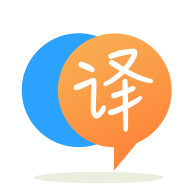
[英]How to make a xaml textbox in silverlight accept only numbers with maximum one decimal point precision
[英]How to make a textBox accept only Numbers and just one decimal point in Windows 8
我是 windows 8 電話的新手。 我正在編寫一個計算器應用程序,它只能接受文本框中的數字和一個小數點。 我如何防止用戶在文本框中輸入兩個或更多小數點,因為計算器無法處理。
我一直在使用 Keydown Event,這是最好的還是我應該使用 Key up?
private void textbox_KeyDown(object sender, System.Windows.Input.KeyEventArgs e) {
}
您還可以將RegExp與TextChanged
事件一起使用:下面的代碼段將處理任何整數和浮點數,包括正數和負數
string previousInput = "";
private void InputTextbox_TextChanged(object sender, RoutedEventArgs e)
{
Regex r = new Regex("^-{0,1}\d+\.{0,1}\d*$"); // This is the main part, can be altered to match any desired form or limitations
Match m = r.Match(InputTextbox.Text);
if (m.Success)
{
previousInput = InputTextbox.Text;
}
else
{
InputTextbox.Text = previousInput;
}
}
您可以將此用於KeyPress事件集按鍵事件,用於form.Designer中的文本框
this.yourtextbox.KeyPress +=new System.Windows.Forms.KeyPressEventHandler(yourtextbox_KeyPress);
然后在表單中使用它
//only number And single decimal point input فقط عدد و یک ممیز میتوان نوشت
public void onlynumwithsinglepoint(object sender, KeyPressEventArgs e)
{
if (!(char.IsDigit(e.KeyChar) || e.KeyChar == (char)Keys.Back || e.KeyChar == '.'))
{ e.Handled = true; }
TextBox txtDecimal = sender as TextBox;
if (e.KeyChar == '.' && txtDecimal.Text.Contains("."))
{
e.Handled = true;
}
}
然后
private void yourtextbox_KeyPress(object sender, KeyPressEventArgs e)
{
onlynumwithsinglepoint(sender, e);
}
我曾經在.NET 2天中廣泛使用它,不確定它是否仍然有效。 支持可配置的精度,貨幣,負數並處理所有輸入方法。
using System;
using System.ComponentModel;
using System.Collections;
using System.Diagnostics;
using System.Drawing;
using System.Security.Permissions;
using System.Text.RegularExpressions;
using System.Windows.Forms;
namespace System.Windows.Forms
{
public class NumericTextBox : TextBox
{
private System.ComponentModel.Container components = null;
private bool _AllowNegatives = true;
private int _Precision = 0;
private char _CurrencyChar = (char)0;
private bool _AutoFormat = true;
private decimal _MinValue = Decimal.MinValue;
private decimal _MaxValue = Decimal.MaxValue;
/// <summary>
/// Indicates if the negative values are allowed.
/// </summary>
[DefaultValue(true), RefreshProperties(RefreshProperties.All), Description("Indicates if the negative values are allowed."), Category("Behavior")]
public bool AllowNegatives
{
get
{
return this._AllowNegatives;
}
set
{
this._AllowNegatives = value;
if (!value)
{
if (this._MinValue < 0) this._MinValue = 0;
if (this._MaxValue < 1) this._MaxValue = 1;
}
}
}
/// <summary>
/// Indicates the maximum number of digits allowed after a decimal point.
/// </summary>
[DefaultValue(0), Description("Indicates the maximum number of digits allowed after a decimal point."), Category("Behavior")]
public int Precision
{
get
{
return this._Precision;
}
set
{
if (value < 0)
{
throw new ArgumentOutOfRangeException("Precision", value.ToString(), "The value of precision must be an integer greater than or equal to 0.");
}
else
{
this._Precision = value;
}
}
}
/// <summary>
/// Gets or sets the character to use as a currency symbol when validating input.
