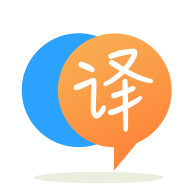
[英]Comparing objects from two instances of a Class and storing result in List<class>
[英]Respond to events from a list of class instances
我有一个.NET类InstrumentConnector,它具有一个事件StatusChanged。 我想创建此类的几个实例(每个类处理一个单独的文件),并在任何实例引发StatusChanged事件时做出响应。 我将如何在.NET中执行此操作? 我是否要创建一个List(Of InstrumentConnector)或类似的IEnumerable? 如何编写事件处理程序以响应特定实例的StatusChanged事件?
这是InstrumentConnector类和带有StatusChanged处理函数的模块:
Public Class InstrumentConnector
Private _inProcessDir As String
Private _doneDir As String
Private _dataFileName As String
Public ReadOnly Property DataFileName() As String
Get
Return _dataFileName
End Get
End Property
Private WithEvents _processTimer As Timers.Timer
Private _fileStatus As Status
Public ReadOnly Property FileStatus() As Status
Get
Return _fileStatus
End Get
End Property
Public Sub New(ByVal inProcessDirectory As String, ByVal doneDirectory As String)
_inProcessDir = inProcessDirectory
_doneDir = doneDirectory
_fileStatus = Status.Waiting
End Sub
Public Sub New(ByVal inProcessDirectory As String)
Me.New(inProcessDirectory, IO.Path.Combine(inProcessDirectory, "done"))
End Sub
Private Sub InitializeTimer()
_processTimer = New Timers.Timer
With _processTimer
.Interval = 1000
End With
End Sub
Public Sub Process(ByVal dataFileName As String)
_dataFileName = IO.Path.GetFileName(dataFileName)
InitializeTimer()
_processTimer.Start()
End Sub
Private Sub _processTimer_Elapsed(ByVal sender As Object, ByVal e As System.Timers.ElapsedEventArgs) Handles _processTimer.Elapsed
'1. Look for file in Done directory
'2. Evaluate its approval status
'3. If not completed change approval setting in file and move to InProcess directory
If IsFileInProcessDirectory() = True Then
MoveFileToDoneDirectory()
Else
If IsFileInDoneDirectory() = True Then
If IsFileApproved() = True Then
_fileStatus = Status.Accepted
RaiseEvent StatusChanged(Nothing)
Else
If NeedsSecondAttempt() = True Then
MoveFileToProcessDirectory()
Else
_fileStatus = Status.Rejected
RaiseEvent StatusChanged(Nothing)
End If
End If
End If
End If
End Sub
Private Sub MoveFileToDoneDirectory()
Dim fileContents As String
fileContents = My.Computer.FileSystem.ReadAllText(IO.Path.Combine(_inProcessDir, _dataFileName))
If GetFileApprovalStatus() = True Then
fileContents = String.Concat("9999", fileContents.Substring(4))
End If
My.Computer.FileSystem.WriteAllText(IO.Path.Combine(_doneDir, _dataFileName), fileContents, False)
My.Computer.FileSystem.DeleteFile(IO.Path.Combine(_inProcessDir, _dataFileName))
End Sub
Private Function NeedsSecondAttempt() As Boolean
Dim fileContents As String
fileContents = My.Computer.FileSystem.ReadAllText(IO.Path.Combine(_doneDir, _dataFileName))
If fileContents.StartsWith("9999") And fileContents.Substring(111, 1) = "1" Then
Return False
Else
Return True
End If
End Function
Private Sub MoveFileToProcessDirectory()
Dim fileContents As String
fileContents = My.Computer.FileSystem.ReadAllText(IO.Path.Combine(_doneDir, _dataFileName))
fileContents = fileContents.Remove(111, 1).Insert(111, "1")
My.Computer.FileSystem.WriteAllText(IO.Path.Combine(_doneDir, _dataFileName), fileContents, False)
