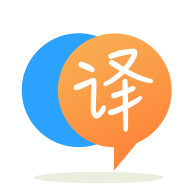
[英]How can i set a default value for a dependency property of type derived from dependencyobject
[英]How can I set default value to exceptions in automapper while mapping from string to int type property?
我想一個對象映射到另一個,但我得到的一個問題,同時映射一個空字符串為int類型或一個非整數字符串來int
,所以我想,如果我這樣的例外發生,必須某些默認值分配給它,假設-1。
例如我們有A
類和B
類
Class A
{
public string a{get;set;}
}
Class B
{
public int a{get;set;}
}
現在,如果我們使用默認規則從類A
映射到B
則它將在字符串為空或非整數的情況下通過異常。
請幫助我解決此問題。
提前致謝。
我想這就是你所追求的。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using AutoMapper;
using NUnit.Framework;
namespace StackOverFlowAnswers
{
public class LineItem
{
public int Id { get; set; }
public string ProductId { get; set; }
public int Amount { get; set; }
}
public class Model
{
public int Id { get; set; }
public string ProductId { get; set; }
public string Amount { get; set; }
}
public class AutoMappingTests
{
[TestFixtureSetUp]
public void TestFixtureSetUp()
{
Mapper.CreateMap<Model, LineItem>()
.ForMember(x => x.Amount, opt => opt.ResolveUsing<StringToInteger>());
}
[Test]
public void TestBadStringToDefaultInteger()
{
// Arrange
var model = new Model() {Id = 1, ProductId = "awesome-product-133-XP", Amount = "EVIL STRING, MWUAHAHAHAH"};
// Act
LineItem mapping1 = Mapper.Map<LineItem>(model);
// Assert
Assert.AreEqual(model.Id, mapping1.Id);
Assert.AreEqual(model.ProductId, mapping1.ProductId);
Assert.AreEqual(0, mapping1.Amount);
// Arrange
model.Amount = null; // now we test null, which we said in options to map from null to -1
// Act
LineItem mapping2 = Mapper.Map<LineItem>(model);
// Assert
Assert.AreEqual(-1, mapping2.Amount);
}
}
public class StringToInteger : ValueResolver<Model, int>
{
protected override int ResolveCore(Model source)
{
if (source.Amount == null)
{
return -1;
}
int value;
if (int.TryParse(source.Amount, out value))
{
return value; // Wahayy!!
}
return 0; // return 0 if it could not parse!
}
}
}
在共享一個我自己編寫的代碼時,上面的代碼也能工作
public class StringToIntTypeConverter : ITypeConverter<string, int>
{
public int Convert(ResolutionContext context)
{
int result;
if (!int.TryParse(context.SourceValue.ToString(), out result))
{
result = -1;
};
return result;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.