/// </summary>
[DefaultValue((char)0), Description("Gets or sets the character to use as a currency symbol when validating input."), Category("Behavior")]
public char CurrencyChar
{
get
{
return this._CurrencyChar;
}
set
{
this._CurrencyChar = value;
}
}
/// <summary>
/// Indicates if the text in the textbox is automatically formatted. Missing currency symbols and trailing spaces are added where applicable.
/// </summary>
[DefaultValue(true), Description("Indicates if the text in the textbox is automatically formatted. Missing currency symbols and trailing spaces are added where applicable."), Category("Behavior")]
public bool AutoFormat
{
get
{
return this._AutoFormat;
}
set
{
this._AutoFormat = value;
}
}
/// <summary>
/// Gets or sets the numerical value of the textbox.
/// </summary>
[Description("Gets or sets the numerical value of the textbox."), Category("Data")]
public decimal Value
{
get
{
if (this.Text.Length == 0)
{
return (decimal)0;
}
else
{
return decimal.Parse(this.Text.Replace(this.CurrencyChar.ToString(), ""));
}
}
set
{
if (value < 0 && !this.AllowNegatives)
{
throw new ArgumentException("The specified decimal is invalid, negative values are not permitted.");
}
this.Text = this.FormatText(value.ToString());
}
}
/// <summary>
/// Gets or sets the maximum numerical value of the textbox.
/// </summary>
[RefreshProperties(RefreshProperties.All), Description("Gets or sets the maximum numerical value of the textbox."), Category("Behavior")]
public decimal MaxValue
{
get
{
return this._MaxValue;
}
set
{
if (value <= this._MinValue)
{
throw new ArgumentException("The Maximum value must be greater than the minimum value.", "MaxValue");
}
else
{
this._MaxValue = Decimal.Round(value, this.Precision);
}
}
}
/// <summary>
/// Gets or sets the minimum numerical value of the textbox.
/// </summary>
[RefreshProperties(RefreshProperties.All), Description("Gets or sets the minimum numerical value of the textbox."), Category("Behavior")]
public decimal MinValue
{
get
{
return this._MinValue;
}
set
{
if (value < 0 && !this.AllowNegatives)
{
throw new ArgumentException("The Minimum value cannot be negative when AllowNegatives is set to false.", "MinValue");
}
else if (value >= this._MaxValue)
{
throw new ArgumentException("The Minimum value must be less than the Maximum value.", "MinValue");
}
else
{
this._MinValue = Decimal.Round(value, this.Precision);
}
}
}
/// <summary>
/// Create a new instance of the NumericTextBox class with the specified container.
/// </summary>
/// <param name="container">The container of the control.</param>
public NumericTextBox(System.ComponentModel.IContainer container)
{
///
/// Required for Windows.Forms Class Composition Designer support
///
container.Add(this);
InitializeComponent();
}
/// <summary>
/// Create a new instance of the NumericTextBox class.
/// </summary>
public NumericTextBox()
{
///
/// Required for Windows.Forms Class Composition Designer support
///
InitializeComponent();
}
#region Component Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
//
// NumericTextBox
//
this.KeyPress += new System.Windows.Forms.KeyPressEventHandler(this.NumericTextBox_KeyPress);
this.Validating += new System.ComponentModel.CancelEventHandler(this.NumericTextBox_Validating);
}
#endregion
/// <summary>
/// Checks to see if the specified text is valid for the properties selected.
/// </summary>
/// <param name="text">The text to test.</param>
/// <returns>A boolean value indicating if the specified text is valid.</returns>
protected bool IsValid(string text)
{
Regex check = new Regex("^" + ((this.AllowNegatives && this.MinValue < 0) ? (@"\-?") : "") + ((this.CurrencyChar != (char)0) ? (@"(" + Regex.Escape(this.CurrencyChar.ToString()) + ")?") : "") + @"\d*" + ((this.Precision > 0) ? (@"(\.\d{0," + this.Precision.ToString() + "})?") : "") + "$");
if (!check.IsMatch(text)) return false;
if (text == "-" || text == this.CurrencyChar.ToString() || text == "-" + this.CurrencyChar.ToString()) return true;
Decimal val = Decimal.Parse(text);
if (val < this.MinValue) return false;
if (val > this.MaxValue) return false;
return true;
}
/// <summary>
/// Formats the specified text into the configured number format.
