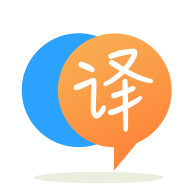
[英]OleDbConnection: How to open and close a connection using a function
[英]Using OleDbConnection how to randomize words
使用OleDbConnection,您將如何連接到Access中包含20個單詞的表,然后使其隨機從表中選擇一個單詞並將其存儲在表單的標簽中?
public partial class MainForm : Form
{
// Use this connection string if your database has the extension .accdb
private const String access7ConnectionString =
@"Provider=Microsoft.ACE.OLEDB.12.0;Data Source=|DataDirectory|\WordsDB.accdb";
// Data components
private OleDbConnection myConnection;
private DataTable myDataTable;
private OleDbDataAdapter myAdapter;
private OleDbCommandBuilder myCommandBuilder;
// Index of the current record
private int currentRecord = 0;
private void MainForm_Load(object sender, EventArgs e)
{
String command = "SELECT * FROM Words";
try
{
myConnection = new OleDbConnection(access7ConnectionString);
myAdapter = new OleDbDataAdapter(access7ConnectionString, myConnection);
myCommandBuilder = new OleDbCommandBuilder(myAdapter);
myDataTable = new DataTable();
FillDataTable(command);
DisplayRow(currentRecord);
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void FillDataTable(String selectCommand)
{
try
{
myConnection.Open();
myAdapter.SelectCommand.CommandText = selectCommand;
// Fill the datatable with the rows reurned by the select command
myAdapter.Fill(myDataTable);
myConnection.Close();
}
catch(Exception ex)
{
MessageBox.Show("Error in FillDataTable : \r\n" + ex.Message);
}
}
private void DisplayRow(int rowIndex)
{
// Check that we can retrieve the given row
if (myDataTable.Rows.Count == 0)
return; // nothing to display
if (rowIndex >= myDataTable.Rows.Count)
return; // the index is out of range
// If we get this far then we can retrieve the data
try
{
DataRow row = myDataTable.Rows[rowIndex];
WordsLbl.Text = row["SpellingWords"].ToString();
}
catch (Exception ex)
{
MessageBox.Show("Error in DisplayRow : \r\n" + ex.Message);
}
}
}
如您所見,這是代碼,我想理解的是,如何從數據庫中的表中獲取隨機單詞並將其存儲在標簽中? 另外,我正在使用OleDbConnection來連接數據庫!
您可以在DataTable
上使用AsEnumerable
,然后可以在其中選擇一個隨機詞。例如,在填充數據表后:
FillDataTable(command);
var words = myDataTable.AsEnumerable()
.Select(d => d["word"].ToString())
.ToList();
Random rnd = new Random();
int randomNumber = rnd.Next(0, words.Count + 1);
string randomWord = words[randomNumber];
label1.Text = randomWord;
這應該起作用。只需在此行中用數據庫中的列名更改word
: .Select(d => d["word"].ToString())
在查看“答案”的“拒絕編輯”后,以下是適用於最初提出的問題的部分:
您可以嘗試使用“ Random”類生成一個隨機數,如下所示:
// =========== Get a Random Number first, then call DisplayRow() ===============
// Figure out the upper range of numbers to choose from the rows returned
int maxCount = myDataTable.Rows.Count;
// generate a random number to use for "rowIndex" in your 'myDataTable.Rows[rowIndex]'
Random randomNR = new Random();
int myRndNR = randomNR.Next(0, maxCount - 1);
// Execute your DisplayRow(int rowIndex) using myRndNR
DisplayRow(myRndNR);
// ===========
至於為什么您的程序無法正常工作(在評論中提出),我建議以下內容:
我將您的代碼復制並粘貼到一個新項目中,並按照您的描述創建了一個Access數據庫...我相信您遇到的問題是:
問題一:您的程序找不到“ WordsDB.accdb”。
可能的解決方案:
(1)您可以弄清楚如何創建正確的| DataDirectory |。 您的“連接字符串”中的路徑或
(2)將“ WordsDB.accdb”放置在您可以使用完整路徑引用的文件夾中,例如“ Data Source = C:\\ inetpub \\ wwwroot \\ app_data \\ WordsDB.accdb ”,因此您的“連接字符串”如下所示:
private const String access7ConnectionString = @"Provider=Microsoft.ACE.OLEDB.12.0;
Data Source=C:\inetpub\wwwroot\app_data\WordsDB.accdb";
