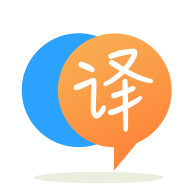
[英]JQuery Promise + Google map setting markers for locations from JSON is not working
[英]Connecting Json to Jquery to Add Markers in Google Map
有人可以幫我嗎,因為我陷入了需要循環json並將其作為標記添加到Google地圖的情況,但它只返回null
有一種方法可以自動將此連接到Json,因為我打算擁有一個Grid並將其設置為style="display: none;"
隱藏它,然后獲取並讀取網格中的值
這是我的Javascript
<script type="text/javascript">
var geocoder;
var map;
function initialize() {
var minZoomLevel = 4;
var zooms = 7;
geocoder = new google.maps.Geocoder();
map = new google.maps.Map(document.getElementById('map'), {
zoom: minZoomLevel,
center: new google.maps.LatLng(38.50, -90.50),
mapTypeId: google.maps.MapTypeId.ROADMAP
});
// Bounds for North America
var strictBounds = new google.maps.LatLngBounds(
new google.maps.LatLng(15.70, -160.50),
new google.maps.LatLng(68.85, -55.90)
);
// Listen for the dragend event
google.maps.event.addListener(map, 'dragend', function () {
if (strictBounds.contains(map.getCenter())) return;
// We're out of bounds - Move the map back within the bounds
var c = map.getCenter(),
x = c.lng(),
y = c.lat(),
maxX = strictBounds.getNorthEast().lng(),
maxY = strictBounds.getNorthEast().lat(),
minX = strictBounds.getSouthWest().lng(),
minY = strictBounds.getSouthWest().lat();
if (x < minX) x = minX;
if (x > maxX) x = maxX;
if (y < minY) y = minY;
if (y > maxY) y = maxY;
map.setCenter(new google.maps.LatLng(y, x));
});
// Limit the zoom level
google.maps.event.addListener(map, 'zoom_changed', function () {
if (map.getZoom() < minZoomLevel) map.setZoom(minZoomLevel);
});
}
function codeAddress() {
var address = $('#workerGrid').find("input[name=shiftDay]");
alert(address);
$.each(address, function () {
address.each(function (e) {
e.preventDefault();
geocoder.geocode({ 'address': address }, function (results, status) {
if (status == google.maps.GeocoderStatus.OK) {
map.setCenter(results[0].geometry.location);
var marker = new google.maps.Marker({
map: map,
position: results[0].geometry.location,
title: address
});
if (results[0].geometry.viewport) {
map.setZoom(10);
}
}
else {
alert("Geocode was not successful for the following reason: " + status);
}
});
});
});
}
window.onload = function () {
initialize();
codeAddress();
}
</script>
這是我的傑森(Json):
public JsonResult LoadWorkerList(string search)
{
var workerList = new List<Worker_Address>();
// check if search string has value
// retrieve list of workers filtered by search criteria
var list = (from a in db.Worker_Address
where a.LogicalDelete == false
select a).ToList();
List<WorkerAddressInfo> wlist = new List<WorkerAddressInfo>();
foreach (var row in list)
{
WorkerAddressInfo ci = new WorkerAddressInfo
{
ID = row.ID,
Worker_ID = row.WorkerID,
AddressLine1 = row.Address_Line1 + " " + row.Address_Line2+ " " +row.City + " "+ GetLookupDisplayValById(row.State_LookID),
//AddressLine2 = row.Address_Line2,
//City = row.City,
//State = GetLookupDisplayValById(row.State_LookID),
LogicalDelete = row.LogicalDelete
};
wlist.Add(ci);
}
var wolist = (from a in wlist
where a.LogicalDelete == false
select a).ToList();
wlist = wolist;
return Json(wlist.ToList().OrderBy(p => p.AddressLine1), JsonRequestBehavior.AllowGet);
}
希望可以有人幫幫我
我相信您可以用另一種方法來做到這一點:獲取地圖,進入config對象並在其中添加標記數組。
我上周工作的一個小例子(谷歌地圖v3):
proj.root.gmap3({
marker: {
values: markersList, // arrGeodesic
options: {
icon: new google.maps.MarkerImage(cfg.markers.custom),
draggable: false
}
}
);
我的標記數組是這樣填充的:
// 2.10 Get markers from ajax request
// @param (lat): float value of latitude
// @param (lng): float value of longitude
// @doc (ajax data): http://encosia.com/3-mistakes-to-avoid-when-using-jquery-with-aspnet-ajax/
// @doc (JSON.stringify): https://github.com/douglascrockford/JSON-js
ajaxGetMarkers: function (lat, lng) {
var proj = this,
json = {};
if (lat && lng) {
// param mapping
json.country = proj.countries.val();
json.postcode = proj.postcode.val();
json.latitude = lat.toFixed(12);
json.longitude = lng.toFixed(12);
// ajax request
$.ajax({
url: cfg.ajaxUrls.locations, // '/GMAPS/Locations.aspx/GetLocations'
data: JSON.stringify(json)
}).done(function (data) {
if (data && data.d) {
proj.root.gmap3("clear", "markers");
proj.loadMarkers(data.d.Locationslist);
}
});
}
},
希望它不會使事情復雜化,但這是我可以從您擁有的數據中提取LngLat對象的方法...在我的示例中,它具有國家和郵政編碼...我相信您可以使用您的數據實現相同的效果.. 。
// 2.9 Load map
// @param (query): array [country, postcode]
loadMap: function (query) {
var proj = this,
options, loc, geocoder;
// init
if (query.length) {
// 2.9.1 geocode
geocoder = new google.maps.Geocoder();
// 2.9.1 set address
geocoder.geocode({ address: query.join(" ") }, function (result, status) {
if (status === google.maps.GeocoderStatus.OK) {
// center map
loc = result[0].geometry.location;
options = $.extend({}, cfg.mapOptions, { center: loc });
// set map options & markers
proj.initMap(options);
proj.ajaxGetMarkers(loc.lat(), loc.lng());
}
});
}
},
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.