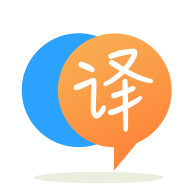
[英]Getting a value of key from xml and change the key value to xml in c#?
[英]Getting a value of a key from an XML file in C#
我有一个具有以下结构的 XML 文件:
<Definitions>
<Definition Execution="Firstkey" Name="Somevalue"></Definition>
<Definition Execution="Secondkey" Name="Someothervalue"></Definition>
</Definitions>
如何在我的 .NET 应用程序中获取键(Firstkey、Secondkey)的值并使用 C# 将它们写下来?
谢谢。
使用 Linq 到 XML 这很简单。
只需获取密钥:
var keys = doc.Descendants("Definition")
.Select(x => x.Attribute("Execution").Value);
foreach (string key in keys)
{
Console.WriteLine("Key = {0}", key);
}
获取所有值:
XDocument doc = XDocument.Load("test.xml");
var definitions = doc.Descendants("Definition")
.Select(x => new { Execution = x.Attribute("Execution").Value,
Name = x.Attribute("Name").Value });
foreach (var def in definitions)
{
Console.WriteLine("Execution = {0}, Value = {1}", def.Execution, def.Name);
}
编辑以回应评论:
我认为你真正想要的是一个字典,它从一个键(“执行”)映射到一个值(“名称”):
XDocument doc = XDocument.Load("test.xml");
Dictionary<string, string> dict = doc.Descendants("Definition")
.ToDictionary(x => x.Attribute("Execution").Value,
x => x.Attribute("Name").Value);
string firstKeyValue = dict["Firstkey"]; //Somevalue
使用 XMLDocumnet/XMLNode(s):
//Load the XML document into memory
XmlDocument doc = new XmlDocument();
doc.Load("myXML.xml");
//get a list of the Definition nodes in the document
XmlNodeList nodes = doc.GetElementsByTagName("Definition");
//loop through each node in the XML
foreach (XmlNode node in nodes)
{
//access the key attribute, since it is named Execution,
//that is what I pass as the index of the attribute to get
string key = node.Attributes["Execution"].Value;
//To select a single node, check if we have the right key
if(key == "SecondKey") //then this is the second node
{
//do stuff with it
}
}
基本上,您将 xml 加载到文档变量 select 您要查看的节点中。 然后遍历它们并存储相关信息。
using System.Xml.Linq;
var keys = XDocument.Load("path to the XML file")
.Root
.Elements()
.Select(x => x.Attribute("Execution"));
XPath将是一个不错的选择,我会说。
下面是一个示例 XPath 表达式。
//Definition[@Execution='Firstkey']/@Name
由于 XPath 表达式是一个字符串,您可以轻松地将“Firstkey”替换为您需要的任何内容。
将此与XmlDocument.SelectSingleNode或XmlDocument.SelectNodes方法一起使用
上述两种方法都返回一个 XmlNode。 您可以轻松访问XmlNode.Value
不要忘记XPath Visualizer ,它使 XPath 的工作变得如此轻松!
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.