問題二:您需要將隨機代碼移出當前位置,以便在填充DataTable之后執行該代碼。
可能的解決方案:
(1)將Form1.cs更改為如下所示:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Data.OleDb;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
// Use this connection string if your database has the extension .accdb
private const String access7ConnectionString =
@"Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\inetpub\wwwroot\app_data\WordsDB.accdb";
// Data components
private OleDbConnection myConnection;
private DataTable myDataTable;
private OleDbDataAdapter myAdapter;
private OleDbCommandBuilder myCommandBuilder;
// Index of the current record
private int currentRecord = 0;
private void MainForm_Load(object sender, EventArgs e)
{
String command = "SELECT * FROM Words";
try
{
myConnection = new OleDbConnection(access7ConnectionString);
myAdapter = new OleDbDataAdapter(access7ConnectionString, myConnection);
myCommandBuilder = new OleDbCommandBuilder(myAdapter);
myDataTable = new DataTable();
FillDataTable(command);
/* Move this "DisplayRow(currentRecord);" down below and use
after you get a random number to pick a random word as shown
below... */
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
// =========== Get a Random Number first, then call DisplayRow() ===============
// Figure out the upper range of numbers to choose from the rows returned
int maxCount = myDataTable.Rows.Count;
// generate a random number to use for "rowIndex" in your 'myDataTable.Rows[rowIndex]'
Random randomNR = new Random();
int myRndNR = randomNR.Next(0, maxCount - 1);
// Execute your DisplayRow(int rowIndex) using myRndNR
DisplayRow(myRndNR);
// ===========
}
private void FillDataTable(String selectCommand)
{
try
{
myConnection.Open();
myAdapter.SelectCommand.CommandText = selectCommand;
// Fill the DataTable with the rows returned by the select command
myAdapter.Fill(myDataTable);
myConnection.Close();
}
catch (Exception ex)
{
MessageBox.Show("Error in FillDataTable : \r\n" + ex.Message);
}
}
private void DisplayRow(int rowIndex)
{
// Check that we can retrieve the given row
if (myDataTable.Rows.Count == 0)
return; // nothing to display
if (rowIndex >= myDataTable.Rows.Count)
return; // the index is out of range
// If we get this far then we can retrieve the data
try
{
DataRow row = myDataTable.Rows[rowIndex];
WordsLbl.Text = row["SpellingWords"].ToString();
}
catch (Exception ex)
{
MessageBox.Show("Error in DisplayRow : \r\n" + ex.Message);
}
}
public Form1()
{
InitializeComponent();
}
}
}
潛在問題:
您可能沒有將“事件,行為,加載”設置為要執行的表單
MainForm_Load
潛在的解決方案:
一種。 轉到Visual Studio中的“設計”視圖,然后右鍵單擊表單。
b。 點擊彈出窗口中的“屬性”
C。 在“屬性”窗口(可能位於Visual Studio的右下角)中查找“事件”
d。 找到“事件”后,請查找“行為”(如果按“類別”對屬性進行排序)或“加載”(如果按字母順序對屬性進行排序)
e。 在“加載”框中,確保其顯示“ MainForm_Load”
由於您正在使用訪問權限,因此,一旦您的數據表中填充了行,便可以執行此操作。 填充數據表后,我已經能夠對此進行測試。 我從另一個數據庫填充了數據表,但這沒關系。 我的代碼的最后4行可幫助您每次從數據表中獲得隨機記錄。
{
const string query = "SELECT name FROM NewTable";
var command = new SqlCommand(){CommandText = query, CommandType = System.Data.CommandType.Text,Connection=sqlConn};
var dataAdapter = new SqlDataAdapter() { SelectCommand = command };
DataTable dataTable = new DataTable("Names");
dataAdapter.Fill(dataTable);
int count = dataTable.Rows.Count;
int index = new Random().Next(count);
DataRow d = dataTable.Rows[index];
Console.WriteLine(d[0]);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.