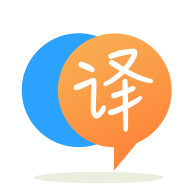
[英]How can I use a class and constructor in a another class, method and store them to my list? C#
[英]How can i store my List<List<Class>> to a text file using C# in WPF?
我正在嘗試使用C#將List<List<Class>>
到文本文件中,我的類就像
public class cEspecie
{
private string nombre;
private BitmapImage imagen;
private int tiempodevida;
private int movilidad;
private int decaimiento;
private int tipo;
private string colordelagrafica;
private bool seleccion;
public int Tipo
{
get
{
return tipo;
}
set
{
tipo = value;
}
}
public string Nombre
{
get
{
return nombre;
}
set
{
nombre = value;
}
}
public BitmapImage Imagen
{
get
{
return imagen;
}
set
{
imagen = value;
}
}
public int TiempoDeVida
{
get
{
return tiempodevida;
}
set
{
tiempodevida = value;
}
}
public int Movilidad
{
get
{
return movilidad;
}
set
{
movilidad = value;
}
}
public int Decaimiento
{
get
{
return decaimiento;
}
set
{
decaimiento = value;
}
}
public string ColorDeLaGrafica
{
get
{
return colordelagrafica;
}
set
{
colordelagrafica = value;
}
}
public bool Seleccion
{
get
{
return seleccion;
}
set
{
seleccion = value;
}
}
}
List<List<cEspecie>> items1 = new List<List<cEspecie>>();
我如何從“ items1”中獲取所有數據,以便使用C#將其存儲在文本文件中? 以及如何將其重新存儲以存儲在另一個列表中?
我能想到的最簡單的方法是實際上使用Xaml保存數據。 因為您已經在使用WPF,所以它是一個合適的解決方案。
簡單的例子:
List<List<cEspecie>> items1 = new List<List<cEspecie>>();
// ... add items...
XamlServices.Save(@"c:\path\to\file.xaml", items1);
然后加載:
var items2 = XamlServices.Load(@"c:\path\to\file.xaml");
請注意,要使此方法起作用,您需要將Imagen
從BitmapImage
更改為其基類BitmapSource
。 僅當圖像加載了URI或文件路徑時,此方法才有效,並且將使用該URI /路徑保存該圖像。 如果要防止將Imagen
屬性保存在Xaml中,則可以使用[DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)]
注釋該屬性。
這是我的測試代碼創建的Xaml文件:
<List x:TypeArguments="List(w:cEspecie)"
Capacity="4"
xmlns="clr-namespace:System.Collections.Generic;assembly=mscorlib"
xmlns:w="clr-namespace:WpfXUnit;assembly=WpfXUnit"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<List x:TypeArguments="w:cEspecie"
Capacity="4">
<w:cEspecie ColorDeLaGrafica="zomg"
Decaimiento="42"
Imagen="file:///w:/dump/test.png"
Movilidad="76"
Nombre="'ello gov'na"
Seleccion="True"
TiempoDeVida="32"
Tipo="1" />
</List>
</List>
我會考慮使用XML或JSON對數據進行序列化/反序列化。 這樣,您可以輕松地將數據存儲在文件中,通過Web服務發送等。
使用Json.NET會很簡單,唯一的問題是存儲位圖。
string json = JsonConvert.SerializeObject(items1);
根據您決定存儲位圖的方式,Json.NET無法將其存儲為JSON,除非您做了一些魔術。
最簡單的方法是不序列化Bitmap屬性,而是在反序列化字符串時加載Image。
這個 stackoverflow帖子中提供了一個簡單的解決方案。
編輯:
這是一個基於上面的stackoverflow帖子的示例,使用您的代碼。
[JsonObject(MemberSerialization.OptIn)]
public class Especie
{
private string m_imagePath;
[JsonProperty]
public Tipo { get; set; }
[JsonProperty]
public string Nombre { get; set; }
[JsonProperty]
public int TiempoDeVida { get; set; }
[JsonProperty]
public int Movilidad { get; set; }
[JsonProperty]
public int Decaimiento { get; set; }
[JsonProperty]
public string ColorDeLaGrafica { get; set; }
[JsonProperty]
public bool Seleccion { get; set; }
// not serialized because mode is opt-in
public Bitmap Imagen { get; private set; }
[JsonProperty]
public string ImagePath
{
get { return m_imagePath; }
set
{
m_imagePath = value;
Imagen = Bitmap.FromFile(m_imagePath);
}
}
}
如果您只擁有基本類型,則只需在類上放置[Serializable]
屬性即可,您可以使用XmlSeralizer
將其寫出到文件中。
[Serializable]
public class cEspecie
{
//...Snip...
}
但是,要正常工作,類中的所有元素也必須標記為[Serializable]
,不幸的是BitmapImage
沒有標記。
要解決此問題,我們標記了無法序列化的對象,將其跳過,然后提供一個可以序列化的新屬性,以表示圖像
[Serializable]
public class cEspecie
{
//...Snip...
[XmlIgnore] //Skip this object
public BitmapImage Imagen
{
get { return imagen; }
set { imagen = value; }
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] //This creates a secret hidden property that does not show up in intellisense
[XmlElement("Imagen")] //It uses the name of the real property for it's name
public BitmapImageSearalizer ImagenSerialized
{
get
{
return new BitmapImageSearalizer(imagen);
}
set
{
imagen = value.GetSearalizedImage();
}
}
}
[Serializable]
internal BitmapImageSearalizer
{
public BitmapImageSearalizer(BitmapImage sourceImage)
{
//...Snip...
}
public BitmapImage GetSearalizedImage()
{
//...Snip...
}
//...Snip...
}
我把它留給你寫BitmapImageSearalizer
我還沒有與這個類一起寫它。
為了向您展示如何做的更完整的已知工作示例,這是我的一個項目的摘錄,其中我僅用一個簡單的Bitmap
對象就完成了這一絕招
/// <summary>
/// 1 bit per pixel bitmap to use as the image source.
/// </summary>
[XmlIgnore]
public Bitmap BitmapImage
{
get { return _Bitmap; }
set
{
if (value.PixelFormat != System.Drawing.Imaging.PixelFormat.Format1bppIndexed)
throw new FormatException("Bitmap Must be in PixelFormat.Format1bppIndexed");
_Bitmap = value;
}
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)]
[XmlElement("Bitmap")]
public byte[] BitmapSerialized
{
get
{ // serialize
if (BitmapImage == null) return null;
using (MemoryStream ms = new MemoryStream())
{
BitmapImage.Save(ms, ImageFormat.Bmp);
return ms.ToArray();
}
}
set
{ // deserialize
if (value == null)
{
BitmapImage = null;
}
else
{
using (MemoryStream ms = new MemoryStream(value))
{
BitmapImage = new Bitmap(ms);
}
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.