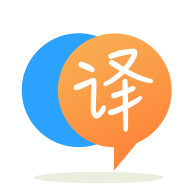
[英]How do I call a non-static method from another class in a non-static method? (java)
[英]Java: How do i create objects in a static method and also call for methods from another class?
我正在做這個Java任務幾個小時,並堅持使用這個測試器類近5個小時。
在這個作業中,我創建了一個Product類,一個Money類,一個LineItem類和一個Inventory類。 現在我需要創建一個測試類來測試程序,方法是將新的lineitems放入庫存數組中。
在測試器類中,我試圖創建一個靜態方法public static void addTestItems(Inventory theInventory)
,它假設添加4個項目。 對於每個項目,我將需要創建一個產品對象,后跟一個LineItem對象以包含新創建的產品。 接下來,我需要使用庫存類中的方法將項目添加到庫存類中的數組中。
我到目前為止所嘗試的內容:
private static void addTestItems(Inventory theInventory)
{
Inventory[] _items;
Product product1 = new Product("Book","Objects first with Java"," An excellent introductory Java textbook");
Product product2 = new Product("CD","The dark side of the moon","The all-time classic Pink Floyd album");
Product product3 = new Product("DVD", "Transformers","Robots in disguise");
Product product4 = new Product("Laptop","Lenovo T42","A good yet affordabble laptop");
Money unitPrice1 = new Money(29,99);
Money unitPrice2 = new Money(4,99);
Money unitPrice3 = new Money(9,99);
Money unitPrice4 = new Money(450,0);
_items[0] = new LineItem(product1,5,unitPrice1);
_items[1] = new LineItem(product2,8,unitPrice2);
_items[2] = new LineItem(product3,200,unitPrice3);
_items[3] = new LineItem(product4,9,unitPrice4);
}
當前錯誤是incompatible types- found LineItem but expected Inventory
因此我嘗試更改Inventory[] _items;
to LineItem[] _items;
。 但錯誤是變量_items可能不是初始化。
對不起伙計我是Java中的真正菜鳥,我嘗試在線搜索多年,但我不太了解大多數結果。 我理解的唯一一個是http://forums.devshed.com/java-help-9/bluej-compiler-error-cannot-find-symbol-variable-object-688573.html但我厭倦了進入我的背景但是失敗了。 我也發現了很多結果但是它們中有構造函數和實例變量,我老師特別提到我不需要它們。
不知道專家能指導我,就像讓我知道自己的錯誤一樣。 謝謝,謝謝。
庫存類:
/**
* In the Inventory class, it is merely to create a list / array of product which allows the information from the linitem to be put with an index.
* For example, for the first product, we can use the inventory class to input it into the index 1. and he next product into index 2 and so on.
* It is suse to create an array and inputing the lineitem information into it.
*
* @author (your name)
* @version (a version number or a date)
*/
public class Inventory
{
// instance variables - replace the example below with your own
private LineItem[] _items;
private int _numItems;
/**
* Constructor for objects of class Inventory
*/
public Inventory()
{
// initialise instance variables
_items = new LineItem[1000];
_numItems = 0;
}
/**
* An example of a method - replace this comment with your own
*
* @param y a sample parameter for a method
* @return the sum of x and y
*/
public void addItem(LineItem item)
{
_items[_numItems]= item;
_numItems++;
}
public String toString()
{
String result="";
int i=0;
while (i < _numItems)
{
result = result + _items[i] + "/n";
i++;
}
return result;
}
public void print()
{
String myResult=this.toString();
System.out.println(myResult);
}
public Money getTotalValue()
{
int i=0;
Money total= new Money(0);
while (i<_items.length)
{
total = total.add(Money.NO_MONEY);
i++;
}
return total;
}
public LineItem getItem(String productName)
{
int i = 0;
LineItem itemDetails = null;
while (i<_items.length)
{
if (_items[i].equals(productName))
{
itemDetails= _items[i];
}
else
{
//do nothing
}
i++;
}
return itemDetails;
}
}
我還沒有對這些方法發表評論,但一旦理解了它就會這樣做。
您的數組是Inventory[]
類型 - 但您嘗試分配LineItem
類型的引用。 你也沒有初始化它。 改變這個:
Inventory[] _items;
對此:
LineItem[] _items = new LineItem[5];
一切都應該很好 - 雖然你沒有使用索引0(這就是為什么你需要它的大小為5)並且你之后沒有對數組做任何事情......
使用數組的另一種方法是使用List:
List<LineItem> items = new ArrayList<LineItem>();
items.add(new LineItem(product1, 5, unitPrice1));
items.add(new LineItem(product2, 8, unitPrice2));
items.add(new LineItem(product3, 200, unitPrice3));
items.add(new LineItem(product4, 9, unitPrice4));
...接下來想想你對items
變量實際想做些什么。
LineItem[] _items = new LineItem[4];
那么索引從0開始而不是從1開始,
_items[4]
將返回indexoutofbounds錯誤
一些東西:
incompatible types- found LineItem but expected Inventory
是由於您的數組應該包含Inventory對象,而您正在為其分配LineItems
variable _items may not be initialise
表示您擁有_items對象,但尚未將其初始化為任何對象。 你想做
LineItem[] _items = new LineItem[4];
PS:如果你想要動態大小的數組,不知道你可能會加載多少行項目等等,使用向量或集合或沿着這些行的東西。
也,
_items[1] = new LineItem(product1,5,unitPrice1);
_items[2] = new LineItem(product2,8,unitPrice2);
_items[3] = new LineItem(product3,200,unitPrice3);
_items[4] = new LineItem(product4,9,unitPrice4);
在Java中,數組元素以索引0開頭,而不是1
_items
是一個多變的名稱,讓你的隊友在你的咖啡中打噴嚏
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.