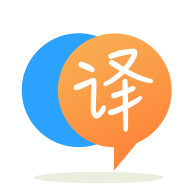
[英]C# How do I replace objects in one collection with Objects from another collection
[英]C# How can I do a string replace method on the collection?
我有以下方法返回一個集合,然后我可以在MVC中構建一個下拉菜單。 問題是,我想替換集合中的值中的子字符串,但我不知道如何在C#中這樣做(我是一個經典的vb家伙)。
public class RolesManagement
{
public static IEnumerable<string> BuildRoles(string DesiredRole)
{
var UserRoles = Roles.GetAllRoles();
IEnumerable<string> roles;
roles = UserRoles.Where(x => x.Contains("Sub")).Except(rejectAdmin);
return roles;
}
}
為簡潔起見,上述內容已經簡化。 角色集合中的每個角色如下所示:SubUser SubAdmin SubManager。
我只想返回用戶管理員
請問C#中最好的方法是什么?
我的猜測是我必須做一個foreach並替換每個循環上的子字符串並在移動到下一個項目之前重新填充該值。
如果你能提供一個很棒的代碼示例,因為我似乎還在喋喋不休地解決語法問題。
非常感激!
你可以用LINQ做到這一點。
roles = UserRoles
.Where(x => x.Contains("Sub"))
.Except(rejectAdmin)
.Select(x => x.Replace("Sub", ""));
編輯:請注意,此方法只是替換roles
中所有字符串中出現的所有字符串“Sub”。 這是一個非常廣泛的行程,如果你只想做你提到的替換,你可能需要使用不同的lambda函數。 請參閱Romoku的帖子以獲取幫助。
我會說,如果角色不會經常改變,那么制作一個映射Dictionary
。
public class RolesManagement
{
private static readonly Dictionary<string,string> RoleMapping =
new Dictionary<string,string>
{
{"SubUser", "User" },
{"SubManager", "Manager" },
{"SubAdmin", "Admin" }
};
public static IEnumerable<string> BuildRoles(string DesiredRole)
{
var UserRoles = Roles.GetAllRoles();
IEnumerable<string> roles;
roles = UserRoles.Where(x => x.Contains("Sub")).Except(rejectAdmin);
return roles.Select(r => RoleMapping.ContainsKey(r) ? RoleMapping[r] : r);
}
}
或者只是將它們作為FriendlyName
列存儲在數據庫中。
這將返回所有以sub開頭的項目,並刪除子
IEnumerable<string> = UserRoles .Where(x => x.StartsWith("sub")) .Select(y => y.Remove(0,3));
public static IEnumerable<string> GetCorrectedRoleNames(IEnumerable<string> roles)
{
List<string> correctedRoles = new List<string>();
foreach (string role in roles)
correctedRoles.Add(Regex.Replace(role, "Sub", string.Empty));
return correctedRoles.AsEnumerable();
}
public static IEnumerable<string> BuildRoles(string DesiredRole)
{
var UserRoles = Roles.GetAllRoles();
IEnumerable<string> roles;
roles = UserRoles.Where(x => x.Contains("Sub")).Except(rejectAdmin).Select(x=>x.Replace("Sub","");
return roles;
}
我認為x是string
變量,如果不是你必須在字符串集合上執行此替換
如果我理解你的問題,你需要刪除字符串中“Sub”的出現。 如果您有其他條件,可以執行其他檢查,例如“Sub”位於字符串的開頭,不區分大小寫等。
其中一種方法是找到要刪除的字符串的索引然后刪除。
var s = "SubUser";
var t = s.Remove(s.IndexOf("Sub"), "Sub".Length);
或者,您也可以使用空字符串替換要刪除的字符串。
var t = s.Replace("Sub", "");
希望有所幫助。
嘗試這個
List<string> x = new List<string>();
x.Add("SubUser");
x.Add("SubAdmin");
x.Add("SubManager");
var m = x.Select( y => y.Replace("Sub", ""));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.