My.Computer.FileSystem.MoveFile(IO.Path.Combine(_doneDir, _dataFileName), IO.Path.Combine(_inProcessDir, _dataFileName))
End Sub
Private Function IsFileInDoneDirectory() As Boolean
Dim fileExists As Boolean
fileExists = My.Computer.FileSystem.FileExists(IO.Path.Combine(_doneDir, _dataFileName))
Return fileExists
End Function
Private Function IsFileInProcessDirectory() As Boolean
Dim fileExists As Boolean
fileExists = My.Computer.FileSystem.FileExists(IO.Path.Combine(_inProcessDir, _dataFileName))
Return fileExists
End Function
Private Function IsFileApproved() As Boolean
Dim fileContents As String
fileContents = My.Computer.FileSystem.ReadAllText(IO.Path.Combine(_doneDir, _dataFileName))
If fileContents.Substring(0, 4) = "9999" Then
Return True
Else
Return False
End If
End Function
Private Function GetFileApprovalStatus() As Boolean
Dim randNum As New Random
Dim nextNum = randNum.Next(1, 10)
'60% of all samples will be approved
If nextNum > 6 Then
Return False
Else
Return True
End If
End Function
Public Event StatusChanged(ByVal e As System.EventArgs)
Protected Overridable Sub OnStatusChanged(ByVal e As System.EventArgs) Handles Me.StatusChanged
_processTimer.Stop()
End Sub
Protected Overrides Sub Finalize()
MyBase.Finalize()
_processTimer.Stop()
End Sub
Public Enum Status
Accepted
Rejected
Waiting
End Enum
末级
Module Module1
Private WithEvents detector As NewFileDetector
Private WithEvents newIC As InstrumentConnector
Private ICList As New List(Of InstrumentConnector)
Private fileDirectory As String
Sub Main()
fileDirectory = "c:\temp\inproc"
Console.WriteLine("Mock IC program running...")
Console.WriteLine()
Console.WriteLine("Press ESC to quit...")
detector = New NewFileDetector(fileDirectory)
Dim keyresponse As ConsoleKeyInfo = Nothing
Do
keyresponse = Console.ReadKey
Loop Until keyresponse.Key = ConsoleKey.Escape 'Quit program on ESC
End Sub
Private Sub detector_FilesFound(ByVal e As System.EventArgs) Handles detector.FilesFound
Console.WriteLine("Files found: {0}", detector.FileList.Count)
For Each filename In detector.FileList
newIC = New InstrumentConnector(fileDirectory)
ICList.Add(newIC)
newIC.Process(filename)
Next
End Sub
Private Sub newIC_StatusChanged(ByVal e As System.EventArgs) Handles newIC.StatusChanged
Console.WriteLine("File: {0} Status: {1}", newIC.DataFileName, newIC.FileStatus.ToString)
ICList.Remove(newIC)
End Sub
终端模块
在这方面有点晚了,但是:这正是Microsoft的CAB框架解决的问题。 它允许任何事件具有多个订阅者和多个发布者。
有了这个框架提供的一个开放源码的版本在这里 。
事件的存储方式与InstrumentConnectors的存储方式无关。 一个List(Of T)
很好。
您可以将侦听器类连接到多个事件源。 这就是.NET中的标准模式具有sender
参数的原因。 只需将其投射到InstrumentConnector即可。
也就是说,只要StatusChanged是根据规则设计的。
现在有太多代码。 很难通过。
Public Event StatusChanged(ByVal e As System.EventArgs)
没有正确定义。 应该是
Public Event StatusChanged(ByVal sender as Object, ByVal e As System.EventArgs)
而且,我的VB有点生疏,在“句柄”符号旁边,您应该可以声明(在某处)
HandlesEvent Me.StatusChangedHandler, someInstrument.Statuschanged ' very sloppy approx
然后在您选择的课程中(可能是表格)
Sub StatusChangedHandler(ByVal sender as Object, ByVal e As System.EventArgs)
Dim InsConn as InstrumentConnector = sender ' may need a CType() here
....
End Sub
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.