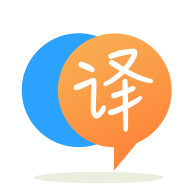
[英]Convert Hex To Byte Array in Java gives different results from C#.NET [port from C# to Java]
[英]Hex to Byte Array in C# and Java Gives Different Results
首先,對於長篇文章感到抱歉,我想包括我的所有想法,以便你們更容易找到我的代碼有什么問題。
我想將一個Hex字符串從C#應用程序傳輸到Java應用程序。 但是,當我在兩種語言中將相同的十六進制值轉換為字節數組時,輸出是不同的。
例如,相同的十六進制值給出
[101, 247, 11, 173, 46, 74, 56, 137, 185, 38, 40, 191, 204, 104, 83, 154]
在C#和
[101, -9, 11, -83, 46, 74, 56, -119, -71, 38, 40, -65, -52, 104, 83, -102]
在Java中
以下是我在C#中使用的方法:
public static string ByteArrayToHexString(byte[] byteArray)
{
return BitConverter.ToString(byteArray).Replace("-",""); //To convert the whole array
}
public static byte[] HexStringToByteArray(string hexString)
{
byte[] HexAsBytes = new byte[hexString.Length / 2];
for (int index = 0; index < HexAsBytes.Length; index++)
{
string byteValue = hexString.Substring(index * 2, 2);
HexAsBytes[index] = byte.Parse(byteValue, NumberStyles.HexNumber, CultureInfo.InvariantCulture);
}
return HexAsBytes;
}
Java中的那些:
public static String ByteArrayToHexString(byte[] bytes) {
StringBuilder builder = new StringBuilder();
for (byte b: bytes) {
builder.append(String.format("%02x", b));
}
return builder.toString().toUpperCase();
}
public static byte[] HexStringToByteArray(String s) {
int len = s.length();
byte[] data = new byte[len / 2];
for (int i = 0; i < len; i += 2) {
data[i / 2] = (byte) ((Character.digit(s.charAt(i), 16) << 4)
+ Character.digit(s.charAt(i+1), 16));
}
return data;
}
這是C#中的一個例子:
String hexString = "65F70BAD2E4A3889B92628BFCC68539A";
byte[] byteArray = HexBytes.HexStringToByteArray(hexString);
//Using the debugger, byteArray = [101, 247, 11, 173, 46, 74, 56, 137, 185, 38, 40, 191, 204, 104, 83, 154]
String hexString2 = HexBytes.ByteArrayToHexString(byteArray)
Console.Write("HEX: " + hexString2);
//Outputs 65F70BAD2E4A3889B92628BFCC68539A
以及Java中的一個例子:
String hexString = "65F70BAD2E4A3889B92628BFCC68539A";
byte[] byteArray = HexBytes.HexStringToByteArray(hexString);
//Using the debugger, byteArray = [101, -9, 11, -83, 46, 74, 56, -119, -71, 38, 40, -65, -52, 104, 83, -102]
String hexString2 = HexBytes.ByteArrayToHexString(byteArray);
System.out.println("HEX: " + hexString2);
//Outputs 65F70BAD2E4A3889B92628BFCC68539A
正如您所看到的,當我執行相反的操作時,最終的十六進制值等於第一個,這意味着我轉換的方式在兩種語言中都可能是單獨的。 但我不明白為什么在這兩種語言中從Hex到字節數組的轉換是不同的。 我認為Hexadecimal只是另一個基礎上的數字。
謝謝您的幫助
Cydrick
更新
我通過使用以下代碼替換C#代碼來解決此問題:
public static string ByteArrayToHexString(sbyte[] byteArray)
{
return BitConverter.ToString(convert(byteArray)).Replace("-", ""); //To convert the whole array
}
public static sbyte[] HexStringToByteArray(string hexString)
{
byte[] HexAsBytes = new byte[hexString.Length / 2];
for (int index = 0; index < HexAsBytes.Length; index++)
{
string byteValue = hexString.Substring(index * 2, 2);
HexAsBytes[index] = byte.Parse(byteValue, NumberStyles.HexNumber, CultureInfo.InvariantCulture);
}
return convert(HexAsBytes);
}
private static sbyte[] convert(byte[] byteArray)
{
sbyte[] sbyteArray = new sbyte[byteArray.Length];
for (int i = 0; i < sbyteArray.Length; i++)
{
sbyteArray[i] = unchecked((sbyte) byteArray[i]);
}
return sbyteArray;
}
private static byte[] convert(sbyte[] sbyteArray)
{
byte[] byteArray = new byte[sbyteArray.Length];
for (int i = 0; i < byteArray.Length; i++)
{
byteArray[i] = (byte) sbyteArray[i];
}
return byteArray;
}
但是,當我在兩種語言中將相同的十六進制值轉換為字節數組時,輸出是不同的。
您所看到的只是字節用Java簽名而在C#中無符號。 因此,如果在Java中向任何負值添加256,您將獲得C#中顯示的值。 值中的實際位是相同的 - 這只是頂部位是否被視為符號位的問題。
編輯:如注釋中所述,如果您在調試器輸出之外使用該字節作為整數,則可以始終使用:
int someInt = someByte & 0xff;
獲取無符號值。
看起來這只是一個調試器問題。 您在Java調試器中看到的那些負值是您在C#調試器中看到的無符號值的等效符號。 例如,有符號字節-9 ==無符號字節247(注意它們總是相差256)。 數據很好。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.