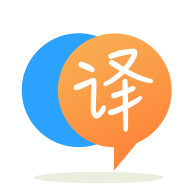
[英]How to check if string contains certain amounts of letters and numbers C#
[英]How to check repeated letters in a string c#
我正在創建一個檢查字符串中重復字母的程序。
例如:
嗚嗚嗚
快樂的
這是我的代碼:
string repeatedWord = "woooooooow";
for (int i = 0; i < repeatedWord.Count(); i++)
{
if (repeatedWord[i] == repeatedWord[i+1])
{
// ....
}
}
該代碼有效,但總是會出錯,因為最后一個字符[i + 1]
為空/null。
錯誤是索引超出了數組的范圍。
有什么解決辦法嗎?
運行循環直到repeatedWord.Count()-1
另一種選擇是使用匹配重復字符的正則表達式。 然后,對於每個匹配項,您可以使用Length
屬性獲取字符數。
string input = "wooooooow happppppppy";
var matches = Regex.Matches(input, @"(.)\1+");
for (int i = 0; i < matches.Count; i++)
{
Console.WriteLine("\"" + matches[i].Value + "\" is " + matches[i].Length + " characters long.");
//...
}
Console.Read();
正則表達式:
Regex rxContainsMultipleChars = new Regex( @"(?<char>.)\k<char>" , RegexOptions.ExplicitCapture|RegexOptions.Singleline ) ;
.
.
.
string myString = SomeStringValue() ;
bool containsDuplicates = rxDupes.Match(myString) ;
或 Linq
string s = SomeStringValue() ;
bool containsDuplicates = s.Where( (c,i) => i > 0 && c == s[i-1] )
.Cast<char?>()
.FirstOrDefault() != null
;
或滾你自己的:
public bool ContainsDuplicateChars( string s )
{
if ( string.IsNullOrEmpty(s) ) return false ;
bool containsDupes = false ;
for ( int i = 1 ; i < s.Length && !containsDupes ; ++i )
{
containsDupes = s[i] == s[i-1] ;
}
return containsDupes ;
}
甚至
public static class EnumerableHelpers
{
public static IEnumerable<Tuple<char,int>> RunLengthEncoder( this IEnumerable<char> list )
{
char? prev = null ;
int count = 0 ;
foreach ( char curr in list )
{
if ( prev == null ) { ++count ; prev = curr ; }
else if ( prev == curr ) { ++count ; }
else if ( curr != prev )
{
yield return new Tuple<char, int>((char)prev,count) ;
prev = curr ;
count = 1 ;
}
}
}
}
有了這最后一個...
bool hasDupes = s.RunLengthEncoder().FirstOrDefault( x => x.Item2 > 1 ) != null ;
要么
foreach (Tuple<char,int> run in myString.RunLengthEncoder() )
{
if ( run.Item2 > 1 )
{
// do something with the run of repeated chars.
}
}
只需“記住”我要說的最后一個字母。
string repeatedWord = "woooooooow";
if (string.IsNullOrEmpty( repeatedWord))
// empty. return, throw whatever.
char previousLetter = repeatedWord[0];
for (int i = 1; i < repeatedWord.Count(); i++)
{
if (repeatedWord[i] == previousLetter)
{
// ....
}
else
previousLetter = repeatedWord[i];
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Delegate
{
class Program
{
public int repeatcount(string str,char ch)
{
var count = 0;
for (int i = 0; i<str.Length; i++)
{
if (ch == str[i])
{
count++;
}
}
return count;
}
static void Main(string[] args)
{
Console.WriteLine("Enter a string");
string str = Console.ReadLine();
Console.WriteLine("Enter to know the reperted char");
char ch = Convert.ToChar(Console.ReadLine());
Program obj = new Program();
int p=obj.repeatcount(str, ch);
Console.WriteLine(p);
Console.ReadLine();
}
}
}
您可以將循環條件更改為-1
(正如其他人已經指出的那樣),或者您可以通過酷孩子的方式來實現。
var text = "wooooooooooow happpppppppy";
var repeats = text.Zip(text.Skip(1), (a, b) => a == b).Count(x => x);
public int RepeatedLetters(string word)
{
var count = 0;
for (var i = 0; i < word.Count()-1; i++)
{
if (word[i] == word[i+1])
{
count++;
}
}
return count;
}
using System;
namespace temp1
{
class Program
{
static string str = "proffession";
static int n = str.Length;
static string dupstr = "";
static int cnt = 0;
static void Main()
{
RepeatedCharsString();
}
public static void RepeatedCharsString()
{
for (int i = 0; i < n ; i++)
{
for(int j = i + 1; j <= n-1; j++)
{
if (str[i] == str[j])
{
dupstr = dupstr + str[i];
cnt = cnt + 1;
}
}
}
Console.WriteLine("Repeated chars are: " + dupstr);
Console.WriteLine("No of repeated chars are: " + cnt);
}
}
}
你也可以這樣做:
string myString = "longstringwithccc";
var list = new List<char>();
var duplicates = new List<char>();
foreach(char c in myString)
{
if (!list.Contains(c))
{
list.Add(c);
}
else
{
if (!duplicates.Contains(c))
{
duplicates.Add(c);
}
}
}
duplicates
將包含原始字符串中的重復字符。
list
將包含刪除重復項的原始字符串。
您可以按如下方式找到第一個不重復的數字。
static string intputString = "oossmmaanntuurrkt";
static void Main(string[] args)
{
Console.WriteLine(OutPutValue(intputString));
Console.ReadLine();
}
public static char OutPutValue(string strValue)
{
Dictionary<char, int> repeatValue = new Dictionary<char, int>();
char[] arrValue = intputString.ToCharArray();
foreach (var item in arrValue)
{
int repearCount = 0;
if (repeatValue.ContainsKey(item))
{
repeatValue[item] = repeatValue.FirstOrDefault(t => t.Key == item).Value + 1;
}
else
{
repearCount++;
repeatValue.Add(item, repearCount);
}
}
return repeatValue.FirstOrDefault(t => t.Value == 1).Key;
}
您可以獲得如下的重復次數和次數信息。
repeatValue.Where(t=> t.Value > 1)
您運行循環一次迭代的時間太長。
或者,您可以使用 LINQ 查找單詞中的唯一(不同)字符,然后檢查它們在單詞中的出現次數。 如果它出現不止一次,請對其進行處理。
void RepeatedLetters()
{
string word = "wooooooow";
var distinctChars = word.Distinct();
foreach (char c in distinctChars)
if (word.Count(p => p == c) > 1)
{
// do work on c
}
}
使用 C# 在給定字符串中查找重復或重復的字母
string str = "Welcome Programming";
char[] Array = str.ToCharArray();
var duplicates = Array.GroupBy(p => p).Where(g => g.Count() > 1).Select(g => g.Key).ToList();
string duplicateval= string.Join(",", duplicates.ToArray());
輸出:
e,o,m,r,g
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.