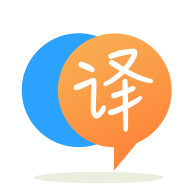
[英]How to set folder write permissions for application add-on with python
[英]How to set folder permissions in Windows?
创建用户 AD 帐户时,我正在使用 Python 创建一个新的个人文件夹。 正在创建文件夹,但权限不正确。 Python 可以将用户添加到新创建的文件夹并更改其权限吗? 我不确定从哪里开始编码。
您需要win32security
模块,它是pywin32的一部分。 这是一个做你想做的事情的例子。
该示例为文件创建了一个新的 DACL 并替换了旧的 DACL,但修改现有的很容易; 您需要做的就是从安全描述符中获取现有的 DACL,而不是创建一个空的 DACL,如下所示:
import win32security
import ntsecuritycon as con
FILENAME = "whatever"
userx, domain, type = win32security.LookupAccountName ("", "User X")
usery, domain, type = win32security.LookupAccountName ("", "User Y")
sd = win32security.GetFileSecurity(FILENAME, win32security.DACL_SECURITY_INFORMATION)
dacl = sd.GetSecurityDescriptorDacl() # instead of dacl = win32security.ACL()
dacl.AddAccessAllowedAce(win32security.ACL_REVISION, con.FILE_GENERIC_READ | con.FILE_GENERIC_WRITE, userx)
dacl.AddAccessAllowedAce(win32security.ACL_REVISION, con.FILE_ALL_ACCESS, usery)
sd.SetSecurityDescriptorDacl(1, dacl, 0) # may not be necessary
win32security.SetFileSecurity(FILENAME, win32security.DACL_SECURITY_INFORMATION, sd)
这是kindall 的答案的一个版本,它使用带有SetEntriesInAcl
EXPLICIT_ACCESS
条目,它以规范的顺序使用 ACE 创建一个正确的 ACL(例如,首先列出拒绝访问的 ACE)。 此外,此版本使用SetNamedSecurityInfo
设置 DACL,它支持传播可继承的 ACE,与过时的函数SetFileSecurity
。
import ntsecuritycon
import win32security
FILENAME = "whatever"
USERX = "UserX"
USERY = "UserY"
entries = [{'AccessMode': win32security.GRANT_ACCESS,
'AccessPermissions': 0,
'Inheritance': win32security.CONTAINER_INHERIT_ACE |
win32security.OBJECT_INHERIT_ACE,
'Trustee': {'TrusteeType': win32security.TRUSTEE_IS_USER,
'TrusteeForm': win32security.TRUSTEE_IS_NAME,
'Identifier': ''}}
for i in range(2)]
entries[0]['AccessPermissions'] = (ntsecuritycon.GENERIC_READ |
ntsecuritycon.GENERIC_WRITE)
entries[0]['Trustee']['Identifier'] = USERX
entries[1]['AccessPermissions'] = ntsecuritycon.GENERIC_ALL
entries[1]['Trustee']['Identifier'] = USERY
sd = win32security.GetNamedSecurityInfo(FILENAME, win32security.SE_FILE_OBJECT,
win32security.DACL_SECURITY_INFORMATION)
dacl = sd.GetSecurityDescriptorDacl()
dacl.SetEntriesInAcl(entries)
win32security.SetNamedSecurityInfo(FILENAME, win32security.SE_FILE_OBJECT,
win32security.DACL_SECURITY_INFORMATION |
win32security.UNPROTECTED_DACL_SECURITY_INFORMATION,
None, None, dacl, None)
对于那些对 ACE 的安全描述符“列表”感兴趣的人,可以使用以下数据结构。 我在这方面得到了一些帮助,从那以后就一直在使用它。
typical_aces={
2032127L:"Full Control(All)",
1179817L:"Read(RX)",
1180086L:"Add",
1180095L:"Add&Read",
1245631L:"Change"
}
binary_aces={
1:"ACCESS_READ", #0x00000001
2:"ACCESS_WRITE", #0x00000002
4:"ACCESS_CREATE", #0x00000004
8:"ACCESS_EXEC", #0x00000008
16:"ACCESS_DELETE", #0x00000010
