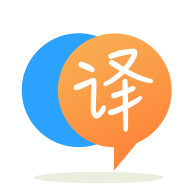
[英]C# HttpClient.SendAsync await throws NullReferenceException
[英]await PostAsync throws NullReferenceException in mscorlib
我需要將文件從一個Mvc4應用程序發送到另一個(另一個是Mvc4 WebApi應用程序)。 為了進行發送,我使用了HttpClient的PostAsync方法。 這是執行發送的代碼:
public class HomeController : Controller
{
public async Task<ActionResult> Index()
{
var result =
await Upload("http://localhost/target/api/test/post", "Test", System.IO.File.Open(@"C:\SomeFile", FileMode.Open, FileAccess.ReadWrite, FileShare.ReadWrite));
return View(result);
}
public async Task<String> Upload(String url, string filename, Stream stream)
{
using (var client = new HttpClient())
{
var formData = new MultipartFormDataContent();
var fileContent = new StreamContent(stream);
var header = new ContentDispositionHeaderValue("attachment") { FileName = filename };
fileContent.Headers.ContentDisposition = header;
formData.Add(fileContent);
var result = await client.PostAsync(url, formData); // Use your url here
return "123";
}
}
}
出於接收目的,我使用官方Web API教程中提供的示例之一,以下是執行此操作的代碼:
public class TestController : ApiController
{
// POST api/values
public Task<HttpResponseMessage> Post()
{
if (!Request.Content.IsMimeMultipartContent())
{
throw new HttpResponseException(HttpStatusCode.UnsupportedMediaType);
}
string root = HttpContext.Current.Server.MapPath("~/App_Data");
var provider = new MultipartFormDataStreamProvider(root);
// Read the form data and return an async task.
var task = Request.Content.ReadAsMultipartAsync(provider).ContinueWith(t =>
{
if (t.IsFaulted || t.IsCanceled)
{
return Request.CreateErrorResponse(HttpStatusCode.InternalServerError, t.Exception);
}
// This illustrates how to get the file names.
foreach (MultipartFileData file in provider.FileData)
{
Trace.WriteLine(file.Headers.ContentDisposition.FileName);
Trace.WriteLine("Server file path: " + file.LocalFileName);
}
return new HttpResponseMessage
{
Content = new StringContent("File uploaded.")
};
//var response = Request.CreateResponse(HttpStatusCode.OK);
//return response;
});
return task;
}
}
接收器成功獲取文件,但是當其使用以下代碼響應時:
return new HttpResponseMessage
{
Content = new StringContent("File uploaded.")
};
發送方在.Net的mscorlib內部的某個深處中斷。 即使我用try / catch包裹了await
調用,也不處理異常。
我想保留異步實現,並且不想使用同步,這可能嗎? 為什么會出現問題? 有什么想法嗎?
仔細檢查您的教程代碼。 我假設您正在談論此頁面 ,在這種情況下,您應該使用.NET 4.5版本的代碼(帶有await
),而不是舊的.NET 4.0示例。
只能從請求上下文訪問ASP.NET內在函數( HttpContext
,請求,響應等)。 您的代碼使用的是ContinueWith
而未指定TaskScheduler
,在這種情況下,這將導致lambda在請求上下文之外的線程池上運行。
在async
代碼中,您根本不應該使用ContinueWith
(除非您確實需要 ); 使用await
代替:
// POST api/values
public async Task<HttpResponseMessage> Post()
{
if (!Request.Content.IsMimeMultipartContent())
{
throw new HttpResponseException(HttpStatusCode.UnsupportedMediaType);
}
string root = HttpContext.Current.Server.MapPath("~/App_Data");
var provider = new MultipartFormDataStreamProvider(root);
try
{
// Read the form data.
await Request.Content.ReadAsMultipartAsync(provider);
// This illustrates how to get the file names.
foreach (MultipartFileData file in provider.FileData)
{
Trace.WriteLine(file.Headers.ContentDisposition.FileName);
Trace.WriteLine("Server file path: " + file.LocalFileName);
}
return new HttpResponseMessage
{
Content = new StringContent("File uploaded.")
};
}
catch (Exception ex)
{
return Request.CreateErrorResponse(HttpStatusCode.InternalServerError, ex);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.