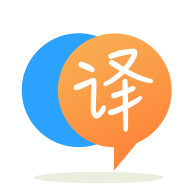
[英]beautifulsoup - how to find tags starting with certain attribute?
[英]How to find tags with only certain attributes - BeautifulSoup
我将如何使用 BeautifulSoup 搜索仅包含我搜索的属性的标签?
例如,我想查找所有<td valign="top">
标签。
以下代码: raw_card_data = soup.fetch('td', {'valign':re.compile('top')})
获取我想要的所有数据,但也获取任何具有属性valign:top
的<td>
标记
我也尝试过: raw_card_data = soup.findAll(re.compile('<td valign="top">'))
并且没有返回任何内容(可能是因为正则表达式错误)
我想知道 BeautifulSoup 中是否有办法说“查找唯一属性为valign:top
的<td>
标签”
更新例如,如果 HTML 文档包含以下<td>
标记:
<td valign="top">.....</td><br />
<td width="580" valign="top">.......</td><br />
<td>.....</td><br />
我只想返回第一个<td>
标记( <td width="580" valign="top">
)
你可以使用这个:
soup = BeautifulSoup(html)
results = soup.findAll("td", {"valign" : "top"})
编辑 :
要返回只有 valign="top" 属性的标签,您可以检查标签attrs
属性的长度:
from BeautifulSoup import BeautifulSoup
html = '<td valign="top">.....</td>\
<td width="580" valign="top">.......</td>\
<td>.....</td>'
soup = BeautifulSoup(html)
results = soup.findAll("td", {"valign" : "top"})
for result in results :
if len(result.attrs) == 1 :
print result
那返回:
<td valign="top">.....</td>
您可以按照文档中的说明在findAll
使用lambda
函数。 因此,在您的情况下,仅使用valign = "top"
搜索td
标签,请使用以下内容:
td_tag_list = soup.findAll(
lambda tag:tag.name == "td" and
len(tag.attrs) == 1 and
tag["valign"] == "top")
如果您只想使用具有任何值的属性名称进行搜索
from bs4 import BeautifulSoup
import re
soup= BeautifulSoup(html.text,'lxml')
results = soup.findAll("td", {"valign" : re.compile(r".*")})
根据 Steve Lorimer 的说法,最好通过 True 而不是正则表达式
results = soup.findAll("td", {"valign" : True})
最简单的方法是使用新的 CSS 样式select
方法:
soup = BeautifulSoup(html)
results = soup.select('td[valign="top"]')
只需将其作为findAll
的参数传递即可:
>>> from BeautifulSoup import BeautifulSoup
>>> soup = BeautifulSoup("""
... <html>
... <head><title>My Title!</title></head>
... <body><table>
... <tr><td>First!</td>
... <td valign="top">Second!</td></tr>
... </table></body><html>
... """)
>>>
>>> soup.findAll('td')
[<td>First!</td>, <td valign="top">Second!</td>]
>>>
>>> soup.findAll('td', valign='top')
[<td valign="top">Second!</td>]
添加 Chris Redford 和 Amr 的答案的组合,您还可以使用 select 命令搜索具有任何值的属性名称:
from bs4 import BeautifulSoup as Soup
html = '<td valign="top">.....</td>\
<td width="580" valign="top">.......</td>\
<td>.....</td>'
soup = Soup(html, 'lxml')
results = soup.select('td[valign]')
在任何标签中使用属性查找
<th class="team" data-sort="team">Team</th>
soup.find_all(attrs={"class": "team"})
<th data-sort="team">Team</th>
soup.find_all(attrs={"data-sort": "team"})
如果您希望提取存在特定属性的所有标签,您可以使用与接受的答案相同的代码,但不要为标签指定值,只需输入 True。
soup = BeautifulSoup(html)
results = soup.findAll("td", {"valign" : True})
这将返回所有具有 valign 属性的 td 标签。 如果您的项目涉及从 div 之类的标签中提取信息,这将非常有用,但它可以处理您可能正在寻找的非常特定的属性。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.