/// </summary>
/// <param name="text">The text to format.</param>
/// <returns>The correctly formatted text.</returns>
protected string FormatText(string text)
{
string format = "{0:" + this.CurrencyChar.ToString() + "0" + ((this.Precision > 0) ? "." + new String(Convert.ToChar("0"), this.Precision) : "") + ";-" + this.CurrencyChar.ToString() + "0" + ((this.Precision > 0) ? "." + new String(Convert.ToChar("0"), this.Precision) : "") + "; £0" + ((this.Precision > 0) ? "." + new String(Convert.ToChar("0"), this.Precision) : "") + "}";
return String.Format(format, text).Trim();
}
/// <summary>
/// Overrides message handler in order to pre-process and validate pasted data.
/// </summary>
/// <param name="m">Message</param>
protected override void WndProc(ref Message m)
{
switch (m.Msg)
{
case 0x0302:
if (Clipboard.GetDataObject().GetDataPresent(DataFormats.Text))
{
string paste = Clipboard.GetDataObject().GetData(DataFormats.Text).ToString();
string text = this.Text.Substring(0, this.SelectionStart) + paste + this.Text.Substring(this.SelectionStart + this.SelectionLength);
if (this.IsValid(text))
{
base.WndProc(ref m);
}
}
break;
default:
base.WndProc(ref m);
break;
}
}
protected override void Dispose(bool disposing)
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void NumericTextBox_KeyPress(object sender, System.Windows.Forms.KeyPressEventArgs e)
{
if (Char.IsNumber(e.KeyChar) ||
(this.AllowNegatives && e.KeyChar == Convert.ToChar("-")) ||
(this.Precision > 0 && e.KeyChar == Convert.ToChar(".")) ||
e.KeyChar == this.CurrencyChar)
{
string text = this.Text.Substring(0, this.SelectionStart) + e.KeyChar.ToString() + this.Text.Substring(this.SelectionStart + this.SelectionLength);
if (!this.IsValid(text))
{
e.Handled = true;
}
}
else if (!Char.IsControl(e.KeyChar))
{
e.Handled = true;
}
}
private void NumericTextBox_Validating(object sender, System.ComponentModel.CancelEventArgs e)
{
if (this.Text.Length == 0)
{
this.Text = this.FormatText("0");
}
else if (this.IsValid(this.Text))
{
this.Text = this.FormatText(this.Text);
}
else
{
e.Cancel = true;
}
}
}
}
簡單
private void textBox1_KeyPress(object sender, KeyPressEventArgs e)
{
char ch = e.KeyChar;
decimal x;
if (ch == (char)Keys.Back)
{
e.Handled = false;
}
else if (!char.IsDigit(ch) && ch != '.' || !Decimal.TryParse(textBox1.Text+ch, out x) )
{
e.Handled = true;
}
}
private void txtBox_KeyPress(object sender, KeyPressEventArgs e)
{
if (Char.IsDigit(e.KeyChar) || e.KeyChar == '\b')
{
// Allow Digits and BackSpace char
}
else if (e.KeyChar == '.' && !((TextBox)sender).Text.Contains('.'))
