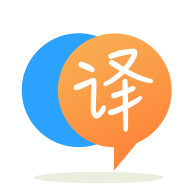
[英]c#: Using Linq to get a IEnumerable collection of XML Nodes to Traverse
[英]Get nodes from XML using LINQ in C#
我的代碼如下:
XDocument xmlDoc = XDocument.Load("myXML.xml");
var data = from items in xmlDoc.Root.Elements("category")
where items.Attribute("value").Value == "idxCategoryPlatformEngine"
select new
{
attribute = items.Element("attributes").Element("attribute").Element("name").Value,
trigger = items.Element("attributes").Element("attribute").Element("trigger").Value,
value = items.Element("attributes").Element("attribute").Element("value").Value,
minutes = items.Element("attributes").Element("attribute").Element("minutes").Value
};
我擁有的XML如下:
<?xml version="1.0" encoding="utf-8"?>
<GMS>
<category value="idxCategoryPlatformEngine">
<attributes>
<attribute>
<name>CheckpointFileCorruptionAlarm.InAlarm</name>
<trigger>EQ</trigger>
<value>TRUE</value>
<minutes>0</minutes>
</attribute>
<attribute>
<name>CPULoad</name>
<trigger>GT</trigger>
<value>60</value>
<minutes>5</minutes>
</attribute>
<attribute>
<name>Engine.Historian.InStoreForward</name>
<trigger>EQ</trigger>
<value>TRUE</value>
<minutes>0</minutes>
</attribute>
</attributes>
</category>
<category value="idxCategoryApplicationEngine">
<attributes>
<attribute>
<name>CheckpointFileCorruptionAlarm.InAlarm</name>
<trigger>EQ</trigger>
<value>TRUE</value>
<minutes>0</minutes>
</attribute>
<attribute>
<name>CPULoad</name>
<trigger>GT</trigger>
<value>60</value>
<minutes>5</minutes>
</attribute>
<attribute>
<name>Engine.Historian.InStoreForward</name>
<trigger>EQ</trigger>
<value>TRUE</value>
<minutes>0</minutes>
</attribute>
</attributes>
</category>
</GMS>
現在,當我運行代碼時,它會執行查詢,但是它只返回我實際上需要所有屬性的第一個屬性。
我很樂意提供任何幫助,因為它使我發瘋,我為嘗試解決此問題所做的每項更改只會導致更多問題!
問題在於,通過向下選擇select語句中的attribute元素,您只執行一次。 換句話說,您傳遞的是一個要選擇的集合,並且select正在運行諸如items.Element("attributes").Element("attribute").Element("name").Value
類的語句以獲取單個值和一個匿名對象。 它看起來應該更像這樣(有效):
var data2 = doc.Root.Elements("category")
.Where(x => x.Attribute("value").Value == "idxCategoryPlatformEngine")
.Elements("attributes")
.Elements("attribute")
.Select(x => new
{
attribute = x.Element("name").Value,
trigger = x.Element("trigger").Value,
value = x.Element("value").Value,
minutes = x.Element("minutes").Value
});
我對Linq的擴展語法比較滿意,但是可以輕松將其轉換為其他語法。
您應該在select new { }
添加一個附加的select
,現在只返回第一個attribute
值:
var data = from item in xmlDoc.Root.Elements("category")
where item.Attribute("value").Value == "idxCategoryPlatformEngine"
select new
{
category = item.Attribute("value").Value,
attributes = from attr in item.Element("attributes")
select new {
attribute = attr.Element("name").Value,
trigger = attr.Element("trigger").Value,
...
}
};
首先,您需要獲取Attributes
元素,然后迭代其下的所有Attribute
元素。
您可以這樣做:
XDocument xmlDoc = XDocument.Load("myXML.xml");
var data = (from items in xmlDoc.Root.Elements("category")
where items.Attribute("value").Value == "idxCategoryPlatformEngine"
select new {attrs = items.Element("attributes")})
.SelectMany(a => a.attrs.Elements("attribute"))
.Select(item => new {
attribute = item.Element("name").Value,
trigger = item.Element("trigger").Value,
value = item.Element("value").Value,
minutes = item.Element("minutes").Value
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.