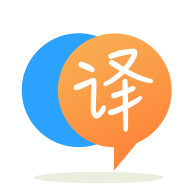
[英]How do I call a non-static method from another class in a non-static method? (java)
[英]Java: How do i create objects in a static method and also call for methods from another class?
我正在做这个Java任务几个小时,并坚持使用这个测试器类近5个小时。
在这个作业中,我创建了一个Product类,一个Money类,一个LineItem类和一个Inventory类。 现在我需要创建一个测试类来测试程序,方法是将新的lineitems放入库存数组中。
在测试器类中,我试图创建一个静态方法public static void addTestItems(Inventory theInventory)
,它假设添加4个项目。 对于每个项目,我将需要创建一个产品对象,后跟一个LineItem对象以包含新创建的产品。 接下来,我需要使用库存类中的方法将项目添加到库存类中的数组中。
我到目前为止所尝试的内容:
private static void addTestItems(Inventory theInventory)
{
Inventory[] _items;
Product product1 = new Product("Book","Objects first with Java"," An excellent introductory Java textbook");
Product product2 = new Product("CD","The dark side of the moon","The all-time classic Pink Floyd album");
Product product3 = new Product("DVD", "Transformers","Robots in disguise");
Product product4 = new Product("Laptop","Lenovo T42","A good yet affordabble laptop");
Money unitPrice1 = new Money(29,99);
Money unitPrice2 = new Money(4,99);
Money unitPrice3 = new Money(9,99);
Money unitPrice4 = new Money(450,0);
_items[0] = new LineItem(product1,5,unitPrice1);
_items[1] = new LineItem(product2,8,unitPrice2);
_items[2] = new LineItem(product3,200,unitPrice3);
_items[3] = new LineItem(product4,9,unitPrice4);
}
当前错误是incompatible types- found LineItem but expected Inventory
因此我尝试更改Inventory[] _items;
to LineItem[] _items;
。 但错误是变量_items可能不是初始化。
对不起伙计我是Java中的真正菜鸟,我尝试在线搜索多年,但我不太了解大多数结果。 我理解的唯一一个是http://forums.devshed.com/java-help-9/bluej-compiler-error-cannot-find-symbol-variable-object-688573.html但我厌倦了进入我的背景但是失败了。 我也发现了很多结果但是它们中有构造函数和实例变量,我老师特别提到我不需要它们。
不知道专家能指导我,就像让我知道自己的错误一样。 谢谢,谢谢。
库存类:
/**
* In the Inventory class, it is merely to create a list / array of product which allows the information from the linitem to be put with an index.
* For example, for the first product, we can use the inventory class to input it into the index 1. and he next product into index 2 and so on.
* It is suse to create an array and inputing the lineitem information into it.
*
* @author (your name)
* @version (a version number or a date)
*/
public class Inventory
{
// instance variables - replace the example below with your own
private LineItem[] _items;
private int _numItems;
/**
* Constructor for objects of class Inventory
*/
public Inventory()
{
// initialise instance variables
_items = new LineItem[1000];
_numItems = 0;
}
/**
* An example of a method - replace this comment with your own
*
* @param y a sample parameter for a method
* @return the sum of x and y
*/
public void addItem(LineItem item)
{
_items[_numItems]= item;
_numItems++;
}
public String toString()
{
String result="";
int i=0;
while (i < _numItems)
{
result = result + _items[i] + "/n";
i++;
}
return result;
}
public void print()
{
String myResult=this.toString();
System.out.println(myResult);
}
public Money getTotalValue()
{
int i=0;
Money total= new Money(0);
while (i<_items.length)
{
total = total.add(Money.NO_MONEY);
i++;
}
return total;
}
public LineItem getItem(String productName)
{
int i = 0;
LineItem itemDetails = null;
while (i<_items.length)
{
if (_items[i].equals(productName))
{
itemDetails= _items[i];
}
else
{
//do nothing
}
i++;
}
return itemDetails;
}
}
我还没有对这些方法发表评论,但一旦理解了它就会这样做。
您的数组是Inventory[]
类型 - 但您尝试分配LineItem
类型的引用。 你也没有初始化它。 改变这个:
Inventory[] _items;
对此:
LineItem[] _items = new LineItem[5];
一切都应该很好 - 虽然你没有使用索引0(这就是为什么你需要它的大小为5)并且你之后没有对数组做任何事情......
使用数组的另一种方法是使用List:
List<LineItem> items = new ArrayList<LineItem>();
items.add(new LineItem(product1, 5, unitPrice1));
items.add(new LineItem(product2, 8, unitPrice2));
items.add(new LineItem(product3, 200, unitPrice3));
items.add(new LineItem(product4, 9, unitPrice4));
...接下来想想你对items
变量实际想做些什么。
LineItem[] _items = new LineItem[4];
那么索引从0开始而不是从1开始,
_items[4]
将返回indexoutofbounds错误
一些东西:
incompatible types- found LineItem but expected Inventory
是由于您的数组应该包含Inventory对象,而您正在为其分配LineItems
variable _items may not be initialise
表示您拥有_items对象,但尚未将其初始化为任何对象。 你想做
LineItem[] _items = new LineItem[4];
PS:如果你想要动态大小的数组,不知道你可能会加载多少行项目等等,使用向量或集合或沿着这些行的东西。
也,
_items[1] = new LineItem(product1,5,unitPrice1);
_items[2] = new LineItem(product2,8,unitPrice2);
_items[3] = new LineItem(product3,200,unitPrice3);
_items[4] = new LineItem(product4,9,unitPrice4);
在Java中,数组元素以索引0开头,而不是1
_items
是一个多变的名称,让你的队友在你的咖啡中打喷嚏
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.