from collections import Counter
people_list = [{"name":"joe", "age":20}, {"name":"tom", "age":35}, {"name":"joe", "age":46}]
counter = Counter([person["name"] for person in people_list])
print([name for name, count in counter.items() if count > 1]) # ['joe']
您可以使用Counter
来统计每个值出现的次数,并检查是否有任何键值对出现超过一次。
from collections import Counter
people_list = [{"name":"joe", "age":20}, {"name":"tom", "age":35}, {"name":"joe", "age":46}]
# Count the number of occurrences of each value
counts = Counter(d["name"] for d in people_list)
# Check if any key-value pair appears more than once
for key, value in counts.items():
if value > 1:
print(f"{key} appears more than once")
给出:
joe appears more than once
我会将每一对转换为字符串表示形式,然后将其放在列表中。 之后,您可以使用该问题中已经存在的Counter
逻辑。 虽然这不是处理数据的最有效方法,但它仍然是一个简单的解决方案。
from functools import reduce
from collections import Counter
people_list = [{"name":"joe", "age":20}, {"name":"tom", "age":35}, {"name":"joe", "age":46}]
str_lists = [[f'{k}_{v}' for k,v in value.items()] for value in people_list]
# [['name_joe', 'age_20'], ['name_tom', 'age_35'], ['name_joe', 'age_46']]
normalized_list = list(reduce(lambda a,b: a+b,str_lists))
# ['name_joe', 'age_20', 'name_tom', 'age_35', 'name_joe', 'age_46']
dict_ = dict(Counter(normalized_list))
# {'name_joe': 2, 'age_20': 1, 'name_tom': 1, 'age_35': 1, 'age_46': 1}
print([k for k,v in dict_.items() if v>1])
# ['name_joe']
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.