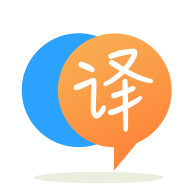
[英]Javascript - Find position of specific occurrence of a word in a string (not first or last)
[英]How to find out the position of the first occurrence of the difference between the two string?
例如, Hello World!
和Hi World!
- 差异的第一次出现是第二个字符。 什么是JavaScript / jQuery函数?
假设,与其他答案一样,匹配的字符串返回-1
:
// Find common prefix of strings a and b. var prefix = function(a,b){ return a && a[0] === b[0] ? a[0] + prefix(a.slice(1), b.slice(1)) : ''; }; // Find index of first difference. var diff = function(a,b){ return a===b ? -1 : prefix(a,b).length; }; var tests = [ ['Hello World!', 'Hi World!'], ['aaabab', 'aaabzbzz'], ['', ''], ['abc', 'abc'], ['qrs', 'tu'], ['abc', ''], ['', 'abc'] ]; console.log('diff', tests.map(test => diff(test[0], test[1]))); // Or just count up to the first difference // Trickier nested ternary to handle the -1 however. var diff2 = function(a,b){ return a === b ? -1 : a[0] === b[0] ? 1 + diff2(a.slice(1), b.slice(1)) : 0; }; console.log('diff2', tests.map(test => diff2(test[0], test[1])));
也许是这样的? 它按顺序返回第一个差异的位置(如果有的话),最短字符串的长度(如果有的话),或者如果一切都相等则返回-1。
function findDiff(a, b) {
a = a.toString();
b = b.toString();
for (var i = 0; i < Math.min(a.length, b.length); i++) {
if (a.charAt(i) !== b.charAt(i)) { return i; }
}
if (a.length !== b.length) { return Math.min(a.length, b.length); }
return -1;
}
感谢Phil的建议!
function firstDiff(a, b) {
var i = 0;
while (a.charAt(i) === b.charAt(i))
if (a.charAt(i++) === '')
return -1;
return i;
}
返回两个字符串a和b首先不同的位置,如果它们相等,则返回-1。
更高效但可读性更低的版本:
function firstDiff(a, b) {
for (var i = 0, c; (c = a.charAt(i)) === b.charAt(i); ++i)
if (c === '')
return -1;
return i;
}
如果您认为首先应对参数进行字符串化,那么请在调用中进行:
firstDiff(toString(a), toString(b))
大多数情况下,这将是浪费时间。 了解你的数据!
function strDiff(first, second) {
if(first==second)
return -1;
first = first.toString();
second = second.toString();
var minLen = min(first.length,second.length);
for(var i = 0; i<minLen; i++) {
if(first.charAt(i) != second.charAt(i)) {
return i;
}
}
return minLen;
}
如果字符串没有不同,则返回-1,或者它们所执行的字符的索引(从0开始)(如果它们的长度不同,则为最短字符串的长度,例如'abcd'和'abcdef '会回来4。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.