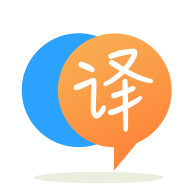
[英]In Python, how can I compare a numpy array to each row of a matrix to chose the row that is most similar to the vector?
[英]How can I apply a matrix transform to each row of a NumPy array efficiently?
假設我有一個2d NumPy ndarray,就像這樣:
[[ 0, 1, 2, 3 ],
[ 4, 5, 6, 7 ],
[ 8, 9, 10, 11 ]]
從概念上講,我想要做的是:
For each row:
Transpose the row
Multiply the transposed row by a transformation matrix
Transpose the result
Store the result in the original ndarray, overwriting the original row data
我有一個極其緩慢,強力的方法,在功能上實現了這一點:
import numpy as np
transform_matrix = np.matrix( /* 4x4 matrix setup clipped for brevity */ )
for i, row in enumerate( data ):
tr = row.reshape( ( 4, 1 ) )
new_row = np.dot( transform_matrix, tr )
data[i] = new_row.reshape( ( 1, 4 ) )
然而,這似乎是NumPy應該做的那種操作。 我認為 - 作為NumPy的新手 - 我只是遺漏了文檔中的一些基本內容。 有什么指針嗎?
請注意,如果創建新的ndarray更快,而不是就地編輯它,那么這也適用於我正在做的事情; 操作速度是首要關注的問題。
您要執行的一系列操作等同於以下內容:
data[:] = data.dot(transform_matrix.T)
或使用新數組而不是修改原始數據,這應該更快一點:
data.dot(transform_matrix.T)
這是解釋:
For each row:
Transpose the row
相當於轉置矩陣然后越過列。
Multiply the transposed row by a transformation matrix
將矩陣的每列左乘第二矩陣相當於將整個事物左乘第二矩陣。 此時,你擁有的是transform_matrix.dot(data.T)
Transpose the result
矩陣轉置的基本屬性之一是transform_matrix.dot(data.T).T
等同於data.dot(transform_matrix.T)
。
Store the result in the original ndarray, overwriting the original row data
切片分配執行此操作。
看來你需要轉置運算符 :
>>> np.random.seed(11)
>>> transform_matrix = np.random.randint(1, 10, (4,4))
>>> np.dot(transform_matrix, data.T).T
matrix([[ 24, 24, 17, 37],
[ 76, 108, 61, 137],
[128, 192, 105, 237]])
或等效地,如(A * B).T =(BT * AT):
>>> np.dot(data, transform_matrix.T)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.