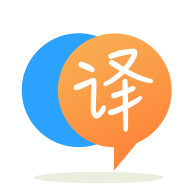
[英]Python: How to simplify multiple statements with the same try/except block
[英]How to write multiple try statements in one block in python?
我想要做:
try:
do()
except:
do2()
except:
do3()
except:
do4()
如果 do() 失败,则执行 do2(),如果 do2() 也失败,则执行 do3() 等等。
最好的祝福
如果你真的不关心异常,你可以循环遍历案例直到你成功:
for fn in (do, do2, do3, do4):
try:
fn()
break
except:
continue
这至少避免了每个案例都必须缩进一次。 如果不同的函数需要不同的参数,你可以使用functools.partial在循环之前“填充”它们。
我会为此写一个快速包装函数first()
。
用法 : value = first([f1, f2, f3, ..., fn], default='All failed')
#!/usr/bin/env
def first(flist, default=None):
""" Try each function in `flist` until one does not throw an exception, and
return the return value of that function. If all functions throw exceptions,
return `default`
Args:
flist - list of functions to try
default - value to return if all functions fail
Returns:
return value of first function that does not throw exception, or
`default` if all throw exceptions.
TODO: Also accept a list of (f, (exceptions)) tuples, where f is the
function as above and (exceptions) is a tuple of exceptions that f should
expect. This allows you to still re-raise unexpected exceptions.
"""
for f in flist:
try:
return f()
except:
continue
else:
return default
# Testing.
def f():
raise TypeError
def g():
raise IndexError
def h():
return 1
# We skip two exception-throwing functions and return value of the last.
assert first([f, g, h]) == 1
assert first([f, g, f], default='monty') == 'monty'
这似乎是一件非常奇怪的事情,但我可能会在没有异常的情况下循环使用函数并突破:
for func in [do, do2, do3]:
try:
func()
except Exception:
pass
else:
break
您应该指定每次尝试捕获的异常类型。
try:
do()
except TypeError: #for example first one - TypeError
do_2()
except KeyError: #for example second one - KeyError
do_3()
等等。
这是我发现的最简单的方法,只是将尝试嵌入前面的除外。
try:
do()
except:
try:
do2()
except:
do3()
如果你想要多个 try 语句,你可以这样做,包括 except 语句。 提取(重构)您的陈述。 并使用 and 和 or 的魔力来决定何时短路。
python def a(): try: # a code except: pass # or raise else: return True
def b():
try: # b code
except: pass # or raise
else: return True
def c():
try: # c code
except: pass # or raise
else: return True
def d():
try: # d code
except: pass # or raise
else: return True
def main():
try:
a() and b() or c() or d()
except:
pass
import sys
try:
f = open('myfile.txt')
s = f.readline()
i = int(s.strip())
except OSError as err:
print("OS error: {0}".format(err))
except ValueError:
print("Could not convert data to an integer.")
except:
print("Unexpected error:", sys.exc_info()[0])
raise
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.