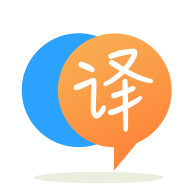
[英]how to download a perticular file using it's name from amazon s3 bucket using java
[英]Key for download bucket in Amazon S3 using Java
public class downloads3 {
private static String bucketName = "s3-upload-sdk-sample-akiaj6ufcgzvw7yukypa";
**private static String key = "__________________________________";**
public static void main(String[] args) throws IOException {
AmazonS3 s3Client = new AmazonS3Client(new PropertiesCredentials(
downloads3.class.getResourceAsStream(
"AwsCredentials.properties")));
try {
System.out.println("Downloading an object");
S3Object s3object = s3Client.getObject(new GetObjectRequest(
bucketName, key));
System.out.println("Content-Type: " +
s3object.getObjectMetadata().getContentType());
displayTextInputStream(s3object.getObjectContent());
// Get a range of bytes from an object.
GetObjectRequest rangeObjectRequest = new GetObjectRequest(
bucketName, key);
rangeObjectRequest.setRange(0, 10);
S3Object objectPortion = s3Client.getObject(rangeObjectRequest);
System.out.println("Printing bytes retrieved.");
displayTextInputStream(objectPortion.getObjectContent());
} catch (AmazonServiceException ase) {
System.out.println("Caught an AmazonServiceException, which" +
" means your request made it " +
"to Amazon S3, but was rejected with an error response" +
" for some reason.");
System.out.println("Error Message: " + ase.getMessage());
System.out.println("HTTP Status Code: " + ase.getStatusCode());
System.out.println("AWS Error Code: " + ase.getErrorCode());
System.out.println("Error Type: " + ase.getErrorType());
System.out.println("Request ID: " + ase.getRequestId());
} catch (AmazonClientException ace) {
System.out.println("Caught an AmazonClientException, which means"+
" the client encountered " +
"an internal error while trying to " +
"communicate with S3, " +
"such as not being able to access the network.");
System.out.println("Error Message: " + ace.getMessage());
}
}
private static void displayTextInputStream(InputStream input)
throws IOException {
// Read one text line at a time and display.
BufferedReader reader = new BufferedReader(new
InputStreamReader(input));
while (true) {
String line = reader.readLine();
if (line == null) break;
System.out.println(" " + line);
}
System.out.println();
}
}
我正在尝试使用Java从Amazon S3存储桶下载对象。 但它似乎不起作用,并一直给我下面显示的错误。 输入的正确键是什么? 访问密钥或密钥?
Downloading an object
Caught an AmazonServiceException, which means your request made it to Amazon S3, but was rejected with an error response for some reason.
Error Message: The specified key does not exist.
HTTP Status Code: 404
AWS Error Code: NoSuchKey
Error Type: Client
Request ID: F9548FC068DB1646
确保您在代码中使用的密钥与存储桶中的密钥一致。 请记住,Amazon S3密钥不区分大小写,这意味着您的密钥名称可能有一些不同的情况,如大写或小写。
检查并重试。
正如其他人指出的那样, 密钥是存储在S3上的对象(文件/文件夹等)的唯一标识符 。
有关命名和概念设计的更多信息,请参见http://docs.aws.amazon.com/AmazonS3/latest/dev/Introduction.html#CoreConcepts 。
注意:如果您尝试上传文件然后立即执行getObjectRequest,有时(大多数情况下)会出现延迟并导致404 NoSuchKey,因此您需要在设计中考虑该场景。
“密钥” 不是用于请求的凭据。 如果是这种情况,你会得到401 Unauthorized 。 404表示设置了适当的认证凭证。
密钥可以被视为文件名(或者就此而言的文件名)。 这可以是任意值,但大多数人更喜欢使用正斜杠模拟文件系统结构(甚至亚马逊S3控制台都这样做,并且创建文件夹按钮等)
如果您可以访问控制台,请浏览您的存储桶并确保存在所有密钥前缀或“父目录”,包括存储桶或“根文件夹”。
Amazon S3存储桶中的“密钥”是您要下载的文件名。
在你的情况下:
private static String key = "__________________________________"; //will be file name/ file path to that file in Amazon Bucket.
我有一个类似的问题。 我的访问密钥,密钥,存储桶,端点和路径都是正确的。
事实证明,对我来说问题是我在S3中指向的文件不存在因此404错误。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.