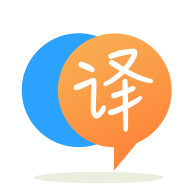
[英]How can I pass textbox value from usercontrol(ascx) to another page (aspx) using Server.Transfer()
[英]How To Pass Textbox Value Present In UserControl To Aspx Page Label By Clicking Button In UserControl
TestUC.ascx 設計代碼
<asp:TextBox ID="txtbox1" runat="server" ClientIDMode="Static" placeholder="Enter Some Text" ></asp:TextBox><br />
<asp:Button ID="btn1" runat="server" Text="Click" OnClick="btn1_Click" ClientIDMode="Static" />
Test.aspx 頁面代碼
<%@ Register Src="~/WebUserControls/TestUC.ascx" TagName="WebUserControlTest"
TagPrefix="uctest" %>
<asp:Content ID="Content1" ContentPlaceHolderID="cphBody" runat="server">
<asp:Label ID="lbl1" runat="server" >Label</asp:Label>
<uctest:WebUserControlTest ID="ucTest" runat="server"></uctest:WebUserControlTest>
</asp:Content>
輸出:
我需要..
步驟 1:在文本框中輸入一些文本
Step2:然后我點擊點擊按鈕【注意:這兩個控件是從UserControl綁定的】
Step3:在TextBox中輸入的文字顯示在標簽中[注意標簽出現在Aspx頁面]
您將需要一個自定義事件,並且您還需要像這樣在 UserControl 中公開TextBox
的Text
屬性。
public partial class YourUserControl : UserControl
{
public String Text
{
get
{
return this.txtBox1.Text;
}
//write the setter property if you would like to set the text
//of the TextBox from your aspx page
//set
//{
// this.txtBox1.Text = value;
//}
}
public delegate void TextAppliedEventHandler(Object sender, EventArgs e);
public event TextAppliedEventHandler TextApplied;
protected virtual void OnTextApplied(EventArgs e)
{
//Taking a local copy of the event,
//as events can be subscribed/unsubscribed asynchronously.
//If that happens after the below null check then
//NullReferenceException will be thrown
TextAppliedEventHandler handler = TextApplied;
//Checking if the event has been subscribed or not...
if (handler != null)
handler(this, e);
}
protected void yourUserControlButton_Click(Object sender, EventArgs e)
{
OnTextApplied(EventArgs.Empty);
}
}
然后在你的 aspx 頁面中,你放置了YourUserControl
(或者你從后面的代碼動態添加它),你可以像這樣訂閱這個事件。
protected void Page_Load(Object sender, EventArgs e)
{
if (!IsPostBack)
{
yourUserControl.TextApplied += new YourUserControl.TextAppliedEventHandler(yourUserControl_TextApplied)
}
}
您可以像這樣在頁面中使用用戶控件的自定義事件。
protected void yourUserControl_TextApplied(Object sender, EventArgs e)
{
yourLabelInYourPage.Text = yourUserControl.Text;
}
你已經完成了......
編輯:您可以根據需要重命名控件和事件。 我僅將名稱用於示例目的。
編輯:在網站項目中,如果要動態添加用戶控件,則可能需要在頁面中包含命名空間ASP
,如下所示。
using ASP;
並在您的頁面中的 aspx 標記中添加此Directive
。
<%@ Reference Control="~/PathToYourUserControl/YourUserControl.ascx" %>
其他解決方案:在用戶控件中創建一個事件,該事件在按鈕單擊中調用。
在 aspx 頁面的代碼隱藏中訂閱此事件。 這樣,您只能在提供值時更新您的界面。
稍微復雜一點,但您可以在將來將此邏輯重新用於更復雜的控制/父控制功能。
如果被問到,我可以添加代碼片段
這個答案是在@Devraj Gadhavi 的幫助下准備的,我編輯了一些代碼。
用戶控件頁面設計代碼
<asp:TextBox ID="txtbox1" runat="server" ClientIDMode="Static" placeholder="Enter Some Text" ></asp:TextBox><br />
<asp:Button ID="btn1" runat="server" Text="Click" OnClick="btn1_Click" ClientIDMode="Static" />
用戶控制頁面代碼
public partial class TestUC : System.Web.UI.UserControl
{
public String Text
{
get
{
return this.txtbox1.Text;
}
}
public delegate void TextAppliedEventHandler(Object sender, EventArgs e);
public event EventHandler TextApplied;
protected virtual void OnTextApplied(EventArgs e)
{
if (TextApplied != null)
TextApplied(this, e);
}
protected void btn1_Click(object sender, EventArgs e)
{
OnTextApplied(EventArgs.Empty);
}
}
Aspx 頁面設計代碼
<%@ Register Src="~/WebUserControls/TestUC.ascx" TagName="WebUserControlTest"
TagPrefix="uctest" %>
<asp:Content ID="Content1" ContentPlaceHolderID="cphBody" runat="server">
<asp:Label ID="lbl1" runat="server" >Label</asp:Label>
<uctest:WebUserControlTest ID="ucTest" runat="server"></uctest:WebUserControlTest>
</asp:Content>
Aspx Cs 文件代碼
public partial class Test2 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
ucTest.TextApplied += new EventHandler(ucTest_TextApplied);
}
protected void ucTest_TextApplied(Object sender, EventArgs e)
{
lbl1.Text = ucTest.Text;
}
}
另一種方法,如果您不想在 UserControl 中公開 TextBox 的 Text 屬性,只需在 Case WebUserControlTest.FindControl(" txtbox1”)。
下面是在父 Web 表單后面的代碼上注冊事件處理程序的更簡單方法。
父表單 asxp.cs 的代碼如下
protected override void OnInit(EventArgs e)
{
//find the button control within the user control
Button button = (Button)WebUserControlTest.FindControl("btn1");
//wire up event handler
button.Click += new EventHandler(button_Click);
base.OnInit(e);
}
void button_Click(object sender, EventArgs e)
{
TextBox txt = (TextBox) WebUserControlTest.FindControl("txtbox1");
//id of lable which is present in the parent webform
lblParentForm.Text=txt.text;
}
此外,您可以使用如下所示的 JavaScript 來實現這一點(給定上面的代碼):
<script type="text/javascript">
function getUCTextboxValue() {
let txtName = document.getElementById('<%=ucTest.FindControl("txtbox1").ClientID %>');
let lbl = document.getElementById('<%=lbl1.ClientID %>');
lbl.innerText = txtName.value
}
此外,從父頁面,您可以添加一個 HiddenField 並將文本框值保存在其上(使用上面的 JavaScript 代碼),然后從后面的代碼中捕獲其值。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.