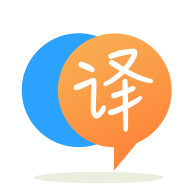
[英]Is there a cross-platform way of getting information from Python's OSError?
[英]Python: Getting AppData folder in a cross-platform way
我想要一個代碼片段,它可以在所有平台(至少是 Win/Mac/Linux)上獲取應用程序數據(配置文件等)的正確目錄。 例如:Windows 上的 %APPDATA%/。
如果您不介意使用appdirs 模塊,它應該可以解決您的問題。 (成本 = 您要么需要安裝模塊,要么直接將其包含在 Python 應用程序中。)
Qt 的QStandardPaths 文檔列出了這樣的路徑。
使用 Python 3.8
import sys
import pathlib
def get_datadir() -> pathlib.Path:
"""
Returns a parent directory path
where persistent application data can be stored.
# linux: ~/.local/share
# macOS: ~/Library/Application Support
# windows: C:/Users/<USER>/AppData/Roaming
"""
home = pathlib.Path.home()
if sys.platform == "win32":
return home / "AppData/Roaming"
elif sys.platform == "linux":
return home / ".local/share"
elif sys.platform == "darwin":
return home / "Library/Application Support"
# create your program's directory
my_datadir = get_datadir() / "program-name"
try:
my_datadir.mkdir(parents=True)
except FileExistsError:
pass
Python 文檔推薦使用sys.platform.startswith('linux')
"idiom" 以與返回 "linux2" 或 "linux3" 之類的舊版本 Python 兼容。
您可以使用以下函數獲取用戶數據目錄,在 linux 和 w10 中測試(返回AppData/Local
目錄)它改編自appdirs包:
import sys
from pathlib import Path
from os import getenv
def get_user_data_dir(appname):
if sys.platform == "win32":
import winreg
key = winreg.OpenKey(
winreg.HKEY_CURRENT_USER,
r"Software\Microsoft\Windows\CurrentVersion\Explorer\Shell Folders"
)
dir_,_ = winreg.QueryValueEx(key, "Local AppData")
ans = Path(dir_).resolve(strict=False)
elif sys.platform == 'darwin':
ans = Path('~/Library/Application Support/').expanduser()
else:
ans=Path(getenv('XDG_DATA_HOME', "~/.local/share")).expanduser()
return ans.joinpath(appname)
我建議在您要使用此程序的操作系統中研究“appdata”的位置。 一旦你知道了位置,你就可以簡單地使用 if 語句來檢測 os 和 do_something()。
import sys
if sys.platform == "platform_value":
do_something()
elif sys.platform == "platform_value":
do_something()
列表來自官方 Python 文檔。 (搜索“sys.platform”)
您可以使用名為appdata的模塊:
pip install appdata
from appdata import AppDataPaths
app_paths = AppDataPaths()
app_paths.app_data_path # cross-platform path to AppData folder
我遇到了一個類似的問題,我想動態地解決所有的 Windows % 路徑而不事先知道它們。 您可以使用os.path.expandvars
動態解析路徑。 像這樣的東西:
from os import path
appdatapath = '%APPDATA%\MyApp'
if '%' in appdatapath:
appdatapath = path.expandvars(appdatapath)
print(appdatapath)
最后的行將打印: C:\Users\\{user}\AppData\Roaming\MyApp
這適用於 Windows,但我尚未在 Linux 上進行測試。 只要路徑是由環境定義的,expandvars 就應該能夠找到它。 您可以在此處閱讀有關擴展變量的更多信息。
如果您在config目錄之后,這里是一個默認為~/.config
的解決方案,除非該方法的本地平台中提供了特定於平台的 ( sys.platform
) 條目:dict
from sys import platform
from os.path import expandvars, join
def platform_config_directory() -> str:
''' Platform config directory '''
home: str = expandvars('$HOME')
platforms: dict = {
"win32": expandvars('%AppData%'),
"darwin": join(home, 'Library', 'Application Support'),
}
if platform in platforms:
return platforms[platform]
return join(home, '.config')
這適用於 windows、mac 和 linux,但是可以根據需要更輕松地進行擴展。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.