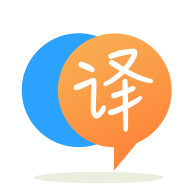
[英]Recursive function to reverse an array implementation of a queue in Java
[英]Queue implementation with Array- Java
我正在嘗試使用數組實現隊列。 (不使用內置的Java Queue函數)。 但是,在測試時,只有在size == maxSize時(如數組的大小達到maxSize / capacity),才會打印出數組。 當大小小於maxSize時,除了不打印以外,測試用例都通過了。 (這是一個雙端隊列,因此可以將元素添加到前面和后面)。 有什么建議嗎?
package vipQueueArray;
import java.util.NoSuchElementException;
public class vipQueue {
private Object[] array;
private int size = 0;
private int head = 0; // index of the current front item, if one exists
private int tail = 0; // index of next item to be added
private int maxSize;
public vipQueue(int capacity) {
array = new Object[capacity];
maxSize = capacity;
size = 0;
tail = maxSize - 1;
}
public Object Dequeue() {
if (size == 0) {
throw new NoSuchElementException("Cant remove: Empty");
}
Object item = array[head];
array[head] = null;
head = (head + 1) % array.length;
size--;
return item;
}
public void EnqueueVip(Object x) {
if (size == maxSize) {
throw new IllegalStateException("Cant add: Full");
}
array[tail] = x;
tail = (tail - 1) % array.length;
size++;
}
public void Enqueue(Object y) {
if (size == maxSize) {
throw new IllegalStateException("Cant add: Full");
}
array[head] = y;
head = (head + 1) % array.length;
size++;
}
public boolean isFull() {
return (size == maxSize);
}
public boolean isEmpty() {
return (size == 0);
}
}
public class Test{
public static void main(String[] args){
vipQueue Q = new vipQueue(2);
Q.Enqueue(4);
System.out.printf("->%d", Q.Dequeue());
} }
在出隊時,您正在執行以下操作:
head = (head + 1) % array.length;
您應該(根據您的暗示)將其更改為:
head = (head - 1) % array.length;
加法 :
這不是隊列的實現:隊列在FIFO中工作,而您實現的在LIFO中工作,實際上更像是...堆棧。
為了實現隊列,您應該從頭和尾都指向array [0]開始。 然后,每個插入都是addAtTail()
,這意味着該項目將在array [tail]中輸入:
array[tail] = item;
tail++;
size++;
當tail == array.length(拋出異常)時,您將停止插入。
出隊意味着刪除array[tail-1]
處的項目,然后執行以下操作:
tail--;
size--;
入隊時,設置head = head +1。出隊時,返回array [head]
go,入隊后,head = 1,但是您添加的項目在插槽0中。
此外,當tail = Capacity-1時,如果隊列中沒有任何內容,勢必會引起麻煩。 恩
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.