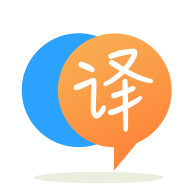
[英]Set Image on UIImageView using UIImagePickerControllerReferenceURL
[英]Set UIImageView image using a url
是否可以读取图像的URL并将UIImageView设置为此URL的图像?
NSString *ImageURL = @"YourURLHere";
NSData *imageData = [NSData dataWithContentsOfURL:[NSURL URLWithString:ImageURL]];
imageView.image = [UIImage imageWithData:imageData];
对于这样一个简单的任务,我强烈建议不要集成像Three20这样的项目,这个库是一个怪物,不是很容易起床和运行。
我会推荐这种方法:
NSString *imageUrl = @"http://www.foo.com/myImage.jpg";
[NSURLConnection sendAsynchronousRequest:[NSURLRequest requestWithURL:[NSURL URLWithString:imageUrl]] queue:[NSOperationQueue mainQueue] completionHandler:^(NSURLResponse *response, NSData *data, NSError *error) {
myImageView.image = [UIImage imageWithData:data];
}];
let urlString = "http://www.foo.com/myImage.jpg"
guard let url = URL(string: urlString) else { return }
URLSession.shared.dataTask(with: url) { (data, response, error) in
if error != nil {
print("Failed fetching image:", error)
return
}
guard let response = response as? HTTPURLResponse, response.statusCode == 200 else {
print("Not a proper HTTPURLResponse or statusCode")
return
}
DispatchQueue.main.async {
self.myImageView.image = UIImage(data: data!)
}
}.resume()
*编辑Swift 2.0
let request = NSURLRequest(URL: NSURL(string: urlString)!)
NSURLSession.sharedSession().dataTaskWithRequest(request) { (data, response, error) -> Void in
if error != nil {
print("Failed to load image for url: \(urlString), error: \(error?.description)")
return
}
guard let httpResponse = response as? NSHTTPURLResponse else {
print("Not an NSHTTPURLResponse from loading url: \(urlString)")
return
}
if httpResponse.statusCode != 200 {
print("Bad response statusCode: \(httpResponse.statusCode) while loading url: \(urlString)")
return
}
dispatch_async(dispatch_get_main_queue(), { () -> Void in
self.myImageView.image = UIImage(data: data!)
})
}.resume()
可以从URL加载NSData并将其转换为图像并将其粘贴到图像视图中,但这是一个非常糟糕的主意,因为它涉及在主线程上执行同步URL下载。 这将锁定UI并可能导致用户认为如果图像下载速度非常快,您的应用程序已崩溃。
编辑 :为了澄清,原来的问题在我看来就像海报想要说的一样
imageView.image = [UIImage imageWithContentsOfURL:theURL];
因此我的答案。 通过NSData可以做到相同,但这是一个坏主意。 相反,应该使用NSURLConnection异步下载图像,并且只有在完全下载后才能将其转换为UIImage并分配给图像视图。
我正在使用https://github.com/rs/SDWebImage这是一个设计精美的库,它可以选择放置占位符图像,无论是内存还是磁盘缓存图像或使用独立于UIImageView
的下载程序。
我试图自己做,但图书馆消除了所有的痛苦。
您需要做的就是为您的案例编写以下代码:
#import "UIImageView+WebCache.h"
[imageView sd_setImageWithURL:[NSURL URLWithString:@"http://www.domain.com/path/to/image.jpg"] placeholderImage:[UIImage imageNamed:@"placeholder.png"]];
对我来说就像一个魅力。
如果你想在ImageView中显示来自Url的图像,并且还希望将此图像保存在缓存中以优化到服务器交互,这将有助于你只需将imageView对象和字符串Url传递给此函数
-(void)downloadingServerImageFromUrl:(UIImageView*)imgView AndUrl:(NSString*)strUrl{
strUrl = [strUrl encodeUrl];
NSString* theFileName = [NSString stringWithFormat:@"%@.png",[[strUrl lastPathComponent] stringByDeletingPathExtension]];
NSFileManager *fileManager =[NSFileManager defaultManager];
NSString *fileName = [NSHomeDirectory() stringByAppendingPathComponent:[NSString stringWithFormat:@"tmp/%@",theFileName]];
