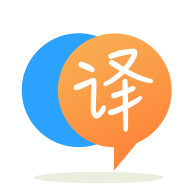
[英]javascript/jquery find id number within multidimensional json object and use the object that id number is in as an object by itself
[英]How can I find the highest value of a particular node within a multidimensional JSON object with JavaScript or jQuery
这是我正在使用的对象的简短示例。
{
"myservices": [
{
"name": "oozie",
"hostidn": "1",
"details": "failed process health monitor....",
"currstatus": "Warning",
"currstatusclass": "warning"
},
{
"name": "oozie",
"hostidn": "2",
"details": "failed process health monitor....",
"currstatus": "Warning",
"currstatusclass": "warning"
},
{
"name": "oozie",
"hostidn": "3",
"details": "failed process health monitor....",
"currstatus": "Warning",
"currstatusclass": "warning"
},
{
"name": "oozie",
"hostidn": "4",
"details": "failed process health monitor....",
"currstatus": "Warning",
"currstatusclass": "warning"
},
{
"name": "oozie",
"hostidn": "5",
"details": "failed process health monitor....",
"currstatus": "Warning",
"currstatusclass": "warning"
},
{
"name": "single-namenode",
"hostidn": "2",
"details": "failed process health monitor....",
"currstatus": "Warning",
"currstatusclass": "warning"
}
]
}
在最终完成所有这些并显示之前,我最终想要找到最高的“hostidn”。 hostidn是第N个数字,它可以是唯一的数字,也可以是数百个,其间有几个重复数字。 我的目标是找到最高的一个,并根据它在它上面做一个for或while循环,在一个可视化显示中将它们组合在一起。 示例通知我下面有一个hostidn,数字2,而其余的都有自己的。 我想把两个一起组合在一个盒子里展示,但在这个场景中有5个不同的hostidn。 我不知道也许我认为它错了我会接受建议。
你可以遵循的基本算法
声明并将变量设置为零
$ currentHighest = 0;
然后迭代json数组,并在每次迭代时使用$currentHighest
的hostidn
值,如果该值高于$currentHighest
已存在的值,则该值为$currentHighest
$currentHighest=0;
$(data.myservices).each(function(index, element){
if(data.myservices[index].hostidn>$currentHighest)
$currentHighest=data.myservices[index].hostidn;
});
//loop ends and `$currentHighest` will have the highest value
在迭代结束时,您将获得$currentHighest
的最高值
试过并测试过
$(function(){
$.post("highest.json",function(data){
$currentHighest=0;
$(data.myservices).each(function(index, element){
if(data.myservices[index].hostidn>$currentHighest)
$currentHighest=data.myservices[index].hostidn;
});
alert($currentHighest);
},'json');
});
返回包含一个或多个idn最高的对象的数组。 另见http://jsfiddle.net/Kai/DUTK7/ (登录到控制台)
function getHighHostIdn (json) {
var i = 0;
hostidns = [],
len = json.myservices.length,
highest = null,
matches = [];
for (; i < len; i++) {
hostidns.push(parseInt(json.myservices[i].hostidn, 10));
}
highest = Math.max.apply(null, hostidns);
for (i = 0, len = hostidns.length; i < len; i++) {
if (hostidns[i] === highest) {
matches.push(json.myservices[i]);
}
}
return matches;
}
我喜欢使用linq.js来做这些事情,它允许你在代码中避免许多传统的for循环(明显地)。 当然,您可以为每个用例编写更多优化代码,但对于大多数情况而言,性能并不那么重要,更清晰/更短的代码是更大的好处。
如果只有一个最大值的机会,或者如果你不关心你得到哪一个,只要它是具有最大值的那个,那么做这样的事情:
var result = Enumerable.From(obj.myservices).MaxBy('$.hostidn');
或者,如果您可以拥有并想要多个最大对象,那么执行以下操作:
var e = Enumerable.From(obj.myservices);
var result2 = e.Where('$.hostidn == ' + e.Max('$.hostidn')).ToArray();
你可以在这里看到代码在运行: http : //jsfiddle.net/sTaHv/13/编辑:我还添加了一个排序示例,因为我看到你提到了。
要了解有关linq.js的更多信息,请访问项目页面: http ://linqjs.codeplex.com/
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.