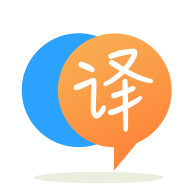
[英]C# Datagridview - Convert TextColumn to ComboBoxColumn
[英]DataGridView Convert TextBoxColumn to ComboBoxColumn
我有一個簡單的數據庫(SQL),汽車表有六個字段:ID,所有者,年份,顏色,品牌和型號。 顏色表就是:ID,ColorName。
我有一個帶有DataGridView的窗體。 當我將Cars表綁定到DataGridView時,到此為止一切正常。
現在,我正在嘗試使DataGridView中的“顏色”列成為用於編輯的comboBox,並僅列出我的“顏色表”中的顏色。 我可以制作一個comboBox並將其添加到DataGridView,但我想只是“轉換”已包含數據的列。
在此先感謝您提供的任何幫助。
using System.Collections.Generic;
using System.Linq;
using System.Windows.Forms;
namespace EditingCells
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
var myDataBase = new CarsEntities();
List<MyCar> data = myDataBase.MyCars.OrderBy(o => o.Owner).ToList();
//dataGridView1.AutoGenerateColumns = false;
dataGridView1.DataSource = data;
const int index = 2; // Column Index for Color
List<string> myColor = myDataBase.Colors.Select(s => s.Color1).ToList();
var comboBoxColumn = new DataGridViewComboBoxColumn
{
DataSource = myColor,
DisplayStyle = DataGridViewComboBoxDisplayStyle.Nothing,
AutoComplete = true,
};
}
private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
MessageBox.Show(dataGridView1.Rows[e.RowIndex].Cells[e.ColumnIndex].EditType.ToString());
}
}
}
基於此主題->“將Databound DataGridView上的單元格類型更改為Custom DataGridViewCell Type ”主題,建議您進行以下操作:
public partial class Form1 : Form
{
List<string> availableCarColors = new List<string>();
List<MyCar> data = new List<MyCar>();
public Form1()
{
InitializeComponent();
availableCarColors.Add("Red");
availableCarColors.Add("Blue");
data.Add(new MyCar("Lexus", "Blue"));
data.Add(new MyCar("BMW", "Red"));
DataGridViewTextBoxColumn colModel = new DataGridViewTextBoxColumn();
colModel.Name = "Model"; //Header Text
colModel.DataPropertyName = "Model"; //Property Name of my car class
DataGridViewComboBoxColumn colColor = new DataGridViewComboBoxColumn();
colColor.Name = "Color";
colColor.DataPropertyName = "Color";
colColor.DataSource = availableCarColors;
dataGridView1.AutoGenerateColumns = false;
dataGridView1.Columns.AddRange(new DataGridViewColumn[] {colModel, colColor});
dataGridView1.DataSource = data;
}
class MyCar
{
public string Model { get; set; }
public string Color { get; set; }
public MyCar(string model, string color)
{
Model = model;
Color = color;
}
}
}
您必須使用以下設置使用“顏色”表數據填充DataGridViewComboBoxColumn:
我只想發布如何使用@Tezzo的解決方案來獲得所需的結果:
using System.Collections.Generic;
using System.Linq;
using System.Windows.Forms;
namespace EditingCells
{
public partial class Form1 : Form
{
private readonly CarsEntities _myDataBase = new CarsEntities();
public Form1()
{
InitializeComponent();
List<MyCar> myData = _myDataBase.MyCars.ToList();
// Yes, you have do make Columns manually.
dataGridView1.AutoGenerateColumns = false;
dataGridView1.DataSource = myData;
List<Color> myColors = _myDataBase.Colors.ToList();
var carOwner = new DataGridViewTextBoxColumn
{
DataPropertyName = "Owner",
HeaderText = @"Owner",
Name = "Owner",
};
dataGridView1.Columns.Add(carOwner);
var carColor = new DataGridViewComboBoxColumn
{
// Field Name where existing data is stored
DataPropertyName = "CarColor",
HeaderText = @"Color",
Name = "Color",
DataSource = myColors,
DisplayStyle = DataGridViewComboBoxDisplayStyle.Nothing,
AutoComplete = true,
// Field value from DataSource to store in Car.Color field
ValueMember = "ColorName",
// Field value from DataSource to show in DataGridView Column
DisplayMember = "ColorName",
};
dataGridView1.Columns.Add(carColor);
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
_myDataBase.SaveChanges();
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.