32:"ACCESS_ATRIB", #0x00000020
64:"ACCESS_PERM", #0x00000040
32768:"ACCESS_GROUP", #0x00008000
65536:"DELETE", #0x00010000
131072:"READ_CONTROL", #0x00020000
262144:"WRITE_DAC", #0x00040000
524288:"WRITE_OWNER", #0x00080000
1048576:"SYNCHRONIZE", #0x00100000
16777216:"ACCESS_SYSTEM_SECURITY",#0x01000000
33554432:"MAXIMUM_ALLOWED", #0x02000000
268435456:"GENERIC_ALL", #0x10000000
536870912:"GENERIC_EXECUTE",#0x20000000
1073741824:"GENERIC_WRITE", #0x40000000
65535:"SPECIFIC_RIGHTS_ALL",#0x0000ffff
983040:"STANDARD_RIGHTS_REQUIRED",#0x000f0000
2031616:"STANDARD_RIGHTS_ALL",#0x001f0000
}
将掩码从给定的 DACL / 路径传递到:
def calculate_plaintext_mask(mask):
a=2147483648L
if typical_aces.has_key(mask):
return typical_aces[mask]
else:
result='NONE'
while a>>1:
a=a>>1
masked=mask&a
if masked:
if binary_aces.has_key(masked):
result=binary_aces[masked]+':'+result
return result
这是一个完整的pythonic方法,可以在Windows下的NTFS文件上递归或不递归地设置文件所有权/ ACL,有或没有ACL继承。
[编辑]
我现在已将此代码作为 Python 包windows_tools.file_utils
,请在此处查看源代码
[/编辑]
首先,我们需要一个可以获取文件所有权的函数(set_file_owner())然后我们需要一个可以处理 Windows 上的 ACL 的函数(set_acls())。
为了简化权限,我们将有一个函数 (easy_permissions()) 将 R、RX、M、F 权限转换为权限位掩码。
一旦我们得到了它,我们就可以递归地将 os.listdir 放入一个目录中(使用 get_files_recursive()),并在 PermissionError 上执行一个函数来处理权限。 完成后,只需再次循环所有文件并相应地设置权限。
底层代码:
import os
from fnmatch import fnmatch
from itertools import chain
import win32api
import win32security
import ntsecuritycon
import pywintypes
def glob_path_match(path, pattern_list):
"""
Checks if path is in a list of glob style wildcard paths
:param path: path of file / directory
:param pattern_list: list of wildcard patterns to check for
:return: Boolean
"""
return any(fnmatch(path, pattern) for pattern in pattern_list)
def get_files_recursive(root, d_exclude_list=None, f_exclude_list=None,
ext_exclude_list=None, ext_include_list=None,
depth=0, primary_root=None, fn_on_perm_error=None,
include_dirs=False):
"""
Walk a path to recursively find files
Modified version of https://stackoverflow.com/a/24771959/2635443 that includes exclusion lists
and accepts glob style wildcards on files and directories
:param root: (str) path to explore
:param include_dirs: (bool) should output list include directories
:param d_exclude_list: (list) list of root relative directories paths to exclude
:param f_exclude_list: (list) list of filenames without paths to exclude
:param ext_exclude_list: list() list of file extensions to exclude, ex: ['.log', '.bak'],
takes precedence over ext_include_list
:param ext_include_lsit: (list) only include list of file extensions, ex: ['.py']
:param depth: (int) depth of recursion to acheieve, 0 means unlimited, 1 is just the current dir...