imgView.backgroundColor = [UIColor darkGrayColor];
UIActivityIndicatorView *actView = [[UIActivityIndicatorView alloc]initWithActivityIndicatorStyle:UIActivityIndicatorViewStyleWhite];
[imgView addSubview:actView];
[actView startAnimating];
CGSize boundsSize = imgView.bounds.size;
CGRect frameToCenter = actView.frame;
// center horizontally
if (frameToCenter.size.width < boundsSize.width)
frameToCenter.origin.x = (boundsSize.width - frameToCenter.size.width) / 2;
else
frameToCenter.origin.x = 0;
// center vertically
if (frameToCenter.size.height < boundsSize.height)
frameToCenter.origin.y = (boundsSize.height - frameToCenter.size.height) / 2;
else
frameToCenter.origin.y = 0;
actView.frame = frameToCenter;
dispatch_queue_t queue = dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0);
dispatch_async(queue, ^{
NSData *dataFromFile = nil;
NSData *dataFromUrl = nil;
dataFromFile = [fileManager contentsAtPath:fileName];
if(dataFromFile==nil){
dataFromUrl=[[[NSData alloc] initWithContentsOfURL:[NSURL URLWithString:strUrl]] autorelease];
}
dispatch_sync(dispatch_get_main_queue(), ^{
if(dataFromFile!=nil){
imgView.image = [UIImage imageWithData:dataFromFile];
}else if(dataFromUrl!=nil){
imgView.image = [UIImage imageWithData:dataFromUrl];
NSString *fileName = [NSHomeDirectory() stringByAppendingPathComponent:[NSString stringWithFormat:@"tmp/%@",theFileName]];
BOOL filecreationSuccess = [fileManager createFileAtPath:fileName contents:dataFromUrl attributes:nil];
if(filecreationSuccess == NO){
NSLog(@"Failed to create the html file");
}
}else{
imgView.image = [UIImage imageNamed:@"NO_Image.png"];
}
[actView removeFromSuperview];
[actView release];
[imgView setBackgroundColor:[UIColor clearColor]];
});
});
}
这是最佳答案的更新答案,因为imageWithContentsOfURL不再是UIImage的方法。 你必须使用CIImage:
NSURL *url = [NSURL URLWithString:@"http://url_goes_here.com/logo.png"];
imageView.image = [UIImage imageWithCIImage:[CIImage imageWithContentsOfURL:url]];
不幸的是,在撰写本文时,此功能无法使用...相反,您必须自己实现以下功能:
幸运的是,您不必因为所述功能而出现问题,因为Apple提供了一个示例,可以作为其代码示例的一部分。
遵循代码,我相信您将能够满足您的需求。
EGOImageView* imageView = [[EGOImageView alloc] initWithPlaceholderImage:[UIImage imageNamed:@"placeholder.png"]];
imageView.frame = CGRectMake(0.0f, 0.0f, 36.0f, 36.0f);
//show the placeholder image instantly
[self.anyView addSubview:imageView];
[imageView release] //if you want
//load the image from url asynchronously with caching automagically
imageView.imageURL = [NSURL URLWithString:photoURL];
如果你想要更多,有一个委托来加载后处理动作
@protocol EGOImageViewDelegate<NSObject>
@optional
- (void)imageViewLoadedImage:(EGOImageView*)imageView;
- (void)imageViewFailedToLoadImage:(EGOImageView*)imageView error:(NSError*)error;
@end
另一个选项是来自Three20库的TTImageView,它处理图像的异步加载。 控制效果很好。 然而,缺点是你必须处理安装Three20的噩梦(这几乎是不可能完全删除)。
使用AFNetworking
类别UIImageView+AFNetworking.h
#import "UIImageView+AFNetworking.h
[profileImageView setImageWithURL:[NSURL URLWithString:photoURL] placeholderImage:[UIImage imageNamed:@"placeholder"]];
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.