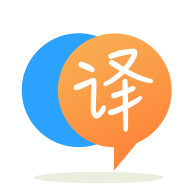
[英]How to hide a text inside a Powerpoint slide using OpenXML SDK?
[英]How to insert a shape in a powerpoint slide using OpenXML
這個問題看似相當基本,但如何在c#中使用OpenXML在幻燈片中插入形狀(即矩形)?
我四處搜索,我看到的只是“創建一個帶有形狀的幻燈片,並使用SDK生產力工具來反映代碼。這不是真的有用:(
在我的頭靠在牆上一段時間之后,最后我接受了建議,創建了一個帶有形狀的幻燈片並使用該工具來反映代碼。 因此,對於下一代,這里有一個簡要的解釋,說明如何手動完成。
你必須要知道的是,一個形狀插入到ShapeTree中,它是CommonSlideData的一部分:
Slide s = GetDesiredSlide(); // Get the slide where you want to insert the shape.
s.CommonSlideData.ShapeTree.Append(GenerateShape());
其次你應該知道一個形狀必須包含至少4個描述其行為的孩子:
在下面的示例代碼中,我使用以下命名空間和變量:
using System;
using System.Collections.Generic;
using System.Linq;
using DocumentFormat.OpenXml;
using DocumentFormat.OpenXml.Packaging;
using DocumentFormat.OpenXml.Presentation;
using D = DocumentFormat.OpenXml.Drawing;
D.ShapeTypeValues shapeType; // Any of the built-in shapes (ellipse, rectangle, etc)
string rgbColorHex; // Hexadecimal RGB color code to fill the shape.
long x; // Represents the shape x position in 1/36000 cm.
long y; // Represents the shape y position in 1/36000 cm.
long width; // Shapw width in in 1/36000 cm.
long heigth;// Shapw heigth in in 1/36000 cm.
ShapeStyle對象
形狀樣式對象描述一般形狀樣式屬性,如邊框,填充樣式,文本字體和視覺效果(陰影等)
ShapeStyle shapeStyle1 = new ShapeStyle();
D.LineReference lineReference1 = new D.LineReference() { Index = (UInt32Value)2U };
D.SchemeColor schemeColor2 = new D.SchemeColor() { Val = D.SchemeColorValues.Accent1 };
D.Shade shade1 = new D.Shade() { Val = 50000 };
schemeColor2.Append(shade1);
lineReference1.Append(schemeColor2);
D.FillReference fillReference1 = new D.FillReference() { Index = (UInt32Value)1U };
D.SchemeColor schemeColor3 = new D.SchemeColor() { Val = D.SchemeColorValues.Accent1 };
fillReference1.Append(schemeColor3);
D.EffectReference effectReference1 = new D.EffectReference() { Index = (UInt32Value)0U };
D.SchemeColor schemeColor4 = new D.SchemeColor() { Val = D.SchemeColorValues.Accent1 };
effectReference1.Append(schemeColor4);
D.FontReference fontReference1 = new D.FontReference() { Index = D.FontCollectionIndexValues.Minor };
D.SchemeColor schemeColor5 = new D.SchemeColor() { Val = D.SchemeColorValues.Light1 };
fontReference1.Append(schemeColor5);
shapeStyle1.Append(lineReference1);
shapeStyle1.Append(fillReference1);
shapeStyle1.Append(effectReference1);
shapeStyle1.Append(fontReference1);
ShapeProperties
定義形狀的視覺屬性,如填充方法(實體,漸變等)幾何(大小,位置,翻轉,旋轉)填充和輪廓。
ShapeProperties shapeProperties1 = new ShapeProperties();
D.Transform2D transform2D1 = new D.Transform2D();
D.Offset offset1 = new D.Offset() { X = x, Y = y };
D.Extents extents1 = new D.Extents() { Cx = width, Cy = heigth };
transform2D1.Append(offset1);
transform2D1.Append(extents1);
D.PresetGeometry presetGeometry1 = new D.PresetGeometry() { Preset = shapeType };
D.AdjustValueList adjustValueList1 = new D.AdjustValueList();
presetGeometry1.Append(adjustValueList1);
D.SolidFill solidFill1 = new D.SolidFill();
D.RgbColorModelHex rgbColorModelHex1 = new D.RgbColorModelHex() { Val = rgbColorHex };
solidFill1.Append(rgbColorModelHex1);
D.Outline outline1 = new D.Outline() { Width = 12700 };
D.SolidFill solidFill2 = new D.SolidFill();
D.SchemeColor schemeColor1 = new D.SchemeColor() { Val = D.SchemeColorValues.Text1 };
solidFill2.Append(schemeColor1);
outline1.Append(solidFill2);
shapeProperties1.Append(transform2D1);
shapeProperties1.Append(presetGeometry1);
shapeProperties1.Append(solidFill1);
shapeProperties1.Append(outline1);
的TextBody
定義文本框屬性,如列數,對齊方式,錨定等。
TextBody textBody1 = new TextBody();
D.BodyProperties bodyProperties1 = new D.BodyProperties() { RightToLeftColumns = false, Anchor = D.TextAnchoringTypeValues.Center };
D.ListStyle listStyle1 = new D.ListStyle();
D.Paragraph paragraph1 = new D.Paragraph();
D.ParagraphProperties paragraphProperties1 = new D.ParagraphProperties() { Alignment = D.TextAlignmentTypeValues.Center };
D.EndParagraphRunProperties endParagraphRunProperties1 = new D.EndParagraphRunProperties() { Language = "es-ES" };
paragraph1.Append(paragraphProperties1);
paragraph1.Append(endParagraphRunProperties1);
textBody1.Append(bodyProperties1);
textBody1.Append(listStyle1);
textBody1.Append(paragraph1);
NonVisualShapeProperties
定義非可視屬性,如Name和Id。
NonVisualShapeProperties nonVisualShapeProperties1 = new NonVisualShapeProperties();
NonVisualDrawingProperties nonVisualDrawingProperties1 = new NonVisualDrawingProperties() { Id = (UInt32Value)4U, Name = "1 Shape Name" };
NonVisualShapeDrawingProperties nonVisualShapeDrawingProperties1 = new NonVisualShapeDrawingProperties();
ApplicationNonVisualDrawingProperties applicationNonVisualDrawingProperties1 = new ApplicationNonVisualDrawingProperties();
nonVisualShapeProperties1.Append(nonVisualDrawingProperties1);
nonVisualShapeProperties1.Append(nonVisualShapeDrawingProperties1);
nonVisualShapeProperties1.Append(applicationNonVisualDrawingProperties1);
全部放在一起
最后,您必須創建形狀對象並將這些屬性附加到它:
Shape shape1 = new Shape();
shape1.Append(nonVisualShapeProperties1);
shape1.Append(shapeProperties1);
shape1.Append(shapeStyle1);
shape1.Append(textBody1);
然后,將形狀添加到幻燈片的形狀樹中:
s.CommonSlideData.ShapeTree.Append(shape1);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.