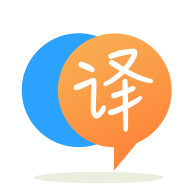
[英]Python: Read all config values from .ini file and store in Dictionary and access in other files
[英]Read all the contents in ini file into dictionary with Python
通常,我按如下方式编码,以获取变量中的特定项目,如下所示
try:
config = ConfigParser.ConfigParser()
config.read(self.iniPathName)
except ConfigParser.MissingSectionHeaderError, e:
raise WrongIniFormatError(`e`)
try:
self.makeDB = config.get("DB","makeDB")
except ConfigParser.NoOptionError:
self.makeDB = 0
有没有办法读取python字典中的所有内容?
例如
[A] x=1 y=2 z=3 [B] x=1 y=2 z=3
被写入
val["A"]["x"] = 1 ... val["B"]["z"] = 3
我建议ConfigParser.ConfigParser
(或SafeConfigParser
,&c)以安全地访问“受保护”属性(以单下划线开头的名称 - “private”将是以两个下划线开头的名称,即使在子类中也不能访问...) :
import ConfigParser
class MyParser(ConfigParser.ConfigParser):
def as_dict(self):
d = dict(self._sections)
for k in d:
d[k] = dict(self._defaults, **d[k])
d[k].pop('__name__', None)
return d
这模拟了配置解析器的通常逻辑,并保证在所有版本的Python中都有效,其中有一个ConfigParser.py
模块(最多2.7个,这是2.*
系列中的最后一个 - 知道没有未来Python 2.任何版本都可以保证兼容性;-)。
如果你需要支持未来的Python 3.*
版本(最多3.1版本,可能是即将推出的3.2版本应该没问题,只需将模块重命名为全小写的configparser
而不是当然)它可能需要一些关注/调整几年这条路,但我不指望有什么重大的。
我设法得到答案,但我希望应该有一个更好的答案。
dictionary = {}
for section in config.sections():
dictionary[section] = {}
for option in config.options(section):
dictionary[section][option] = config.get(section, option)
ConfigParser的实例数据在内部存储为嵌套的dict。 您可以复制它,而不是重新创建它。
>>> import ConfigParser
>>> p = ConfigParser.ConfigParser()
>>> p.read("sample_config.ini")
['sample_config.ini']
>>> p.__dict__
{'_defaults': {}, '_sections': {'A': {'y': '2', '__name__': 'A', 'z': '3', 'x': '1'}, 'B': {'y': '2', '__name__': 'B', 'z': '3', 'x': '1'}}, '_dict': <type 'dict'>}
>>> d = p.__dict__['_sections'].copy()
>>> d
{'A': {'y': '2', '__name__': 'A', 'z': '3', 'x': '1'}, 'B': {'y': '2', '__name__': 'B', 'z': '3', 'x': '1'}}
编辑:
Alex Martelli的解决方案更清洁,更强大,更漂亮。 虽然这是公认的答案,但我建议改用他的方法。 有关详细信息,请参阅他对此解决方案的评论
我知道这个问题是5年前提出的,但是今天我已经把这个词汇理解得很好了:
parser = ConfigParser()
parser.read(filename)
confdict = {section: dict(parser.items(section)) for section in parser.sections()}
如何在py中解析ini文件?
import ConfigParser
config = ConfigParser.ConfigParser()
config.read('/var/tmp/test.ini')
print config.get('DEFAULT', 'network')
test.ini文件包含:
[DEFAULT]
network=shutup
others=talk
需要注意的另一件事是, ConfigParser
将键值转换为小写,因此如果您将配置条目转换为字典,请检查您的要求。 因为这个我遇到了问题。 对我来说,我有骆驼式密钥,因此,当我开始使用字典而不是文件时,必须更改一些代码。 ConfigParser.get()
方法在内部将密钥转换为小写。
假设file:config.properties包含以下内容:
python代码:
def read_config_file(file_path):
with open(file=file_path, mode='r') as fs:
return {k.strip(): v.strip() for i in [l for l in fs.readlines() if l.strip() != ''] for k, v in [i.split('=')]}
print('file as dic: ', read_config_file('config.properties'))
来自https://wiki.python.org/moin/ConfigParserExamples
def ConfigSectionMap(section):
dict1 = {}
options = Config.options(section)
for option in options:
try:
dict1[option] = Config.get(section, option)
if dict1[option] == -1:
DebugPrint("skip: %s" % option)
except:
print("exception on %s!" % option)
dict1[option] = None
return dict1
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.