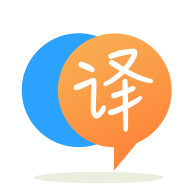
[英]while running an android application, i am getting the following error in console
[英]i am using http post method to update the database in android application but i am getting the following error
11-10 13:32:47.398:E / AndroidRuntime(2155):致命異常:主11-10 13:32:47.398:E / AndroidRuntime(2155):android.os.NetworkOnMainThreadException 11-10 13:32:47.398: E / AndroidRuntime(2155):位於android.os.StrictMode $ AndroidBlockGuardPolicy.onNetwork(StrictMode.java:1117)11-10 13:32:47.398:E / AndroidRuntime(2155):位於java.net.InetAddress.lookupHostByName(InetAddress .java:385)11-10 13:32:47.398:E / AndroidRuntime(2155):在java.net.InetAddress.getAllByNameImpl(InetAddress.java:236)11-10 13:32:47.398:E / AndroidRuntime(2155) ):位於java.net.InetAddress.getAllByName(InetAddress.java:214)
公共類UpdatingDatabase上的錯誤擴展了AsyncTask
package com.nikhil.svs;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import android.os.AsyncTask;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
public class Register extends MainActivity {
EditText reg,pwd;
@Override
protected void onCreate(Bundle savedInstanceState) {
// TODO Auto-generated method stub
super.onCreate(savedInstanceState);
setContentView(R.layout.register);
reg=(EditText) findViewById(R.id.editusername);
pwd=(EditText) findViewById(R.id.editpassword);
Button register=(Button) findViewById(R.id.register);
register.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
new UpdatingDatabase().execute();
}
public class UpdatingDatabase extends AsyncTask<String, String, String>
{
protected String doInBackground(String... arg0) { //add this line
HttpClient httpclient = new DefaultHttpClient();
HttpPost httppost = new HttpPost("http://nikhilvaddepati.tk.hostinghood.com/test.php");
String a=reg.getText().toString();
String b=pwd.getText().toString();
try {
// Add your data
List<NameValuePair> nameValuePairs = new ArrayList<NameValuePair>(2);
nameValuePairs.add(new BasicNameValuePair("regno", ""+a));
nameValuePairs.add(new BasicNameValuePair("pwd",""+b));
httppost.setEntity((HttpEntity) new UrlEncodedFormEntity(nameValuePairs));
// Execute HTTP Post Request
HttpResponse response = httpclient.execute(httppost);
}catch (ClientProtocolException e) {
// TODO Auto-generated catch block
} catch (IOException e) {
// TODO Auto-generated catch block
}
}
public void execute() {
// TODO Auto-generated method stub
}
}// and this brackets
});
}
}
如我在評論中所述,您需要使用線程來獲得網絡,並確保您不對UI Thread進行任何網絡訪問,而要在異步任務中執行
您必須將onClick按鈕中的代碼移動到AsynTask ...
它應該如下所示。
編輯!
public void onClick(View v) {
new UpdatingDatabase().execute();
}
public class UpdatingDatabase extends AsyncTask<String, String, String>
{
protected String doInBackground(String... arg0) { //add this line
HttpClient httpclient = new DefaultHttpClient();
HttpPost httppost = new HttpPost("http://nikhilvaddepati.tk.hostinghood.com/test.php");
String a=reg.getText().toString();
String b=pwd.getText().toString();
try {
// Add your data
List<NameValuePair> nameValuePairs = new ArrayList<NameValuePair>(2);
nameValuePairs.add(new BasicNameValuePair("regno", ""+a));
nameValuePairs.add(new BasicNameValuePair("pwd",""+b));
httppost.setEntity((HttpEntity) new UrlEncodedFormEntity(nameValuePairs));
// Execute HTTP Post Request
HttpResponse response = httpclient.execute(httppost);
}catch (ClientProtocolException e) {
// TODO Auto-generated catch block
} catch (IOException e) {
// TODO Auto-generated catch block
}
}
}// and this brackets
}
現在應該是這樣,它將解決您的錯誤。 請通知我是否可行
編輯:
final EditText reg, pwd;
protected void onCreate(Bundle savedInstanceState) {
// TODO Auto-generated method stub
super.onCreate(savedInstanceState);
setContentView(R.layout.register);
reg=(EditText) findViewById(R.id.editusername);
pwd=(EditText) findViewById(R.id.editpassword);
...// complete with the code
編輯2:
package com.nikhil.svs;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import android.os.AsyncTask;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
public class Register extends MainActivity {
EditText reg,pwd;
@Override
protected void onCreate(Bundle savedInstanceState) {
// TODO Auto-generated method stub
super.onCreate(savedInstanceState);
setContentView(R.layout.register);
reg=(EditText) findViewById(R.id.editusername);
pwd=(EditText) findViewById(R.id.editpassword);
Button register=(Button) findViewById(R.id.register);
register.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
new UpdatingDatabase().execute();
}
});
}
public class UpdatingDatabase extends AsyncTask<String, String, String>
{
protected String doInBackground(String... arg0) { //add this line
HttpClient httpclient = new DefaultHttpClient();
HttpPost httppost = new HttpPost("http://nikhilvaddepati.tk.hostinghood.com/test.php");
String a=reg.getText().toString();
String b=pwd.getText().toString();
try {
// Add your data
List<NameValuePair> nameValuePairs = new ArrayList<NameValuePair>(2);
nameValuePairs.add(new BasicNameValuePair("regno", ""+a));
nameValuePairs.add(new BasicNameValuePair("pwd",""+b));
httppost.setEntity((HttpEntity) new UrlEncodedFormEntity(nameValuePairs));
// Execute HTTP Post Request
HttpResponse response = httpclient.execute(httppost);
}catch (ClientProtocolException e) {
// TODO Auto-generated catch block
} catch (IOException e) {
// TODO Auto-generated catch block
}
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.