:param primary_root: (str) Only used for internal recursive exclusion lookup, don't pass an argument here
:param fn_on_perm_error: (function) Optional function to pass, which argument will be the file / directory that has permission errors
:return: list of files found in path
"""
# Make sure we don't get paths with antislashes on Windows
if os.path.isdir(root):
root = os.path.normpath(root)
else:
return root
# Check if we are allowed to read directory, if not, try to fix permissions if fn_on_perm_error is passed
try:
os.listdir(root)
except PermissionError:
if fn_on_perm_error is not None:
fn_on_perm_error(root)
# Make sure we clean d_exclude_list only on first function call
if primary_root is None:
if d_exclude_list is not None:
# Make sure we use a valid os separator for exclusion lists
d_exclude_list = [os.path.normpath(d) for d in d_exclude_list]
else:
d_exclude_list = []
if f_exclude_list is None:
f_exclude_list = []
if ext_exclude_list is None:
ext_exclude_list = []
def _find_files():
try:
if include_dirs:
yield root
for f in os.listdir(root):
file_ext = os.path.splitext(f)[1]
if os.path.isfile(os.path.join(root, f)) and not glob_path_match(f, f_exclude_list) \
and file_ext not in ext_exclude_list \
and (file_ext in ext_include_list if ext_include_list is not None else True):
yield os.path.join(root, f)
except PermissionError:
pass
def _find_files_in_dirs(depth):
if depth == 0 or depth > 1:
depth = depth - 1 if depth > 1 else 0
try:
for d in os.listdir(root):
d_full_path = os.path.join(root, d)
if os.path.isdir(d_full_path):
# p_root is the relative root the function has been called with recursively
# Let's check if p_root + d is in d_exclude_list
p_root = os.path.join(primary_root, d) if primary_root is not None else d
if not glob_path_match(p_root, d_exclude_list):
files_in_d = get_files_recursive(d_full_path,
d_exclude_list=d_exclude_list,
f_exclude_list=f_exclude_list,
ext_exclude_list=ext_exclude_list,
ext_include_list=ext_include_list,
depth=depth, primary_root=p_root,
fn_on_perm_error=fn_on_perm_error,
include_dirs=include_dirs)
if include_dirs:
yield d
if files_in_d:
for f in files_in_d:
yield f
except PermissionError:
pass
# Chain both generators
return chain(_find_files(), _find_files_in_dirs(depth))
def get_binary_sid(string=None):
"""
Wrapper function that returns PySID object from SID identifier or username
If none given, we'll get current user
:param string: (str) SID identifier or username
:return: (PySID) object
"""
if string is None:
string = win32api.GetUserName()
if string.startswith('S-1-'):
# Consider we deal with a sid string
return win32security.GetBinarySid(string)
else:
# Try to resolve username
# LookupAccountName returns tuple (user, domain, type)
try:
user, _, _ = win32security.LookupAccountName('', string)
print(user)
return user
except pywintypes.error as e:
raise OSError('Cannot map security ID: {0} with name. {1}'.format(string, e))
def set_file_owner(path, owner=None, force=False):
"""
Set owner on NTFS files / directories
https://stackoverflow.com/a/61009508/2635443
:param path: (str) path
:param owner: (PySID) object that represents the security identifier. If not set, current security identifier will be used
:param force: (bool) Shall we force take ownership
:return:
"""
try:
hToken = win32security.OpenThreadToken(win32api.GetCurrentThread(),
win32security.TOKEN_ALL_ACCESS, True)
except win32security.error:
hToken = win32security.OpenProcessToken(win32api.GetCurrentProcess(),
win32security.TOKEN_ALL_ACCESS)
if owner is None:
owner = win32security.GetTokenInformation(hToken, win32security.TokenOwner)
prev_state = ()
if force:
new_state = [(win32security.LookupPrivilegeValue(None, name),
win32security.SE_PRIVILEGE_ENABLED)
for name in (win32security.SE_TAKE_OWNERSHIP_NAME,
win32security.SE_RESTORE_NAME)]
prev_state = win32security.AdjustTokenPrivileges(hToken, False,
new_state)
try:
sd = win32security.SECURITY_DESCRIPTOR()
sd.SetSecurityDescriptorOwner(owner, False)
