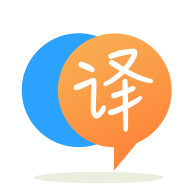
[英]how to download a perticular file using it's name from amazon s3 bucket using java
[英]Key for download bucket in Amazon S3 using Java
public class downloads3 {
private static String bucketName = "s3-upload-sdk-sample-akiaj6ufcgzvw7yukypa";
**private static String key = "__________________________________";**
public static void main(String[] args) throws IOException {
AmazonS3 s3Client = new AmazonS3Client(new PropertiesCredentials(
downloads3.class.getResourceAsStream(
"AwsCredentials.properties")));
try {
System.out.println("Downloading an object");
S3Object s3object = s3Client.getObject(new GetObjectRequest(
bucketName, key));
System.out.println("Content-Type: " +
s3object.getObjectMetadata().getContentType());
displayTextInputStream(s3object.getObjectContent());
// Get a range of bytes from an object.
GetObjectRequest rangeObjectRequest = new GetObjectRequest(
bucketName, key);
rangeObjectRequest.setRange(0, 10);
S3Object objectPortion = s3Client.getObject(rangeObjectRequest);
System.out.println("Printing bytes retrieved.");
displayTextInputStream(objectPortion.getObjectContent());
} catch (AmazonServiceException ase) {
System.out.println("Caught an AmazonServiceException, which" +
" means your request made it " +
"to Amazon S3, but was rejected with an error response" +
" for some reason.");
System.out.println("Error Message: " + ase.getMessage());
System.out.println("HTTP Status Code: " + ase.getStatusCode());
System.out.println("AWS Error Code: " + ase.getErrorCode());
System.out.println("Error Type: " + ase.getErrorType());
System.out.println("Request ID: " + ase.getRequestId());
} catch (AmazonClientException ace) {
System.out.println("Caught an AmazonClientException, which means"+
" the client encountered " +
"an internal error while trying to " +
"communicate with S3, " +
"such as not being able to access the network.");
System.out.println("Error Message: " + ace.getMessage());
}
}
private static void displayTextInputStream(InputStream input)
throws IOException {
// Read one text line at a time and display.
BufferedReader reader = new BufferedReader(new
InputStreamReader(input));
while (true) {
String line = reader.readLine();
if (line == null) break;
System.out.println(" " + line);
}
System.out.println();
}
}
我正在嘗試使用Java從Amazon S3存儲桶下載對象。 但它似乎不起作用,並一直給我下面顯示的錯誤。 輸入的正確鍵是什么? 訪問密鑰或密鑰?
Downloading an object
Caught an AmazonServiceException, which means your request made it to Amazon S3, but was rejected with an error response for some reason.
Error Message: The specified key does not exist.
HTTP Status Code: 404
AWS Error Code: NoSuchKey
Error Type: Client
Request ID: F9548FC068DB1646
確保您在代碼中使用的密鑰與存儲桶中的密鑰一致。 請記住,Amazon S3密鑰不區分大小寫,這意味着您的密鑰名稱可能有一些不同的情況,如大寫或小寫。
檢查並重試。
正如其他人指出的那樣, 密鑰是存儲在S3上的對象(文件/文件夾等)的唯一標識符 。
有關命名和概念設計的更多信息,請參見http://docs.aws.amazon.com/AmazonS3/latest/dev/Introduction.html#CoreConcepts 。
注意:如果您嘗試上傳文件然后立即執行getObjectRequest,有時(大多數情況下)會出現延遲並導致404 NoSuchKey,因此您需要在設計中考慮該場景。
“密鑰” 不是用於請求的憑據。 如果是這種情況,你會得到401 Unauthorized 。 404表示設置了適當的認證憑證。
密鑰可以被視為文件名(或者就此而言的文件名)。 這可以是任意值,但大多數人更喜歡使用正斜杠模擬文件系統結構(甚至亞馬遜S3控制台都這樣做,並且創建文件夾按鈕等)
如果您可以訪問控制台,請瀏覽您的存儲桶並確保存在所有密鑰前綴或“父目錄”,包括存儲桶或“根文件夾”。
Amazon S3存儲桶中的“密鑰”是您要下載的文件名。
在你的情況下:
private static String key = "__________________________________"; //will be file name/ file path to that file in Amazon Bucket.
我有一個類似的問題。 我的訪問密鑰,密鑰,存儲桶,端點和路徑都是正確的。
事實證明,對我來說問題是我在S3中指向的文件不存在因此404錯誤。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.