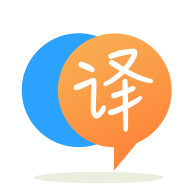
[英]WPF Select multiple items in DataGrid from ViewModel with twoway binding
[英]Select multiple items from a DataGrid in an MVVM WPF project
如何從 MVVM WPF 項目的DataGrid
中選擇多個項目?
你可以簡單地添加一個自定義依賴屬性來做到這一點:
public class CustomDataGrid : DataGrid
{
public CustomDataGrid ()
{
this.SelectionChanged += CustomDataGrid_SelectionChanged;
}
void CustomDataGrid_SelectionChanged (object sender, SelectionChangedEventArgs e)
{
this.SelectedItemsList = this.SelectedItems;
}
#region SelectedItemsList
public IList SelectedItemsList
{
get { return (IList)GetValue (SelectedItemsListProperty); }
set { SetValue (SelectedItemsListProperty, value); }
}
public static readonly DependencyProperty SelectedItemsListProperty =
DependencyProperty.Register ("SelectedItemsList", typeof (IList), typeof (CustomDataGrid), new PropertyMetadata (null));
#endregion
}
現在您可以在 XAML 中使用這個dataGrid
:
<Window x:Class="DataGridTesting.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:i="http://schemas.microsoft.com/expression/2010/interactivity"
xmlns:local="clr-namespace:DataGridTesting.CustomDatagrid"
Title="MainWindow"
Height="350"
Width="525">
<DockPanel>
<local:CustomDataGrid ItemsSource="{Binding Model}"
SelectionMode="Extended"
AlternatingRowBackground="Aquamarine"
SelectionUnit="FullRow"
IsReadOnly="True"
SnapsToDevicePixels="True"
SelectedItemsList="{Binding TestSelected, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}"/>
</DockPanel>
</Window>
我的ViewModel
:
public class MyViewModel : INotifyPropertyChanged
{
private static object _lock = new object ();
private List<MyModel> _myModel;
public IEnumerable<MyModel> Model { get { return _myModel; } }
private IList _selectedModels = new ArrayList ();
public IList TestSelected
{
get { return _selectedModels; }
set
{
_selectedModels = value;
RaisePropertyChanged ("TestSelected");
}
}
public MyViewModel ()
{
_myModel = new List<MyModel> ();
BindingOperations.EnableCollectionSynchronization (_myModel, _lock);
for (int i = 0; i < 10; i++)
{
_myModel.Add (new MyModel
{
Name = "Test " + i,
Age = i * 22
});
}
RaisePropertyChanged ("Model");
}
public event PropertyChangedEventHandler PropertyChanged;
public void RaisePropertyChanged (string propertyName)
{
var pc = PropertyChanged;
if (pc != null)
pc (this, new PropertyChangedEventArgs (propertyName));
}
}
我的型號:
public class MyModel
{
public string Name { get; set; }
public int Age { get; set; }
}
最后,這是MainWindow
背后的代碼:
public partial class MainWindow : Window
{
public MainWindow ()
{
InitializeComponent ();
this.DataContext = new MyViewModel ();
}
}
我希望這個干凈的 MVVM 設計有所幫助。
我會做的是使用System.Windows.Interactivity
創建Behaviors
。 您必須在項目中手動引用它。
給定一個不公開SelectedItems
控件,例如 (ListBox, DataGrid)
你可以創建一個這樣的行為類
public class ListBoxSelectedItemsBehavior : Behavior<ListBox>
{
protected override void OnAttached()
{
AssociatedObject.SelectionChanged += AssociatedObjectSelectionChanged;
}
protected override void OnDetaching()
{
AssociatedObject.SelectionChanged -= AssociatedObjectSelectionChanged;
}
void AssociatedObjectSelectionChanged(object sender, SelectionChangedEventArgs e)
{
var array = new object[AssociatedObject.SelectedItems.Count];
AssociatedObject.SelectedItems.CopyTo(array, 0);
SelectedItems = array;
}
public static readonly DependencyProperty SelectedItemsProperty =
DependencyProperty.Register("SelectedItems", typeof(IEnumerable), typeof(ListBoxSelectedItemsBehavior),
new FrameworkPropertyMetadata(null, FrameworkPropertyMetadataOptions.BindsTwoWayByDefault));
public IEnumerable SelectedItems
{
get { return (IEnumerable)GetValue(SelectedItemsProperty); }
set { SetValue(SelectedItemsProperty, value); }
}
}
在您的XAML
我會像這樣進行Binding
,其中i
是xmlns:i="http://schemas.microsoft.com/expression/2010/interactivity"
並且behaviors
是您的Behavior
類的命名空間
<ListBox>
<i:Interaction.Behaviors>
<behaviors:ListBoxSelectedItemsBehavior SelectedItems="{Binding SelectedItems, Mode=OneWayToSource}" />
</i:Interaction.Behaviors>
假設ListBox
的DataContext
在ViewModel
具有SelectedItems
屬性,那么它將自動更新SelectedItems
。 您已經封裝了從View
訂閱的event
,即,
<ListBox SelectionChanged="ListBox_SelectionChanged"/>
如果需要,您可以將Behavior
類更改為DataGrid
類型。
我在我的應用程序中使用此解決方案:
XAML:
<i:Interaction.Triggers>
<i:EventTrigger EventName="SelectionChanged">
<i:InvokeCommandAction Command="{Binding SelectItemsCommand}" CommandParameter="{Binding Path=SelectedItems,ElementName=TestListView}"/>
</i:EventTrigger>
</i:Interaction.Triggers>
在您的 xaml 文件的頂部,添加以下代碼行:
xmlns:i="http://schemas.microsoft.com/expression/2010/interactivity"
SelectedItemsCommand 是 ICommand 類型,它寫在您的視圖模型中。
使用的DLL:
系統.Windows.Interactivity.dll
對於 WPF 的默認DataGrid
,無法使用綁定,因為SelectedItem
-Property 是可能的,因為SelectedItems
-Property 不是 DependencyProperty。
實現您想要的一種方法是注冊 DataGrid 的SelectionChanged
-Event 以更新存儲所選項目的 ViewModel 的屬性。
DataGrid 的SelectedItems屬性屬於 IList 類型,因此您需要將列表中的項目轉換為您的特定類型。
C#
public MyViewModel {
get{
return this.DataContext as MyViewModel;
}
}
private void DataGrid_SelectionChanged(object sender, SelectionChangedEventArgs e) {
// ... Get SelectedItems from DataGrid.
