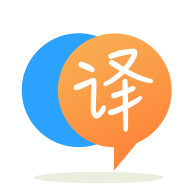
[英]How do I make a timer that when it is finished, it calls a function in JavaScript?
[英]How can I make this JavaScript Timer function properly?
我正在嘗試使用JavaScript制作計時器。 問題是,我無法讓計時器在達到0時停止運行。我嘗試使用return
和if
語句,但是似乎沒有任何作用。 我是在正確的軌道上,還是有更好的方法來做到這一點?
<input type="text" id="minutes" style="width:30px;text-align:center" maxlength="2" placeholder="00">
<span>:</span>
<input type="text" id="seconds" style="width:30px;text-align:center" maxlength="2" placeholder="00">
<button onclick="timer()">Set</button>
<script>
//This prototype correctly uses the modulus function when dealing with negative numbers.
Number.prototype.mod = function (m) {
return ((this % m) + m) % m
}
function timer() {
var minutes = document.getElementById('minutes').value //Value of minutes box.
var seconds = document.getElementById('seconds').value //Value of seconds box.
var initial = seconds * 1000 //Amount of seconds (converted to milliseconds) initially set.
var milliseconds = (minutes * 60000) + (seconds * 1000) //Total amount of milliseconds set.
setTimeout(function () {
alert("Time's up!")
}, milliseconds) //Display a message when the set time is up.
/*\Decreases the minute by one after the initially set amount of seconds have passed.
|*|Then, clears the interval and sets a new one to decrease the minute every 60 seconds.
\*/
test = setInterval(function () {
minutes--;
document.getElementById('minutes').value = minutes;
clearInterval(test)
setInterval(function () {
minutes--;
document.getElementById('minutes').value = minutes
}, 60000)
}, initial)
//Seconds are set to decrease by one every 1000 milliseconds, then be displayed in the seconds box.
setInterval(function () {
seconds--;
document.getElementById('seconds').value = seconds.mod(60)
}, 1000)
}
當您只需要一個時,便具有四個不同的計時器函數(setTimer和兩個setIntervals)。 的jsfiddle
function timer() {
var minutes = document.getElementById('minutes').value //Value of minutes box.
var seconds = document.getElementById('seconds').value //Value of seconds box.
var intervalTimer = setInterval(function () {
seconds--;
if (seconds < 0) {
seconds = 59;
minutes--;
}
document.getElementById('minutes').value = minutes;
document.getElementById('seconds').value = seconds;
if (minutes == 0 && seconds == 0) {
alert("Time's up!");
clearInterval(intervalTimer);
}
}, 1000);
}
通常,您需要確保為每個setInterval
都指定一個名稱( var x = setInterval...
),然后在以后將其清除( clearInterval(x)
)。 如果確實出於某種原因,您可以設置單獨的計時器(一個計時器在給定的秒數后開始,然后每60秒重復一次,分鍾,一個顯示消息),只要您清除所有計時器倒數到零時間隔計時器的間隔。
但是,使用兩個計時器可能很有意義。 這樣可以確保即使在間隔計時器中不精確的情況下,也可以在正確的時間顯示“時間到了”消息。
function timer() {
var minutes = document.getElementById('minutes').value,
seconds = document.getElementById('seconds').value,
intervalTimer = setInterval(function () {
seconds--;
if (seconds < 0) {
seconds = 59;
minutes--;
}
document.getElementById('minutes').value = minutes;
document.getElementById('seconds').value = seconds;
}, 1000);
setTimer(function () {
alert("Time's up!");
clearInterval(intervalTimer);
}, minutes * 60000 + seconds * 1000);
}
我對Stuart的答案進行了改進: 提琴
除了可以正常工作外,基本上是同一件事:
function clearTimer() {
clearInterval(intervalTimer);
}
var intervalTimer;
function timer() {
var minutes = document.getElementById('minutes').value //Value of minutes box.
var seconds = document.getElementById('seconds').value //Value of seconds box.
intervalTimer = setInterval(function () {
seconds--;
if (seconds < 0) {
seconds += 60;
minutes--;
}
if (minutes < 0) {
alert("Time's up!");
clearTimer();
} else {
document.getElementById('minutes').value = minutes;
document.getElementById('seconds').value = seconds;
}
}, 1000);
}
我不是一個很棒的javascript人,但這也許會有所幫助。 我用打字稿http://www.typescriptlang.org/Playground/
但我會做不同的計時,並使用javascript日期對象並計算差異。 這是我將如何開始創建時間的簡單示例(沒有日期對象)
JavaScript的
var Timer = (function () {
function Timer(time) {
this.accuracy = 1000;
this.time = time;
}
Timer.prototype.run = function (button) {
var _this = this;
this.time -= 1; //this is inaccurate, for accurate time use the date objects and calculate the difference.
//http://www.w3schools.com/js/js_obj_date.asp
button.textContent = this.time.toString();
if (this.time > 0) {
setTimeout(function () {
return _this.run(button);
}, 1000);
}
};
return Timer;
})();
var time = new Timer(10);
var button = document.createElement('button');
time.run(button);
document.body.appendChild(button);
打字稿(以防萬一)
class Timer {
accuracy = 1000;//update every second
time: number;
constructor(time: number) {
this.time = time;
}
run(button: HTMLButtonElement) {
this.time -=1;//this is inaccurate, for accurate time use the date objects and calculate the difference.
//http://www.w3schools.com/js/js_obj_date.asp
button.textContent = this.time.toString();
if(this.time > 0)
{
setTimeout(()=> this.run(button),1000);
}
}
}
var time = new Timer(10)
var button = document.createElement('button');
time.run(button);
document.body.appendChild(button);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.