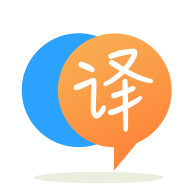
[英]How to convert unsigned int (pointer) to const char in C++
[英]How to convert from const char* to unsigned int c++
我是 C++ 编程的新手,我一直在尝试从 const char* 转换为 unsigned int ,但没有运气。 我有一个:
const char* charVar;
我需要将其转换为:
unsigned int uintVar;
如何在 C++ 中完成?
谢谢
#include <iostream>
#include <sstream>
const char* value = "1234567";
stringstream strValue;
strValue << value;
unsigned int intValue;
strValue >> intValue;
cout << value << endl;
cout << intValue << endl;
输出:
1234567
1234567
你说的转换是什么意思?
如果您正在谈论从文本中读取整数,那么您有多种选择。
提升词法转换: http : //www.boost.org/doc/libs/1_44_0/libs/conversion/lexical_cast.htm
字符串流:
const char* x = "10";
int y;
stringstream s(x);
s >> y;
或者好的旧 C 函数atoi()
和strtol()
如果您真的想将指向常量字符的指针转换为无符号整数,那么您应该在 C++ 中使用:
const char* p;
unsigned int i = reinterpret_cast<unsigned int>( p );
这会将指针指向的地址转换为无符号整数。
如果要将指针指向的内容转换为 unsigned int,则应使用:
const char* p;
unsigned int i = static_cast<unsigned int>( *p );
如果您想从字符串中获取整数,从而将 const char* 解释为指向 const char 数组的指针,则可以使用上述解决方案之一。
C方式:
#include <stdlib.h>
int main() {
const char *charVar = "16";
unsigned int uintVar = 0;
uintVar = atoi(charVar);
return 0;
}
C++方式:
#include <sstream>
int main() {
istringstream myStream("16");
unsigned int uintVar = 0;
myStream >> uintVar;
return 0;
}
请注意,在这两种情况下,我都没有检查转换的返回码以确保它确实有效。
在 C 中,这可以使用atoi
来完成,它也可以通过cstdlib
用于 C++。
我通常使用这个通用函数将字符串转换为“任何东西”:
#include <sstream>
// Convert string values into type T results.
// Returns false in case the conversion fails.
template <typename T>
bool getValueFromString( const std::string & value, T & result )
{
std::istringstream iss( value );
return !( iss >> result ).fail();
}
只需将其用作:
int main()
{
const char * a_string = "44";
unsigned int an_int;
bool success;
// convert from const char * into unsigned int
success = getValueFromString( a_string, an_int );
// or any other generic convertion
double a;
int b;
float c;
// conver to into double
success = getValueFromString( "45", a );
// conve rto into int
success = getValueFromString( "46", b );
// conver to into float
success = getValueFromString( "47.8", c );
}
所以我知道这是旧的,但我想我会提供一种更有效的方法来做到这一点,这会给你一些你想要的基础的灵活性。
#include<iostream>
unsigned long cstring_to_ul(const char* str, char** end = nullptr, int base = 10)
{
errno = 0; // Used to see if there was success or failure
auto ul = strtoul(str, end, base);
if(errno != ERANGE)
{
return ul;
}
return ULONG_MAX;
}
这将做的是围绕 C 中的方法 strtoul(const char* nptr, char** endptr, int base) 方法创建一个包装器。有关此函数的更多信息,您可以在此处阅读手册页中的说明https:// linux.die.net/man/3/strtoul
失败时,您将获得 errno = ERANGE,这将允许您在调用此函数以及值为 ULONG_MAX 后进行检查。
使用它的一个例子如下:
int main()
{
unsigned long ul = cstring_to_ul("3284201");
if(errno == ERANGE && ul == ULONG_MAX)
{
std::cout << "Input out of range of unsigned long.\n";
exit(EXIT_FAILURE);
}
std::cout << "Output: " << ul << "\n";
}
这将给出输出
输出:3284201
const char* charVar = "12345";
unsigned int uintVar;
try {
uintVar = std::stoi( std::string(charVar) );
}
catch(const std::invalid_argument& e) {
std::cout << "Invalid Arg: " << e.what() << endl;
}
catch(const std::out_of_range& e) {
std::cout << "Out of range: " << e.what() << endl;
}
您还可以使用strtoul
或_tcstoul
从const char*
获取 unsigned long 值,然后将该值转换为 unsigned int。
http://msdn.microsoft.com/en-us/library/5k9xb7x1(v=vs.71).aspx
const char* charVar = "1864056953";
unsigned int uintVar = 0;
for (const char* it = charVar; *it != 0; *it++){
if ((*it < 48) || (*it > 57)) break; // see ASCII table
uintVar *= 10; // overflow may occur
uintVar += *it - 48; //
}
std::cout << uintVar << std::endl;
std::cout << charVar << std::endl;
如果没有更多信息,就无法正确回答这个问题。 你到底想转换什么? 如果 charVar 是字符串的 ascii 表示,那么您可以像其他人建议的那样使用 stringstreams、atoi、sscanf 等。
如果您想要 charVar 指向的实际值,那么您需要类似的东西:
intValue = (unsigned int)(*charVal);
或者,如果 charVal 是指向无符号整数的第一个字节的指针,则:
intValue = *((unsigned int*)(charVal));
用这种方法试试
#include<iostream>
#include <typeinfo> //includes typeid
using namespace std;
int main(){
char a = '3';
int k = 3;
const char* ptr = &a;
cout << typeid(*ptr).name() << endl; //prints the data type c = char
cout << typeid(*ptr-'0').name() << endl; //prints the data type i = integer
cout << *ptr-'0' << endl;
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.