var grid = sender as DataGrid;
var selected = grid.SelectedItems;
List<MyObject> selectedObjects = selected.OfType<MyObject>().ToList();
MyViewModel.SelectedMyObjects = selectedObjects;
}
XAML
<Window x:Class="WpfApplication1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid>
<DataGrid
SelectionChanged="DataGrid_SelectionChanged"
/>
</Grid>
</Window>
您可以在模型中添加“IsSelected”屬性並在行中添加一個復選框。
您可以創建一個可重用的通用基類。 通過這種方式,您可以從代碼和 UI 中選擇行。
這是我想要選擇的示例類
public class MyClass
{
public string MyString {get; set;}
}
為可選類制作通用基類。 當您設置 IsSelected 時,INotifyPropertyChanged 會更新 UI。
public class SelectableItem<T> : System.ComponentModel.INotifyPropertyChanged
{
public SelectableItem(T item)
{
Item = item;
}
public T Item { get; set; }
bool _isSelected;
public bool IsSelected {
get {
return _isSelected;
}
set {
if (value == _isSelected)
{
return;
}
_isSelected = value;
if (PropertyChanged != null)
{
PropertyChanged(this, new System.ComponentModel.PropertyChangedEventArgs("IsSelected"));
}
}
}
public event System.ComponentModel.PropertyChangedEventHandler PropertyChanged;
}
創建可選擇的類
public class MySelectableItem: SelectableItem<MyClass>
{
public MySelectableItem(MyClass item)
:base(item)
{
}
}
創建要綁定到的屬性
ObservableCollection<MySelectableItem> MyObservableCollection ...
設置屬性
MyObservableCollection = myItems.Select(x => new MySelectableItem(x));
綁定到數據網格並在綁定到 MySelectedItem 上的 IsSelected 屬性的 DataGridRow 上添加樣式
<DataGrid
ItemsSource="{Binding MyObservableCollection}"
SelectionMode="Extended">
<DataGrid.Resources>
<Style TargetType="DataGridRow">
<Setter Property="IsSelected" Value="{Binding IsSelected}" />
</Style>
</DataGrid.Resources>
</DataGrid>
獲取選定的行/項目
var selectedItems = MyObservableCollection.Where(x=>x.IsSelected).Select(y=>y.Item);
選擇行/項目
MyObservableCollection[0].IsSelected = true;
編輯——> EnableRowVirtualization為true時好像不行
WPF DataGrid 允許這樣做。 只需將 DataGrid.Rows.SelectionMode 和 DataGrid.Rows.SelectionUnit 分別設置為“Extended”和“CellOrRowHeader”。 這可以在 Blend 中完成,如我包含的圖像所示。 這將允許用戶根據需要選擇每個單元格、整行等,使用 shift 或 ctrl 鍵繼續選擇。
我正在處理的項目使用MVVM Light ,我發現這篇博文是最簡單的解決方案。 我將在這里重復解決方案:
查看型號:
using GalaSoft.MvvmLight;
using GalaSoft.MvvmLight.Command;
...
public class SomeVm : ViewModelBase {
public SomeVm() {
SelectItemsCommand = new RelayCommand<IList>((items) => {
Selected.Clear();
foreach (var item in items) Selected.Add((SomeClass)item);
});
ViewCommand = new RelayCommand(() => {
foreach (var selected in Selected) {
// todo do something with selected items
}
});
}
public List<SomeClass> Selected { get; set; }
public RelayCommand<IList> SelectionChangedCommand { get; set; }
public RelayCommand ViewCommand { get; set; }
}
XAML:
<Window
...
xmlns:i="http://schemas.microsoft.com/expression/2010/interactivity"
xmlns:command="http://www.galasoft.ch/mvvmlight"
...
