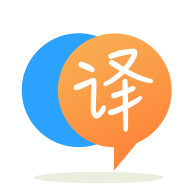
[英]UITableviewcell delete button apprearing when I swipe right to left on a cell
[英]Swipe left or right anywhere in a UITableViewCell to delete the cell with no delete button?
我希望能够在表格视图单元格中的任何位置向左或向右滑动以删除带动画的单元格而不显示删除按钮。 我怎样才能做到这一点?
我没有试过并实现过这个,但我会试一试。 首先,创建一个自定义UITableViewCell,并让它有2个属性可以使用
在您的cellForRowAtIndexPath:
,在创建自定义单元格的位置,设置这些属性。 还要向单元格添加UISwipeGestureRecognizer
cell.tableView=tableView;
cell.indexPath=indexPath;
UISwipeGestureRecognizer *swipeGestureRecognizer=[[UISwipeGestureRecognizer alloc] initWithTarget:self action:@selector(deleteCell:)];
[cell addGestureRecognizer:swipeGestureRecognizer];
[swipeGestureRecognizer release];
确保手势仅接收水平滑动。
-(BOOL) gestureRecognizer:(UIGestureRecognizer *)gestureRecognizer shouldReceiveTouch:(UITouch *)touch
{
if([[gestureRecognizer view] isKindOfClass:[UITableViewCell class]]&&
((UISwipeGestureRecognizer*)gestureRecognizer.direction==UISwipeGestureRecognizerDirectionLeft
||(UISwipeGestureRecognizer*)gestureRecognizer.direction==UISwipeGestureRecognizerDirectionRight)) return YES;
}
在你的deleteCell:
-(void) deleteCell:(UIGestureRecognizer*)gestureRec
{
UIGestureRecognizer *swipeGestureRecognizer=(UISwipeGestureRecognizer*)gestureRec;
CustomCell *cell=[swipeGestureRecognizer view];
UITableView *tableView=cell.tableView;
NSIndexPath *indexPath=cell.indexPath;
//you can now use these two to perform delete operation
}
@MadhavanRP发布的解决方案有效,但它比它需要的更复杂。 您可以采用更简单的方法并创建一个手势识别器来处理表中发生的所有滑动,然后获取滑动的位置以确定用户刷过的单元格。
要设置手势识别器:
- (void)setUpLeftSwipe {
UISwipeGestureRecognizer *recognizer;
recognizer = [[UISwipeGestureRecognizer alloc] initWithTarget:self
action:@selector(swipeLeft:)];
[recognizer setDirection:UISwipeGestureRecognizerDirectionLeft];
[self.tableView addGestureRecognizer:recognizer];
recognizer.delegate = self;
}
在viewDidLoad
调用此方法
要处理滑动:
- (void)swipeLeft:(UISwipeGestureRecognizer *)gestureRecognizer {
CGPoint location = [gestureRecognizer locationInView:self.tableView];
NSIndexPath *indexPath = [self.tableView indexPathForRowAtPoint:location];
... do something with cell now that i have the indexpath, maybe save the world? ...
}
注意:您的vc必须实现手势识别器委托。
实行
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath;
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath;
这两个方法,如果UITableViewCellEditingStyle是UITableViewCellEditingStyleDelete,那么做某事来擦除你的单元格
- (void)deleteRowsAtIndexPaths:(NSArray *)indexPaths withRowAnimation:(UITableViewRowAnimation)animation;
就这样。
为此,你必须在你的项目中添加此代码。
// Override to support conditional editing of the table view.
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath {
// Return YES if you want the specified item to be editable.
return YES;
}
// Override to support editing the table view.
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath {
if (editingStyle == UITableViewCellEditingStyleDelete) {
//add code here for when you hit delete
}
}
其他信息你可以通过这个链接在这里输入链接描述
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.