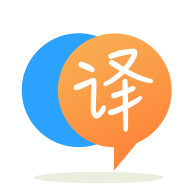
[英]How do I save a custom class to user settings using ApplicationSettingsBase?
[英]How do I save/serialize a custom class to the settings file?
我有一个包含两个字符串的小类,如下所示:
public class ReportType
{
private string displayName;
public string DisplayName
{
get { return displayName; }
}
private string reportName;
public string ReportName
{
get { return reportName; }
}
public ReportType(string displayName, string reportName)
{
this.displayName = displayName;
this.reportName = reportName;
}
}
我想将此类的一个实例保存到我的设置文件中,以便我可以执行以下操作:
ReportType reportType = Settings.Default.SelectedReportType;
谷歌搜索似乎表明这是可能的,但似乎没有任何地方可供我遵循的明确指南。 我知道需要一些序列化,但真的不知道从哪里开始。 此外,当我进入 Visual Studio 中的“设置”屏幕并单击“类型”列下的“浏览”时,没有选择包含 ReportType 类的当前命名空间的选项。
好吧,我想我最终已经解决了。 首先要做的是在需要序列化的ReportType类的每个属性中添加以下属性,并从ApplicationSettingsBase继承该类:
public class ReportType : ApplicationSettingsBase
{
private string displayName;
[UserScopedSetting()]
[SettingsSerializeAs(System.Configuration.SettingsSerializeAs.Xml)]
public string DisplayName
{
get { return displayName; }
}
………………
然后,一旦您重新构建了您的程序集(重要!),您可以进入设置屏幕并单击浏览,然后在底部的文本框中键入您的命名空间和类名(例如 Label_Creator.ReportType)。 命名空间和类名没有出现在树中,所以这部分不是很明显你需要做什么,这就是为什么它有点混乱......
@Calanus 解决方案对我来说并不适用(在 Visual Studio 2015 上)。 缺少的步骤实际上是设置或从实际设置中获取。 至于原来的问题,实现一个简单的 POCO 可以这样实现:
[Serializable]
public class ReportType
{
public string DisplayName { get; set; }
public string ReportName { get; set; }
public ReportType() { }
public ReportType(string displayName, string reportName)
{
DisplayName = displayName;
ReportName = reportName;
}
}
// the class responsible for reading and writing the settings
public sealed class ReportTypeSettings : ApplicationSettingsBase
{
[UserScopedSetting]
[SettingsSerializeAs(SettingsSerializeAs.Xml)]
[DefaultSettingValue("")]
public ReportType ReportType
{
get { return (ReportType)this[nameof(ReportType)]; }
set { this[nameof(ReportType)] = value; }
}
}
我已经用于实际序列化列表:
[Serializable]
public class Schedule
{
public Schedule() : this(string.Empty, DateTime.MaxValue)
{
}
public Schedule(string path, DateTime terminationTime)
{
path = driverPath;
TerminationTime = terminationTime;
}
public DateTime TerminationTime { get; set; }
public string Path { get; set; }
}
public sealed class Schedules : ApplicationSettingsBase
{
[UserScopedSetting]
[SettingsSerializeAs(SettingsSerializeAs.Xml)]
[DefaultSettingValue("")]
public List<Schedule> Entries
{
get { return (List<Schedule>)this[nameof(Entries)]; }
set { this[nameof(Entries)] = value; }
}
}
实例化一个 Schedules (ReportTypeSettings) 对象。 它会自动读取设置。 您可以使用 Reload 方法进行刷新。 例如:
ReportTypeSettings rts = new ReportTypeSettings();
rts.Reload();
rts.ReportType = new ReportType("report!", "report1");
rts.Save();
重要说明:
如何创建一个静态方法,该方法返回包含配置文件中数据的 ReportType 实例。 它更简单,我认为不需要序列化。
public class ReportType
{
public static ReportType GetDefaultSelectedReportType()
{
string displayName = ConfigurationManager.AppSettings["DefaultDisplayName"];
string reportName = ConfigurationManager.AppSettings["DefaultReportName"];
return new ReportType(displayName, reportName);
}
.
.
.
}
只是比查理更清晰的代码
public class ReportType
{
public static ReportType CreateDefaults()
{
return new ReportType
{
DisplayName = ConfigurationManager.AppSettings["DefaultDisplayName"],
ReportName = ConfigurationManager.AppSettings["DefaultReportName"]
};
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.