<DataGrid
Name="SomeGrid"
...
<i:Interaction.Triggers>
<i:EventTrigger EventName="SelectionChanged">
<command:EventToCommand
Command="{Binding SelectionChangedCommand}"
CommandParameter="{Binding SelectedItems, ElementName=SomeGrid}" />
</i:EventTrigger>
</i:Interaction.Triggers>
...
<DataGrid.ContextMenu>
<ContextMenu>
<MenuItem Header="View" Command="{Binding ViewCommand}" />
</ContextMenu>
</DataGrid.ContextMenu>
...
我的解決方案幾乎與 Sandesh 相同。 另一方面,我沒有使用 CustomDataGrid 來解決這個問題。 相反,我在視圖模型中使用了具有適當功能的加號按鈕單擊事件。 在我的代碼中,要點是能夠從綁定到 PeopleList 屬性(ObservableCollection)的 Datagrid 中刪除多個人物對象
這是我的模型:
public class Person
{
public Person()
{
}
public string FirstName { get; set; }
public string LastName { get; set; }
public int Age { get; set; }
}
}
這是我的 ViewModel(只有那些必要的部分) :
public class PersonViewModel : BindableBase
{
private ObservableCollection<Person> _peopleList;
// to be able to delete or save more than one person object
private List<Person> _selectedPersonList;
//MyICommand
public MyICommand AddCommand { get; set; }
public MyICommand DeleteCommand { get; set; }
private string _firstName;
private string _lastName;
private int _age;
public PersonViewModel()
{
_peopleList = new ObservableCollection<Person>();
LoadPerson();
AddCommand = new MyICommand(AddPerson);
DeleteCommand = new MyICommand(DeletePerson, CanDeletePerson);
// To be able to delete or save more than one person
_selectedPersonList = new List<Person>();
}
public ObservableCollection<Person> PeopleList
{
get { return _peopleList; }
set
{
_peopleList = value;
RaisePropertyChanged("PeopleList");
}
}
public List<Person> SelectedPersonList
{
get { return _selectedPersonList; }
set
{
if (_selectedPersonList != value)
{
RaisePropertyChanged("SelectedPersonList");
}
}
}
private void DeletePerson()
{
// to be able to delete more than one person object
foreach (Person person in SelectedPersonList)
{
PeopleList.Remove(person);
}
MessageBox.Show(""+SelectedPersonList.Count); // it is only a checking
SelectedPersonList.Clear(); // it is a temp list, so it has to be cleared after the button push
}
public void GetSelectedPerson(DataGrid datagrid)
{
IList selectedItems = datagrid.SelectedItems;
foreach (Person item in selectedItems)
{
SelectedPersonList.Add(item);
}
}
我的觀點(xmal):
<UserControl x:Class="DataBase.Views.PersonView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:DataBase.Views"
xmlns:viewModel="clr-namespace:DataBase.ViewModels" d:DataContext="{d:DesignInstance Type=viewModel:PersonViewModel}"
mc:Ignorable="d"
d:DesignHeight="450" d:DesignWidth="800">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="*"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*"/>
<ColumnDefinition Width="*"/>
</Grid.ColumnDefinitions>
<StackPanel Orientation="Vertical" Grid.Row="0" Grid.Column="0" Grid.RowSpan="2" Grid.ColumnSpan="2" Background="AliceBlue">
<TextBlock Text="First Name:"/>
<TextBox x:Name="firstNameTxtBox" Text="{Binding FirstName, UpdateSourceTrigger=PropertyChanged}"/>
<TextBlock Text="Last Name:"/>
<TextBox x:Name="lastNameTxtBox" Text="{Binding LastName, UpdateSourceTrigger=PropertyChanged}"/>
<TextBlock Text="Age:"/>
<TextBox x:Name="ageTxtBox" Text="{Binding Age}"/>
<TextBlock Text="{Binding FullName}"/>
<Button Content="Add" IsEnabled="{Binding CanAddPerson}" Command="{Binding AddCommand}"/>
<Button Content="Delete" Command="{Binding DeleteCommand}" Click="Delete_Click"/>
<DataGrid x:Name="datagridPeopleList" ItemsSource="{Binding PeopleList}" AutoGenerateColumns="True" SelectedItem="{Binding SelectedPerson}" SelectionMode="Extended" SelectionUnit="FullRow"/>
<!--<ListView Height="50" ItemsSource="{Binding PeopleList}" SelectedItem="{Binding SelectedPerson}" Margin="10">
</ListView>-->
</StackPanel>
</Grid>
</UserControl>
我的觀點(.cs):
public partial class PersonView : UserControl
{
public PersonViewModel pvm;
public PersonView()
{
pvm = new PersonViewModel();
InitializeComponent();
DataContext = pvm;
}
private void Delete_Click(object sender, RoutedEventArgs e)
{
pvm.GetSelectedPerson(datagridPeopleList);
}
}
我希望它是有用的,而不是世界上最糟糕(不優雅)的解決方案:D
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.