{
//Allows only one Dot Char
}
else
{
e.Handled = true;
}
}
您可以使用數字文本框或
嘗試這個
private void textBox1_KeyPress(object sender, KeyPressEventArgs e)
{
if (Char.IsDigit(e.KeyChar) || e.KeyChar == '\b')
{
e.Handled = false;
}
else
{
e.Handled = true;
}
}
對於數字==> ImputScope ="Number"
您可以使用解析例子來計算字符串中的字符點,例如dat:
char[] c = yourstring.tocharArray();
int i = 0;
foreach var char in c
if(char.equals(".") or char.equals(",")
i++
if (i > 1)
MessageBox.Show("Invalid Number")
這段代碼只是舉例,但它的工作原理。
謝謝大家的幫助。 我終於做到了這一點,它在Text change事件中運行得非常好。 那是在將輸入鏡設置為Numbers之后
string dika = Textbox1.Text;
int countDot = 0;
for (int i = 0; i < dika.Length; i++)
{
if (dika[i] == '.')
{
countDot++;
if (countDot > 1)
{
dika = dika.Remove(i, 1);
i--;
countDot--;
}
}
}
Textbox1.Text = dika;
Textbox1.Select(dika.Text.Length, 0);
private void txtDecimal_KeyPress(object sender, KeyPressEventArgs e)
{
if (!Char.IsDigit(e.KeyChar) && e.KeyChar != '\b' && e.KeyChar!='.')
{
e.Handled = true;
}
if (e.KeyChar == '.' && txtDecimal.Text.Contains("."))
{
e.Handled = true;
}
}
看這個。 這項工作對我來說。
private void txtParciales_KeyDown(object sender, System.Windows.Input.KeyEventArgs e)
{
if (System.Text.RegularExpressions.Regex.IsMatch(txtParciales.Text, @"\d+(\.\d{2,2})"))
e.Handled = true;
else e.Handled = false;
}
這就是我用過的東西。
private void txtBoxBA_KeyDown(object sender, KeyRoutedEventArgs e)
{
// only allow 0-9 and "."
e.Handled = !((e.Key.GetHashCode() >= 48 && e.Key.GetHashCode() <= 57));
// check if "." is already there in box.
if (e.Key.GetHashCode() == 190)
e.Handled = (sender as TextBox).Text.Contains(".");
}
嘗試使用asp:RegularExpressionValidator
控制器
<asp:RegularExpressionValidator ID="rgx"
ValidationExpression="[0-9]*\.?[0-9]" ControlToValidate="YourTextBox" runat="server" ForeColor="Red" ErrorMessage="Decimals only!!" Display="Dynamic" ValidationGroup="lnkSave"></asp:RegularExpressionValidator>
private void textBox1_KeyPress(object sender, KeyPressEventArgs e)
{
if (e.KeyChar != Convert.ToChar(Keys.Enter) && e.KeyChar != Convert.ToChar(Keys.Back) && e.KeyChar != Convert.ToChar(Keys.Delete) && e.KeyChar != '.' && e.KeyChar != '1' && e.KeyChar != '2' && e.KeyChar != '3' && e.KeyChar != '4' && e.KeyChar != '5' && e.KeyChar != '6' && e.KeyChar != '7' && e.KeyChar != '8' && e.KeyChar != '9' && e.KeyChar != '0')
{
e.Handled = true;
}
else
{
e.Handled = false;
}
}
驗證所需列表
Keypress事件
private void txtEstimate_KeyPress(object sender, KeyPressEventArgs e)
{
if (e.KeyChar == '.' && txtEstimate.Text.Contains("."))
{
// Stop more than one dot Char
e.Handled = true;
}
else if (e.KeyChar == '.' && txtEstimate.Text.Length == 0)
{
// Stop first char as a dot input
e.Handled = true;
}
else if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && e.KeyChar != '.')
{
// Stop allow other than digit and control
e.Handled = true;
}
}
低於輸入驗證
它適用於 KeyPress
private void textBox1_KeyPress(object sender, KeyPressEventArgs e)
{
if ((!char.IsNumber(e.KeyChar) && e.KeyChar != '.'
&& e.KeyChar != (char)Keys.Back)
|| (e.KeyChar == '.' && textBox1.Text.Contains('.')))
{
e.Handled = true;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.