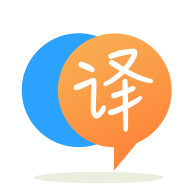
[英]How do I display the contents of an array without having the “0”'s in the display using a foreach loop?
[英]Using a FOR LOOP, how do I display top 3 value in an array?
使用For Loop
,如何顯示數組中的前3個值?
例如,我的數組大小為10,並且數組中列出了一些值,我想使用一個for循環提取前3個數據並在消息框中顯示。
//Loop to find Highest Qty of Fish Sold
for (int i = 1; i < fish_name.Length; i++)
{
if (sales_qty[i] > max_value1)
{
max_value1 = sales_qty[i];
max_index1 = i;
}
if (sales_qty[max_index1] > max_value2 && sales_qty[i] != max_value1)
{
max_value2 = sales_qty[i];
max_index2 = i;
}
if (sales_qty[max_index2] > max_value3 && sales_qty[i] != max_value1 && sales_qty[i] != max_value2)
{
max_value3 = sales_qty[i];
max_index3 = i;
}
}
我應該在loop
使用3 max index
嗎?
更新
目前我想到的是:
Array.Sort(sales_qty, fish_name);
Array.Reverse(sales_qty);
Array.Reverse(fish_name);
MessageBox.Show("The Top Three Best Selling Fish is: "+ "\n"
+ "1. "
+ fish_name[0]
+ "-"
+ sales_qty[0]
+ "\n"
+ "2. "
+ fish_name[1]
+ "-"
+ sales_qty[1]
+ "\n"
+ "3. "
+ fish_name[2]
+ "-"
+ sales_qty[2]
);
簡單的事情怎么樣
double[] sales_qty = new double[10] {1,2,3,4,5,6,7,8,9,10};
var result = sales_qty.OrderByDescending(x => x).Take(3);
如果您對最高值的索引感興趣,請嘗試
double[] sales_qty = new double[10] {1,2,3,4,5,6,7,8,9,10};
var result = sales_qty.Select((x, i) => new
{
Index = i,
Value = x
}).OrderByDescending(x => x.Value).Take(3);
創建第二個數組,在遍歷的同時,比較所有對象並找到前3個對象,將它們放在第二個數組中,完成后在消息框中打印這些對象。
或對數組排序並挑選出第三個對象。
您可以這樣做:使用Linq將是理想且容易的
using System;
using System.Linq;
public class Test
{
static void Main()
{
int[] array = new int[7] { 1, 3, 5, 2, 8, 6, 4 };
var topThree = (from i in array
orderby i descending
select i).Take(3);
foreach (var x in topThree)
{
Console.WriteLine(x);
}
}
}
希望能幫助到你..
首先,正如其他人所說,學習,熱愛並使用LINQ 。 這將使您的生活更輕松。
要回答這個問題:您似乎沒有一個一致的數組,而是兩個一致的數組,一個數組包含已售出的數量,另一個數組具有魚的名稱。 讓我們開始使用LINQ Zip運算符組合數據:
var zipped = fish_name.Zip(sales_qty, (Name, Qty) => new {Name, Qty});
通過此語句,我們正在組合(壓縮)兩個一致的數組。 兩個數組(名稱和數量)中的每個項目都使用lambda表達式組合到一個具有2個屬性的(匿名)對象。
現在,這變得更容易使用。 例如,您可以按數量輕松地排序此列表,並采用前三個元素:
var result = zipped.OrderByDescending(item => item.Qty).Take(3);
現在,您可以使用LINQ Select運算符格式化每個項目,從而將列表轉換為字符串列表:
var formattedItems = result.Select((item, index) =>
string.Format("{0}. {1}-{2}", index, item.Name, item.Qty);
然后使用string.Join格式化您的消息:
var message = "The Top Three Best Selling Fish is: " +
Environment.NewLine +
string.Join(Environment.NewLine, formattedItems);
MessageBox.Show(message);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.