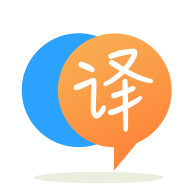
[英]Getting a value of key from xml and change the key value to xml in c#?
[英]Getting a value of a key from an XML file in C#
我有一個具有以下結構的 XML 文件:
<Definitions>
<Definition Execution="Firstkey" Name="Somevalue"></Definition>
<Definition Execution="Secondkey" Name="Someothervalue"></Definition>
</Definitions>
如何在我的 .NET 應用程序中獲取鍵(Firstkey、Secondkey)的值並使用 C# 將它們寫下來?
謝謝。
使用 Linq 到 XML 這很簡單。
只需獲取密鑰:
var keys = doc.Descendants("Definition")
.Select(x => x.Attribute("Execution").Value);
foreach (string key in keys)
{
Console.WriteLine("Key = {0}", key);
}
獲取所有值:
XDocument doc = XDocument.Load("test.xml");
var definitions = doc.Descendants("Definition")
.Select(x => new { Execution = x.Attribute("Execution").Value,
Name = x.Attribute("Name").Value });
foreach (var def in definitions)
{
Console.WriteLine("Execution = {0}, Value = {1}", def.Execution, def.Name);
}
編輯以回應評論:
我認為你真正想要的是一個字典,它從一個鍵(“執行”)映射到一個值(“名稱”):
XDocument doc = XDocument.Load("test.xml");
Dictionary<string, string> dict = doc.Descendants("Definition")
.ToDictionary(x => x.Attribute("Execution").Value,
x => x.Attribute("Name").Value);
string firstKeyValue = dict["Firstkey"]; //Somevalue
使用 XMLDocumnet/XMLNode(s):
//Load the XML document into memory
XmlDocument doc = new XmlDocument();
doc.Load("myXML.xml");
//get a list of the Definition nodes in the document
XmlNodeList nodes = doc.GetElementsByTagName("Definition");
//loop through each node in the XML
foreach (XmlNode node in nodes)
{
//access the key attribute, since it is named Execution,
//that is what I pass as the index of the attribute to get
string key = node.Attributes["Execution"].Value;
//To select a single node, check if we have the right key
if(key == "SecondKey") //then this is the second node
{
//do stuff with it
}
}
基本上,您將 xml 加載到文檔變量 select 您要查看的節點中。 然后遍歷它們並存儲相關信息。
using System.Xml.Linq;
var keys = XDocument.Load("path to the XML file")
.Root
.Elements()
.Select(x => x.Attribute("Execution"));
XPath將是一個不錯的選擇,我會說。
下面是一個示例 XPath 表達式。
//Definition[@Execution='Firstkey']/@Name
由於 XPath 表達式是一個字符串,您可以輕松地將“Firstkey”替換為您需要的任何內容。
將此與XmlDocument.SelectSingleNode或XmlDocument.SelectNodes方法一起使用
上述兩種方法都返回一個 XmlNode。 您可以輕松訪問XmlNode.Value
不要忘記XPath Visualizer ,它使 XPath 的工作變得如此輕松!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.