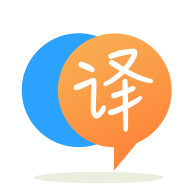
[英]How to write a Python script to download file from the link that starts downloading automatically when clicked?
[英]How to write a python script for downloading?
我想从这个站点下载一些文件: http : //www.emuparadise.me/soundtracks/highquality/index.php
但我只想得到某些。
有没有办法编写一个python脚本来做到这一点? 我有python的中级知识
我只是在寻找一些指导,请指点我去维基或图书馆来完成这个
谢谢,灌木
我看着页面。 这些链接似乎重定向到托管文件的另一个页面,单击该页面即可下载文件。
我会使用mechanize跟随所需的链接到正确的页面,然后使用BeautifulSoup或lxml来解析结果页面以获取文件名。
然后是使用urlopen打开文件并将其内容写入本地文件的简单问题,如下所示:
f = open(localFilePath, 'w')
f.write(urlopen(remoteFilePath).read())
f.close()
希望有帮助
为页面发出 url 请求。 获得源后,过滤并获取 url。
您要下载的文件是包含特定扩展名的 url。 正是有了这个,您可以对符合您的条件的所有网址进行正则表达式搜索。 过滤后,再对每个匹配到的url的数据做一个url请求,写入内存。
示例代码:
#!/usr/bin/python
import re
import sys
import urllib
#Your sample url
sampleUrl = "http://stackoverflow.com"
urlAddInfo = urllib.urlopen(sampleUrl)
data = urlAddInfo.read()
#Sample extensions we'll be looking for: pngs and pdfs
TARGET_EXTENSIONS = "(png|pdf)"
targetCompile = re.compile(TARGET_EXTENSIONS, re.UNICODE|re.MULTILINE)
#Let's get all the urls: match criteria{no spaces or " in a url}
urls = re.findall('(https?://[^\s"]+)', data, re.UNICODE|re.MULTILINE)
#We want these folks
extensionMatches = filter(lambda url: url and targetCompile.search(url), urls)
#The rest of the unmatched urls for which the scrapping can also be repeated.
nonExtMatches = filter(lambda url: url and not targetCompile.search(url), urls)
def fileDl(targetUrl):
#Function to handle downloading of files.
#Arg: url => a String
#Output: Boolean to signify if file has been written to memory
#Validation of the url assumed, for the sake of keeping the illustration short
urlAddInfo = urllib.urlopen(targetUrl)
data = urlAddInfo.read()
fileNameSearch = re.search("([^\/\s]+)$", targetUrl) #Text right before the last slash '/'
if not fileNameSearch:
sys.stderr.write("Could not extract a filename from url '%s'\n"%(targetUrl))
return False
fileName = fileNameSearch.groups(1)[0]
with open(fileName, "wb") as f:
f.write(data)
sys.stderr.write("Wrote %s to memory\n"%(fileName))
return True
#Let's now download the matched files
dlResults = map(lambda fUrl: fileDl(fUrl), extensionMatches)
successfulDls = filter(lambda s: s, dlResults)
sys.stderr.write("Downloaded %d files from %s\n"%(len(successfulDls), sampleUrl))
#You can organize the above code into a function to repeat the process for each of the
#other urls and in that way you can make a crawler.
上面的代码主要是针对Python2.X编写的。 但是, 我写了一个爬虫,适用于从 2.X 开始的任何版本
为什么是! 5 年后,这不仅是可能的,而且您现在有很多方法可以做到。
我将避免在这里使用代码示例,因为主要是想帮助将您的问题分解为多个部分并为您提供一些探索选项:
如果您必须坚持使用stdlib
,对于 python2 或 python3, urllib[n]
*是您要用来从互联网上下拉某些东西的东西。
再说一次,如果您不想依赖其他软件包:
urllib
或urllib2
或者另一个urllib[n]
我忘记了。你很幸运!!!!! 你有:
requests
与文档在这里。 requests
是使用 python 从网络上获取东西的黄金标准。 我建议你使用它。uplink
与文档在这里。 它相对较新,适用于更多程序化的客户端界面。aiohttp
通过asyncio
与 docs here 。 asyncio
仅包含在 python >= 3.5 中,这也更加令人困惑。 也就是说,如果您愿意投入时间,那么对于这个用例来说,它的效率会高得离谱。......我也有失职,更不用说我最喜欢的爬行工具之一:
fake_useragent
回购在这里。 文档之类的,真的没有必要。 因此,如果您必须坚持使用 stdlib 并且不使用pip
安装任何东西,您就可以使用额外的额外乐趣和安全 (<==extreme-sarcasm) xml
内置模块。 具体来说,您可以使用:
xml.etree.ElementTree()
与 docs here 。 值得注意的是ElementTree
对象是 pip-downloadable lxml
包的基础,并且使其更易于使用。 如果你想重新创建轮子并编写一堆你自己的复杂逻辑,使用默认的xml
模块是你的选择。
lxml
在这里。 正如我之前所说, lxml
是xml.etree
的包装器,它使它可供人类使用并实现您需要自己制作的所有解析工具。 但是,正如您通过访问文档所看到的,它本身并不容易使用。 这让我们...BeautifulSoup
又名bs4
, 这里有文档。 BeautifulSoup 让一切变得更简单。 这是我的建议。 这部分几乎与“Segment 1”完全相同,只是你有一堆链接而不是一个。 这部分和“段1”之间的变化是我用什么推荐的唯一的事: aiohttp
这里有多个URL打交道时,因为它会下载方式快,您可以下载它们并行。 **
* - (其中n
以某种令人沮丧的任意方式从 python-version 确定为 ptyhon-version。查找哪个urllib[n]
将.urlopen()
作为顶级函数。您可以阅读有关此命名的更多信息- 约定 clusterf**k此处、 此处和此处。)
** - (这并不完全正确。在人类时间尺度上它在功能上更真实。)
我会使用 wget 的组合进行下载 - http://www.thegeekstuff.com/2009/09/the-ultimate-wget-download-guide-with-15-awesome-examples/#more-1885和 BeautifulSoup http: //www.crummy.com/software/BeautifulSoup/bs4/doc/用于解析下载的文件
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.