win32security.SetFileSecurity(path, win32security.OWNER_SECURITY_INFORMATION, sd)
except pywintypes.error as e:
# Let's raise OSError so we don't need to import pywintypes in parent module to catch the exception
raise OSError('Cannot take ownership of file: {0}. {1}.'.format(path, e))
finally:
if prev_state:
win32security.AdjustTokenPrivileges(hToken, False, prev_state)
def easy_permissions(permission):
"""
Creates ntsecuritycon permission bitmask from simple rights
:param permission: (str) Simple R, RX, RWX, F rights
:return: (int) ntsecuritycon permission bitmask
"""
permission = permission.upper()
if permission == 'R':
return ntsecuritycon.GENERIC_READ
if permission == 'RX':
return ntsecuritycon.GENERIC_READ | ntsecuritycon.GENERIC_EXECUTE
if permission in ['RWX', 'M']:
return ntsecuritycon.GENERIC_READ | ntsecuritycon.GENERIC_WRITE | ntsecuritycon.GENERIC_EXECUTE
if permission == 'F':
return ntsecuritycon.GENERIC_ALL
raise ValueError('Bogus easy permission')
def set_acls(path, user_list=None, group_list=None, owner=None, permission=None, inherit=False, inheritance=False):
"""
Set Windows DACL list
:param path: (str) path to directory/file
:param user_sid_list: (list) str usernames or PySID objects
:param group_sid_list: (list) str groupnames or PySID objects
:param owner: (str) owner name or PySID obect
:param permission: (int) permission bitmask
:param inherit: (bool) inherit parent permissions
:param inheritance: (bool) apply ACL to sub folders and files
"""
if inheritance:
inheritance_flags = win32security.CONTAINER_INHERIT_ACE | win32security.OBJECT_INHERIT_ACE
else:
inheritance_flags = win32security.NO_INHERITANCE
security_descriptor = {'AccessMode': win32security.GRANT_ACCESS,
'AccessPermissions': 0,
'Inheritance': inheritance_flags,
'Trustee': {'TrusteeType': '',
'TrusteeForm': win32security.TRUSTEE_IS_SID,
'Identifier': ''}
}
# Now create a security descriptor for each user in the ACL list
security_descriptors = []
# If no user / group is defined, let's take current user
if user_list is None and group_list is None:
user_list = [get_binary_sid()]
if user_list is not None:
for sid in user_list:
sid = get_binary_sid(sid)
s = security_descriptor
s['AccessPermissions'] = permission
s['Trustee']['TrusteeType'] = win32security.TRUSTEE_IS_USER
s['Trustee']['Identifier'] = sid
security_descriptors.append(s)
if group_list is not None:
for sid in group_list:
sid = get_binary_sid(sid)
s = security_descriptor
s['AccessPermissions'] = permission
s['Trustee']['TrusteeType'] = win32security.TRUSTEE_IS_GROUP
s['Trustee']['Identifier'] = sid
security_descriptors.append(s)
try:
sd = win32security.GetNamedSecurityInfo(path, win32security.SE_FILE_OBJECT,
win32security.DACL_SECURITY_INFORMATION | win32security.UNPROTECTED_DACL_SECURITY_INFORMATION)
except pywintypes.error as e:
raise OSError('Failed to read security for file: {0}. {1}'.format(path, e))
dacl = sd.GetSecurityDescriptorDacl()
dacl.SetEntriesInAcl(security_descriptors)
security_information_flags = win32security.DACL_SECURITY_INFORMATION
if not inherit:
# PROTECTED_DACL_SECURITY_INFORMATION disables inheritance from parent
security_information_flags = security_information_flags | win32security.PROTECTED_DACL_SECURITY_INFORMATION
else:
security_information_flags = security_information_flags | win32security.UNPROTECTED_DACL_SECURITY_INFORMATION
# If we want to change owner, SetNamedSecurityInfo will need win32security.OWNER_SECURITY_INFORMATION in SECURITY_INFORMATION
if owner is not None:
security_information_flags = security_information_flags | win32security.OWNER_SECURITY_INFORMATION
if isinstance(owner, str):
owner = get_binary_sid(owner)
try:
# SetNamedSecurityInfo(path, object_type, security_information, owner, group, dacl, sacl)
win32security.SetNamedSecurityInfo(path, win32security.SE_FILE_OBJECT,
security_information_flags,
owner, None, dacl, None)
except pywintypes.error as e:
raise OSError
def take_ownership_recursive(path, owner=None):
def take_own(path):
