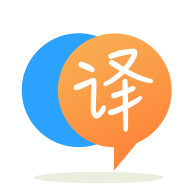
[英]LINQ error: variable 'x' of type 'y' referenced from scope '', but it is not defined
[英]LINQ expressions. Variable 'p' of type referenced from scope, but it is not defined
我正在使用此代碼動態構建LINQ查詢。 它似乎工作,但當我在我的搜索中有多個searchString時,(所以當添加多個表達式時,我得到以下錯誤:
從范圍引用的變量'p',但未定義**
我想我只能定義/使用一次。 但是,如果是這樣,我需要稍微改變我的代碼。 任何人都能指出我在正確的方向嗎?
if (searchStrings != null)
{
foreach (string searchString in searchStrings)
{
Expression<Func<Product, bool>> containsExpression = p => p.Name.Contains(searchString);
filterExpressions.Add(containsExpression);
}
}
Func<Expression, Expression, BinaryExpression>[] operators = new Func<Expression, Expression, BinaryExpression>[] { Expression.AndAlso };
Expression<Func<Product, bool>> filters = this.CombinePredicates<Product>(filterExpressions, operators);
IQueryable<Product> query = cachedProductList.AsQueryable().Where(filters);
query.Take(itemLimit).ToList(); << **error when the query executes**
public Expression<Func<T, bool>> CombinePredicates<T>(IList<Expression<Func<T, bool>>> predicateExpressions, Func<Expression, Expression, BinaryExpression> logicalFunction)
{
Expression<Func<T, bool>> filter = null;
if (predicateExpressions.Count > 0)
{
Expression<Func<T, bool>> firstPredicate = predicateExpressions[0];
Expression body = firstPredicate.Body;
for (int i = 1; i < predicateExpressions.Count; i++)
{
body = logicalFunction(body, predicateExpressions[i].Body);
}
filter = Expression.Lambda<Func<T, bool>>(body, firstPredicate.Parameters);
}
return filter;
}
簡化,這里有幾行你要嘗試(我使用字符串代替產品等,但想法是一樣的):
Expression<Func<string, bool>> c1 = x => x.Contains("111");
Expression<Func<string, bool>> c2 = y => y.Contains("222");
var sum = Expression.AndAlso(c1.Body, c2.Body);
var sumExpr = Expression.Lambda(sum, c1.Parameters);
sumExpr.Compile(); // exception here
請注意我是如何將foreach擴展為帶有x和y的兩個表達式 - 這正是編譯器的樣子,它們是不同的參數。
換句話說,你正試圖做這樣的事情:
x => x.Contains("...") && y.Contains("...");
和編譯器想知道'y'變量是什么?
要修復它,我們需要為所有表達式使用完全相同的參數(不僅僅是名稱,還要引用)。 我們可以修復這個簡化的代碼:
Expression<Func<string, bool>> c1 = x => x.Contains("111");
Expression<Func<string, bool>> c2 = y => y.Contains("222");
var sum = Expression.AndAlso(c1.Body, Expression.Invoke(c2, c1.Parameters[0])); // here is the magic
var sumExpr = Expression.Lambda(sum, c1.Parameters);
sumExpr.Compile(); //ok
所以,修復原始代碼就像:
internal static class Program
{
public class Product
{
public string Name;
}
private static void Main(string[] args)
{
var searchStrings = new[] { "111", "222" };
var cachedProductList = new List<Product>
{
new Product{Name = "111 should not match"},
new Product{Name = "222 should not match"},
new Product{Name = "111 222 should match"},
};
var filterExpressions = new List<Expression<Func<Product, bool>>>();
foreach (string searchString in searchStrings)
{
Expression<Func<Product, bool>> containsExpression = x => x.Name.Contains(searchString); // NOT GOOD
filterExpressions.Add(containsExpression);
}
var filters = CombinePredicates<Product>(filterExpressions, Expression.AndAlso);
var query = cachedProductList.AsQueryable().Where(filters);
var list = query.Take(10).ToList();
foreach (var product in list)
{
Console.WriteLine(product.Name);
}
}
public static Expression<Func<T, bool>> CombinePredicates<T>(IList<Expression<Func<T, bool>>> predicateExpressions, Func<Expression, Expression, BinaryExpression> logicalFunction)
{
Expression<Func<T, bool>> filter = null;
if (predicateExpressions.Count > 0)
{
var firstPredicate = predicateExpressions[0];
Expression body = firstPredicate.Body;
for (int i = 1; i < predicateExpressions.Count; i++)
{
body = logicalFunction(body, Expression.Invoke(predicateExpressions[i], firstPredicate.Parameters));
}
filter = Expression.Lambda<Func<T, bool>>(body, firstPredicate.Parameters);
}
return filter;
}
}
但請注意輸出:
222 should not match
111 222 should match
不是你可能期望的......這是在foreach中使用searchString的結果,應該用以下方式重寫:
...
foreach (string searchString in searchStrings)
{
var name = searchString;
Expression<Func<Product, bool>> containsExpression = x => x.Name.Contains(name);
filterExpressions.Add(containsExpression);
}
...
這是輸出:
111 222 should match
恕我直言,無需列出清單:
var filterExpressions = new List<Expression<Func<Product, bool>>>()
您可以在Visitor類中輕松使用以下內容:
public class FilterConverter : IFilterConverterVisitor<Filter> {
private LambdaExpression ConditionClausePredicate { get; set; }
private ParameterExpression Parameter { get; set; }
public void Visit(Filter filter) {
if (filter == null) {
return;
}
if (this.Parameter == null) {
this.Parameter = Expression.Parameter(filter.BaseType, "x");
}
ConditionClausePredicate = And(filter);
}
public Delegate GetConditionClause() {
if (ConditionClausePredicate != null) {
return ConditionClausePredicate.Compile();
}
return null;
}
private LambdaExpression And(Filter filter) {
if (filter.BaseType == null || string.IsNullOrWhiteSpace(filter.FlattenPropertyName)) {
//Something is wrong, passing by current filter
return ConditionClausePredicate;
}
var conditionType = filter.GetCondition();
var propertyExpression = filter.BaseType.GetFlattenPropertyExpression(filter.FlattenPropertyName, this.Parameter);
switch (conditionType) {
case FilterCondition.Equal: {
var matchValue = TypeDescriptor.GetConverter(propertyExpression.ReturnType).ConvertFromString(filter.Match);
var propertyValue = Expression.Constant(matchValue, propertyExpression.ReturnType);
var equalExpression = Expression.Equal(propertyExpression.Body, propertyValue);
if (ConditionClausePredicate == null) {
ConditionClausePredicate = Expression.Lambda(equalExpression, this.Parameter);
} else {
ConditionClausePredicate = Expression.Lambda(Expression.And(ConditionClausePredicate.Body, equalExpression), this.Parameter);
}
break;
}
// and so on...
}
}
代碼不是最優的,我知道,我是初學者,還有很多東西要實現......但這些東西確實有效。 我們的想法是為每個Visitor類設置唯一的ParameterExpression,然后使用此參數構造表達式。 之后,只需連接每個LambdaExpression子句的所有表達式,並在需要時編譯為委托。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.