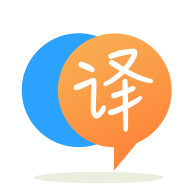
[英]How to connect a mobile application from your android mobile device to your laptop server using sockets?
[英]How to connect an Android application with IIS server on laptop
我已经开发了一个使用C#网络服务的android应用程序。 我已经使用IIS服务器发布了Web服务。 现在,我想将应用程序安装到Android设备上,并将其连接到IIS服务器上运行的WCF Rest服务。
我应该采取什么步骤来完成此任务?
到目前为止,我已经使用了下面提到的方法。 但是有一些错误:(请帮助我修复它们!
public String ServiceCallMethod()
{
//int x1 = 262, x2 = 525, y1 = 100, y2 = 390;
String url = "http://192.168.43.73:85/PathService/PathService.svc/FindPath/"+x1+","+y1+"/"+x2+","+y2+"";
HttpClient httpclient = new DefaultHttpClient();
// Prepare a request object
HttpGet httpget = new HttpGet(url);
// Execute the request
HttpResponse response;
try {
response = httpclient.execute(httpget);
// Examine the response status
Log.i("Praeda",response.getStatusLine().toString());
// Get hold of the response entity
HttpEntity entity = response.getEntity();
// If the response does not enclose an entity, there is no need
// to worry about connection release
if (entity != null) {
// A Simple JSON Response Read
InputStream instream = entity.getContent();
String result= convertStreamToString(instream); // returns error when i debug :(its supposed to return an point array list like this..
//Response - {"FindPathResult":[{"x":262,"y":165},{"x":346,"y":165},{"x":420,"y":165},{"x":473,"y":165},{"x":473,"y":240},{"x":473,"y":277},{"x":473,"y":320},{"x":473,"y":390},{"x":525,"y":390}]}
//Instead it returns this
//<?xml version="1.0" encoding="utf-8"?>
// <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 //Transitional//EN""http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
// <html xmlns="http://www.w3.org/1999/xhtml">
// <head>
// <title>Request Error</title>
// <style>BODY { color: #000000; background-color: white; font-family: Verdana; margin-left: 0px; margin-top: 0px; } #content { margin-left: 30px; font-size: .70em; padding-bottom: 2em; } A:link { color: #336699; font-weight: bold; text-decoration: underline; } A:visited { color: #6699cc; font-weight: bold; text-decoration: underline; } A:active { color: #336699; font-weight: bold; text-decoration: underline; } .heading1 { background-color: #003366; border-bottom: #336699 6px solid; color: #ffffff; font-family: Tahoma; font-size: 26px; font-weight: normal;margin: 0em 0em 10px -20px; padding-bottom: 8px; padding-left: 30px;padding-top: 16px;} pre { font-size:small; background-color: #e5e5cc; padding: 5px; font-family: Courier New; margin-top: 0px; border: 1px #f0f0e0 solid; white-space: pre-wrap; white-space: -pre-wrap; word-wrap: break-word; } table { border-collapse: collapse; border-spacing: 0px; font-family: Verdana;} table th { border-right: 2px white solid; border-bottom: 2px white solid; font-weight: bold; background-color: #cecf9c;} table td { border-right: 2px white solid; border-bottom: 2px white solid; background-color: #e5e5cc;}</style>
//</head>
//<body>
//<div id="content">
//<p class="heading1">Request Error</p>
//<p>The server encountered an error processing the request. See server logs for more details.</p>
//</div>
//</body>
//</html>
// ----------------------------------------------------------------------------
Log.i("Praeda",result);
// A Simple JSONObject Creation
JSONObject json=new JSONObject(result);
Log.i("Praeda","<jsonobject>\n"+json.toString()+"\n</jsonobject>");
// A Simple JSONObject Parsing
JSONArray nameArray=json.names();
JSONArray valArray=json.toJSONArray(nameArray);
for(int i=0;i<valArray.length();i++)
{
Log.i("Praeda","<jsonname"+i+">\n"+nameArray.getString(i)+"\n</jsonname"+i+">\n"
+"<jsonvalue"+i+">\n"+valArray.getString(i)+"\n</jsonvalue"+i+">");
Log.i("TEST","JSONArray Recieved");
}
// A Simple JSONObject Value Pushing
json.put("sample key", "sample value");
Log.i("Praeda","<jsonobject>\n"+json.toString()+"\n</jsonobject>");
// Closing the input stream will trigger connection release
instream.close();
//I want to assign the result to one of my String arrays(this_results) -------------
this_result = result;
//-----------------------------------------------------
System.out.println(" End of ServiceCallMethod() ");
return result;
}
} catch (ClientProtocolException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
private static String convertStreamToString(InputStream is) {
/*
* To convert the InputStream to String we use the BufferedReader.readLine()
* method. We iterate until the BufferedReader return null which means
* there's no more data to read. Each line will appended to a StringBuilder
* and returned as String.
*/
BufferedReader reader = new BufferedReader(new InputStreamReader(is));
StringBuilder sb = new StringBuilder();
String line = null;
try {
while ((line = reader.readLine()) != null) {
sb.append(line + "\n");
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return sb.toString();
}
这是我的WCF Rest Get方法
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.Text;
using testServer;
namespace IGTSMPathService
{
// NOTE: You can use the "Rename" command on the "Refactor" menu to change the class name "PathService" in code, svc and config file together.
public class PathService : IPathService
{
//GET Method
//http://localhost:50837/PathService.svc/FindPath/262,100/525,390
//Response - {"FindPathResult":[{"x":262,"y":165},{"x":346,"y":165},{"x":420,"y":165}, {"x":473,"y":165},{"x":473,"y":240},{"x":473,"y":277},{"x":473,"y":320},{"x":473,"y":390},{"x":525,"y":390}]}
public System.Drawing.Point[] FindPath(string cLocation, string destination)
{
System.Drawing.Point[] list;
Location loc = new Location(cLocation);
System.Drawing.Point cur, des;
cur = new System.Drawing.Point(Int32.Parse(cLocation.Split(',')[0].Trim()), Int32.Parse(cLocation.Split(',')[1].Trim()));
des = new System.Drawing.Point(Int32.Parse(destination.Split(',')[0].Trim()), Int32.Parse(destination.Split(',')[1].Trim()));
list = loc.findPoint(cur, des);
return list;
}
}
}
将包装器用于您的服务方法,例如:对于POST:
[OperationContract]
[WebInvoke(BodyStyle = WebMessageBodyStyle.WrappedRequest,
ResponseFormat = WebMessageFormat.Json,
Method = "POST",
UriTemplate = "login")]
对于GET:
[OperationContract]
[WebInvoke(BodyStyle = WebMessageBodyStyle.Bare,
ResponseFormat = WebMessageFormat.Json,
Method = "GET",
UriTemplate = "users/{userId}")]
在您的android应用程序中,使用任何与Web服务一起工作的示例,但使用IIS服务器的ip(请确保wi-fi可从设备使用IIS服务器)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.