nonlocal owner
try:
set_file_owner(path, owner=owner, force=True)
except OSError:
print('Permission error on: {0}.'.format(path))
files = get_files_recursive(path, include_dirs=True, fn_on_perm_error=take_own)
for file in files:
set_file_owner(file, force=True)
def get_files_recursive_and_set_permissions(path, owner=None, permissions=None, user_list=None):
def fix_perms(path):
nonlocal permissions
nonlocal owner
nonlocal user_list
if permissions == None:
permissions = easy_permissions('F')
print('Permission error on: {0}.'.format(path))
try:
set_acls(path, user_list=user_list, owner=owner, permission=permissions, inheritance=False)
except OSError:
# Lets force ownership change
try:
set_file_owner(path, force=True)
# Now try again
set_acls(path, user_list=user_list, owner=owner, permission=permissions, inheritance=False)
except OSError as e:
print('Cannot fix permission on {0}. {1}'.format(path, e))
files = get_files_recursive(path, include_dirs=True, fn_on_perm_error=fix_perms)
for file in files:
set_acls(file, user_list=user_list, owner=owner, permission=easy_permissions('F'), inheritance=False)
以下是如何使用代码的一些示例:
# Recursively set owner
take_ownership_recursive(r'C:\MYPATH', owner=get_binary_sid('MyUser'))
# Recursively set permissions
get_files_recursive_and_set_permissions(r'C;\MYPATH', permissions=easy_permissions('F'), user_list=['MyUser', 'MyOtherUser'])
# Recursively set permissions with inheritance
get_files_recursive_and_set_permissions(r'C:\MYPATH', permissions=easy_permissions('RX'), user_list=['S-1-5-18'], inheritance=True)
# Set permissions
set_acls(r'C:\MYPATH', permissions=easy_permissions('F'), user_list['MyUser'])
# Take ownership
set_file_owner(r'C:\MYPATH', owner=get_binary_sid('MyUser'), Force=True)
非常感谢 Eryk Sun 的所有关于在 Python 中处理 win32security 文件的帖子,这些帖子使编写正确的代码成为可能。 见https://stackoverflow.com/a/43244697/2635443和https://stackoverflow.com/a/61009508/2635443
这是最简单的解决方案(您还可以指定用户并添加修改权限):
command = r'icacls "C:\Users\PC\PycharmProjects\IIS_configuration\someFolder" /grant "userA":(OI)(CI)RWRXM'
appcmd = subprocess.run(command, shell=True, check=True)
要查看它是如何工作的,您可以通过 CMD 运行:
icacls "C:\Users\PC\PycharmProjects\IIS_configuration\someFolder" /grant "userA":(OI)(CI)RWRXM
我使用了 icacls(windows 命令)——你可以在这里和这里找到有关它的信息。
然后我就用 python子进程运行了这个命令
有关此特定命令的信息:
可以使用Python for .NET包分配 Windows 文件夹权限。 这个包让程序员可以直接在 Python 中使用 .NET 结构。 这种方法的一个优点是强大的 .NET 在线文档,它将帮助识别特定的函数调用和使用权限。 例如,所有可能的文件/文件夹权限都记录在FileSystemRights Enum 的文章中。
请注意,尝试将“None”的枚举值直接作为属性访问会导致 Python 语法错误。 getattr()
函数可用作解决方法。
import clr
import System
from System.IO import Directory
from System.Security.AccessControl import (
AccessControlType,
FileSystemAccessRule,
FileSystemRights,
InheritanceFlags,
PropagationFlags,
)
path = r"C:\path\to\folder"
accessControl = Directory.GetAccessControl(path)
accessRule = FileSystemAccessRule(
"UserX",
FileSystemRights.Modify | FileSystemRights.Synchronize,
InheritanceFlags.ContainerInherit | InheritanceFlags.ObjectInherit,
getattr(PropagationFlags, "None"),
AccessControlType.Allow,
)
accessControl.AddAccessRule(accessRule)
Directory.SetAccessControl(path, accessControl)
使用 os.chmod
http://docs.python.org/library/os.html#os.chmod
您可以使用 os.chmod 设置权限
mod 是用 base 8 写的,如果你把它转换成二进制,那就是
000 111 111 000 rwx rwx rwx 第一个rwx是所有者,第二个是组,第三个是世界
r=读,w=写,x=执行
您最常看到的权限是 7 读/写/执行 - 您需要执行目录才能查看内容 6 读/写 4 只读
当您使用 os.chmod 时,使用八进制表示法最有意义,因此
os.chmod('myfile',0o666) # read/write by everyone
os.chmod('myfile',0o644) # read/write by me, readable for everone else
请记住我说过您通常希望目录是“可执行的”,以便您可以看到内容。
os.chmod('mydir',0o777) # read/write by everyone
os.chmod('mydir',0o755) # read/write by me, readable for everone else
注意:0o777 的语法适用于 Python 2.6 和 3+。 否则,对于 2 系列,它是 0777。2.6 接受任一语法,因此您选择的语法取决于您是要向前兼容还是向后兼容。
首先,如果用户的配置文件目录不存在,则会自动创建该目录,并将权限设置为合理的默认值。 除非您有使用 python 的特定需求,否则您可以让 Windows 创建文件夹并为您整理权限。
如果你无论如何都想使用 python,你可以考虑只使用 os.system() 用正确的参数调用 cacls 或 icacls。 而不是权限,您可能只需要将文件夹的所有者更改为将拥有该文件夹的用户。
祝你的努力好运。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.