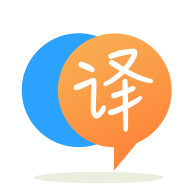
[英]C# VS2010 - Why am I getting the message “An object reference is required for the non-static field”?
[英]Why am I getting “object reference is required for the non-static field, method, or property” error in C#?
我正在尝试在c#中实现AES,我遇到了错误。
到目前为止这是我的代码。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace aes1
{
class Program
{
public byte[,] m_sbox = new byte[16, 16]
{{0x63 ,0x7c ,0x77 ,0x7b ,0xf2 ,0x6b ,0x6f ,0xc5 ,0x30 ,0x01 ,0x67 ,0x2b ,0xfe ,0xd7 ,0xab ,0x76}
,{0xca ,0x82 ,0xc9 ,0x7d ,0xfa ,0x59 ,0x47 ,0xf0 ,0xad ,0xd4 ,0xa2 ,0xaf ,0x9c ,0xa4 ,0x72 ,0xc0}
,{0xb7 ,0xfd ,0x93 ,0x26 ,0x36 ,0x3f ,0xf7 ,0xcc ,0x34 ,0xa5 ,0xe5 ,0xf1 ,0x71 ,0xd8 ,0x31 ,0x15}
,{0x04 ,0xc7 ,0x23 ,0xc3 ,0x18 ,0x96 ,0x05 ,0x9a ,0x07 ,0x12 ,0x80 ,0xe2 ,0xeb ,0x27 ,0xb2 ,0x75}
,{0x09 ,0x83 ,0x2c ,0x1a ,0x1b ,0x6e ,0x5a ,0xa0 ,0x52 ,0x3b ,0xd6 ,0xb3 ,0x29 ,0xe3 ,0x2f ,0x84}
,{0x53 ,0xd1 ,0x00 ,0xed ,0x20 ,0xfc ,0xb1 ,0x5b ,0x6a ,0xcb ,0xbe ,0x39 ,0x4a ,0x4c ,0x58 ,0xcf}
,{0xd0 ,0xef ,0xaa ,0xfb ,0x43 ,0x4d ,0x33 ,0x85 ,0x45 ,0xf9 ,0x02 ,0x7f ,0x50 ,0x3c ,0x9f ,0xa8}
,{0x51 ,0xa3 ,0x40 ,0x8f ,0x92 ,0x9d ,0x38 ,0xf5 ,0xbc ,0xb6 ,0xda ,0x21 ,0x10 ,0xff ,0xf3 ,0xd2}
,{0xcd ,0x0c ,0x13 ,0xec ,0x5f ,0x97 ,0x44 ,0x17 ,0xc4 ,0xa7 ,0x7e ,0x3d ,0x64 ,0x5d ,0x19 ,0x73}
,{0x60 ,0x81 ,0x4f ,0xdc ,0x22 ,0x2a ,0x90 ,0x88 ,0x46 ,0xee ,0xb8 ,0x14 ,0xde ,0x5e ,0x0b ,0xdb}
,{0xe0 ,0x32 ,0x3a ,0x0a ,0x49 ,0x06 ,0x24 ,0x5c ,0xc2 ,0xd3 ,0xac ,0x62 ,0x91 ,0x95 ,0xe4 ,0x79}
,{0xe7 ,0xc8 ,0x37 ,0x6d ,0x8d ,0xd5 ,0x4e ,0xa9 ,0x6c ,0x56 ,0xf4 ,0xea ,0x65 ,0x7a ,0xae ,0x08}
,{0xba ,0x78 ,0x25 ,0x2e ,0x1c ,0xa6 ,0xb4 ,0xc6 ,0xe8 ,0xdd ,0x74 ,0x1f ,0x4b ,0xbd ,0x8b ,0x8a}
,{0x70 ,0x3e ,0xb5 ,0x66 ,0x48 ,0x03 ,0xf6 ,0x0e ,0x61 ,0x35 ,0x57 ,0xb9 ,0x86 ,0xc1 ,0x1d ,0x9e}
,{0xe1 ,0xf8 ,0x98 ,0x11 ,0x69 ,0xd9 ,0x8e ,0x94 ,0x9b ,0x1e ,0x87 ,0xe9 ,0xce ,0x55 ,0x28 ,0xdf}
,{0x8c ,0xa1 ,0x89 ,0x0d ,0xbf ,0xe6 ,0x42 ,0x68 ,0x41 ,0x99 ,0x2d ,0x0f ,0xb0 ,0x54 ,0xbb ,0x16}};
public byte[,] SubBytes(byte[,] input)
{
byte[,] output = new byte[4, 4];
for (int j = 0; j <= 3; j++)
{
for (int k = 0; k <= 3; k++)
{
output[k, j] = Sbox(input[k, j]);
}
}
return output;
}
public byte Sbox(byte input)
{
return m_sbox[input / 16, input % 16];
}
public static byte[] Str2ByteArray(string str)
{
System.Text.UTF8Encoding encoding = new System.Text.UTF8Encoding();
return encoding.GetBytes(str);
}
static void Main(string[] args)
{
byte[,] state = new byte[4, 4];
//byte[,] input = new byte[4, 4];
byte[,] input = new byte[4, 4] {
{ 0xd4, 0xe0, 0xb8, 0x1e },
{ 0xbf, 0xb4, 0x41, 0x27 },
{ 0x5d, 0x52, 0x11, 0x98 },
{ 0x30, 0xae, 0xf1, 0xe5 }
};
//string text = "abcd";
//byte[] input = Str2ByteArray(text);
state = SubBytes(input);
}
}
}
我已经创建了SubBytes函数,它可以正常工作。 我知道状态的类型必须与输入的类型相同。
error. 最后一行给出了错误。 我为什么要这个? 我究竟做错了什么?
SubBytes
声明为实例方法,而不是静态方法。 这是一个问题,因为您是从静态上下文 - Main
方法调用它。
将方法的定义更改为此,您应该没问题:
public static byte[,] SubBytes(byte[,] input)
您的方法是实例方法。
它们需要一个Program
类的实例才能工作。
但是,你根本不应该这样做。
使用System.Security.Cryptography.RijndaelManged
; 不要重新发明轮子(特别是在加密中)。
你必须使SubBites静态
这是因为您的Main方法是静态的。
您需要声明一个新的Program实例来访问这样的方法:
state = new Program().SubBytes(input);
或者将方法声明为静态(这很好,因为它们不访问任何成员数据)。
尝试将SubBytes方法设为静态:
public static byte[,] SubBytes(byte[,] input)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.